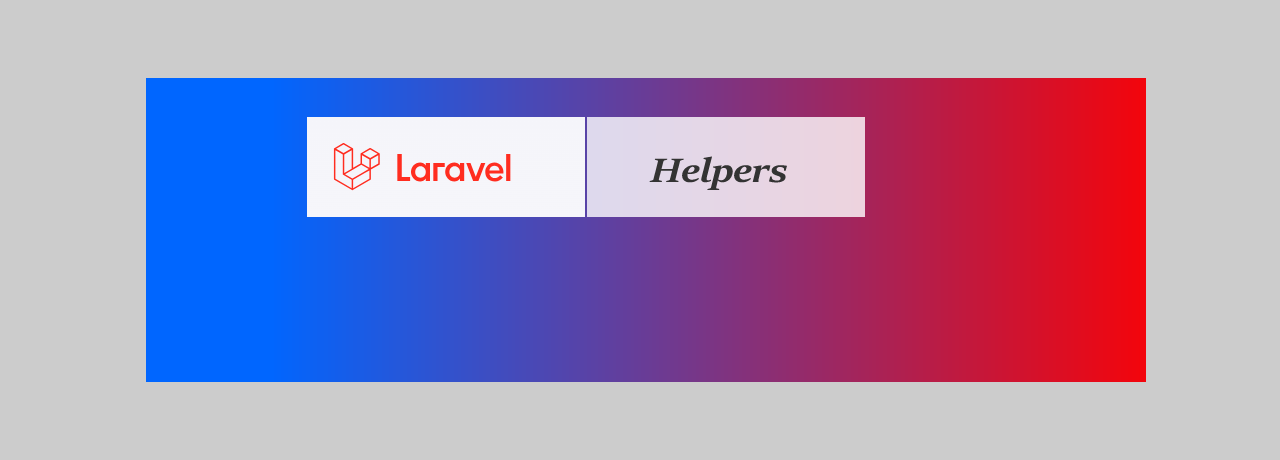
Laravel provides a powerful Number class in its helpers that allows you to format and manipulate numbers easily. In this tutorial, we will explore various methods provided by the Number class, such as abbreviating large numbers, formatting decimals, displaying percentages, working with currencies, converting file sizes, and creating human-readable representations of numbers.
Number::abbreviate
The abbreviate method is used to abbreviate large numbers, making them more readable. For instance, it can convert 1000 to 1k and 1000000 to 1M. Here's an example:
To use Nubmer Class add
use Illuminate\Support\Number;
$abbreviatedNumber = Number::abbreviate(1500);
// Output: 1.5k
// Abbreviate 12345
$abbreviated = Number::abbreviate(12345); // Output: 12.3K
// Specify precision and symbol
$abbreviated = Number::abbreviate(1234567, 2, 'Mn'); // Output: 1.23Mn
// Custom symbol mapping
Number::macro('myAbbr', function ($number, $precision = 2) {
return substr($number, 0, $precision) . '...';
});
$customAbbr = Number::myAbbr(1234567); // Output: 123...
Number::format
The format method, formats numbers based on the current locale, including separators, decimal points, etc.
// Format with default locale
$formatted = Number::format(1234.567); // Output: 1,234.57 (depending on locale)
// Specify locale
$formatted = Number::format(1234.567, 'de'); // Output: 1.234,57 (German format)
// Custom formatting options
$formatted = Number::format(1234.567, 2, ',', '.'); // Output: 1,234.57
Number::percentage
The percentage method converts a number into a percentage format
// Convert 0.5 to percentage
$percentage = Number::percentage(0.5); // Output: 50%
$percentage = Number::percentage(0.75);
// Output: 75%
// Specify precision and symbol
$percentage = Number::percentage(0.523, 2, '%'); // Output: 52.30%
Number::currency
The currency method is used to format numbers as currency:
$currencyAmount = Number::currency(12345.67, 'USD');
// Output: $12,345.67
Number::fileSize
The fileSize method converts a file size in bytes to a human-readable format:
// Convert 1024 bytes to file size
$fileSize = Number::fileSize(1024); // Output: 1 KB
// Specify precision and symbol
$fileSize = Number::fileSize(123456789, 2, 'TiB'); // Output: 117.72 TiB
Number::forHumans
The forHumans method provides a human-readable representation of a number, automatically choosing the appropriate format:
// Convert 60 seconds to human-readable format
$humanTime = Number::forHumans(60); // Output: 1 minute
// Specify units and rounding
$humanTime = Number::forHumans(12345, 'minutes', true); // Output: about 20 minutes
Summary:
In this tutorial, we've explored various methods provided by the Laravel Helper Number class. These methods allow you to manipulate and format numbers in a variety of ways, making it easy to work with different types of numerical data in your Laravel applications.
By incorporating these helper methods into your code, you can enhance the user experience by presenting numbers in a more readable and user-friendly manner. Feel free to experiment with these methods and adjust the parameters to suit your specific requirements.
602 views