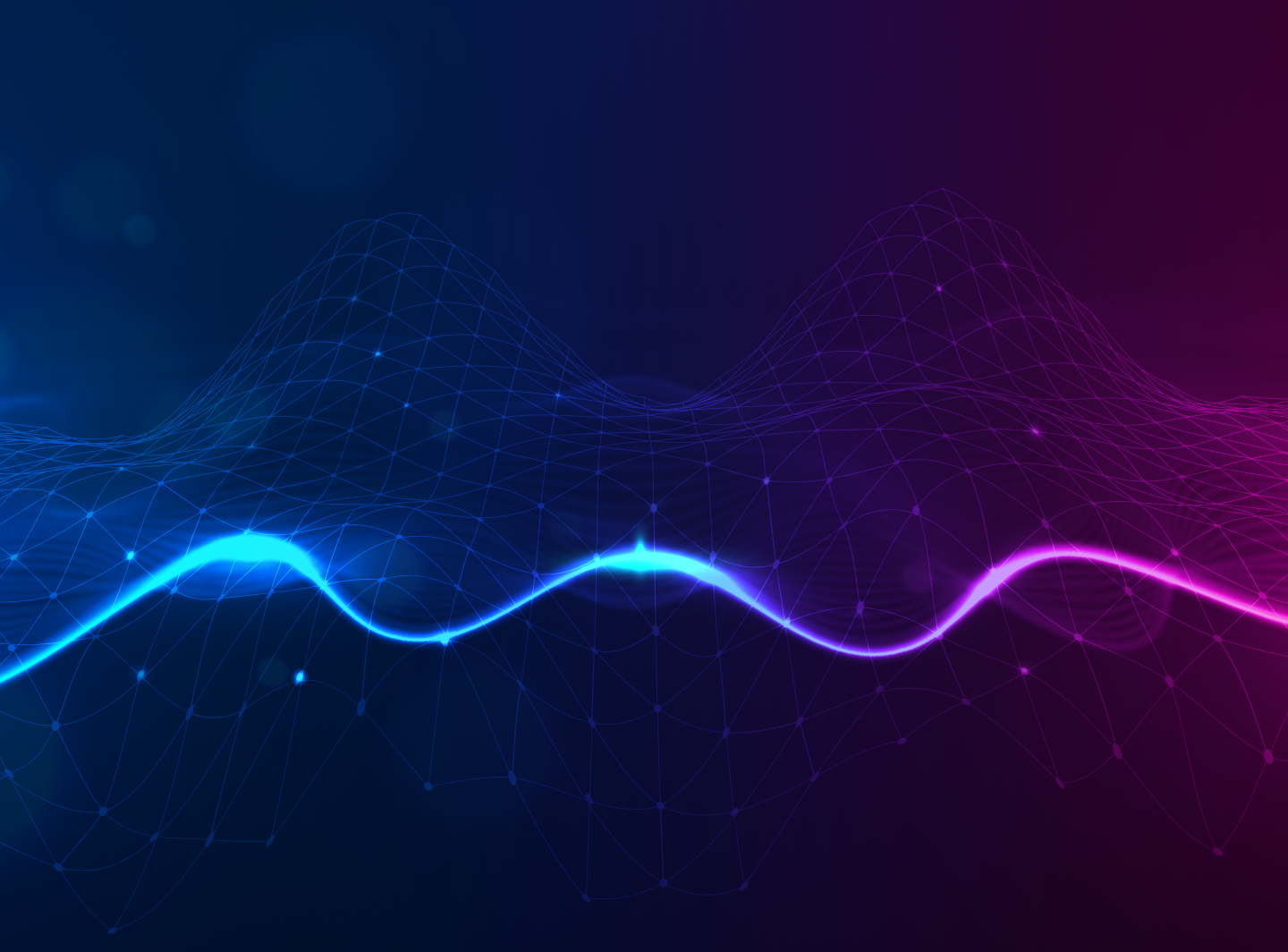
Share what you learn in this blog to prepare for your interview, create your forever-free profile now, and explore how to monetize your valuable knowledge.
In this tutorial, we'll explore how to create a RESTful API using Go, a powerful programming language known for its performance and simplicity. We'll use the standard library along with the popular Gorilla Mux router to handle HTTP requests and responses.
package main
import (
"encoding/json"
"log"
"net/http"
"github.com/gorilla/mux"
)
type Book struct {
ID string `json:"id"`
Title string `json:"title"`
Author string `json:"author"`
Price float64 `json:"price"`
}
var books []Book
func main() {
router := mux.NewRouter()
router.HandleFunc("/books", getBooks).Methods("GET")
router.HandleFunc("/books/{id}", getBook).Methods("GET")
router.HandleFunc("/books", createBook).Methods("POST")
router.HandleFunc("/books/{id}", updateBook).Methods("PUT")
router.HandleFunc("/books/{id}", deleteBook).Methods("DELETE")
log.Fatal(http.ListenAndServe(":8000", router))
}
func getBooks(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
json.NewEncoder(w).Encode(books)
}
func getBook(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
params := mux.Vars(r)
for _, item := range books {
if item.ID == params["id"] {
json.NewEncoder(w).Encode(item)
return
}
}
json.NewEncoder(w).Encode(&Book{})
}
func createBook(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
var book Book
_ = json.NewDecoder(r.Body).Decode(&book)
books = append(books, book)
json.NewEncoder(w).Encode(book)
}
func updateBook(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
params := mux.Vars(r)
for index, item := range books {
if item.ID == params["id"] {
books[index] = Book{
ID: params["id"],
Title: item.Title,
Author: item.Author,
Price: item.Price,
}
json.NewEncoder(w).Encode(books[index])
return
}
}
json.NewEncoder(w).Encode(&Book{})
}
func deleteBook(w http.ResponseWriter, r *http.Request) {
w.Header().Set("Content-Type", "application/json")
params := mux.Vars(r)
for index, item := range books {
if item.ID == params["id"] {
books = append(books[:index], books[index+1:]...)
break
}
}
json.NewEncoder(w).Encode(books)
}
This is a basic example of how to build a RESTful API with Go. We define routes for CRUD operations on a collection of books. The Gorilla Mux router helps us handle different HTTP methods and route parameters easily.
397 views
Please Login to create a Question