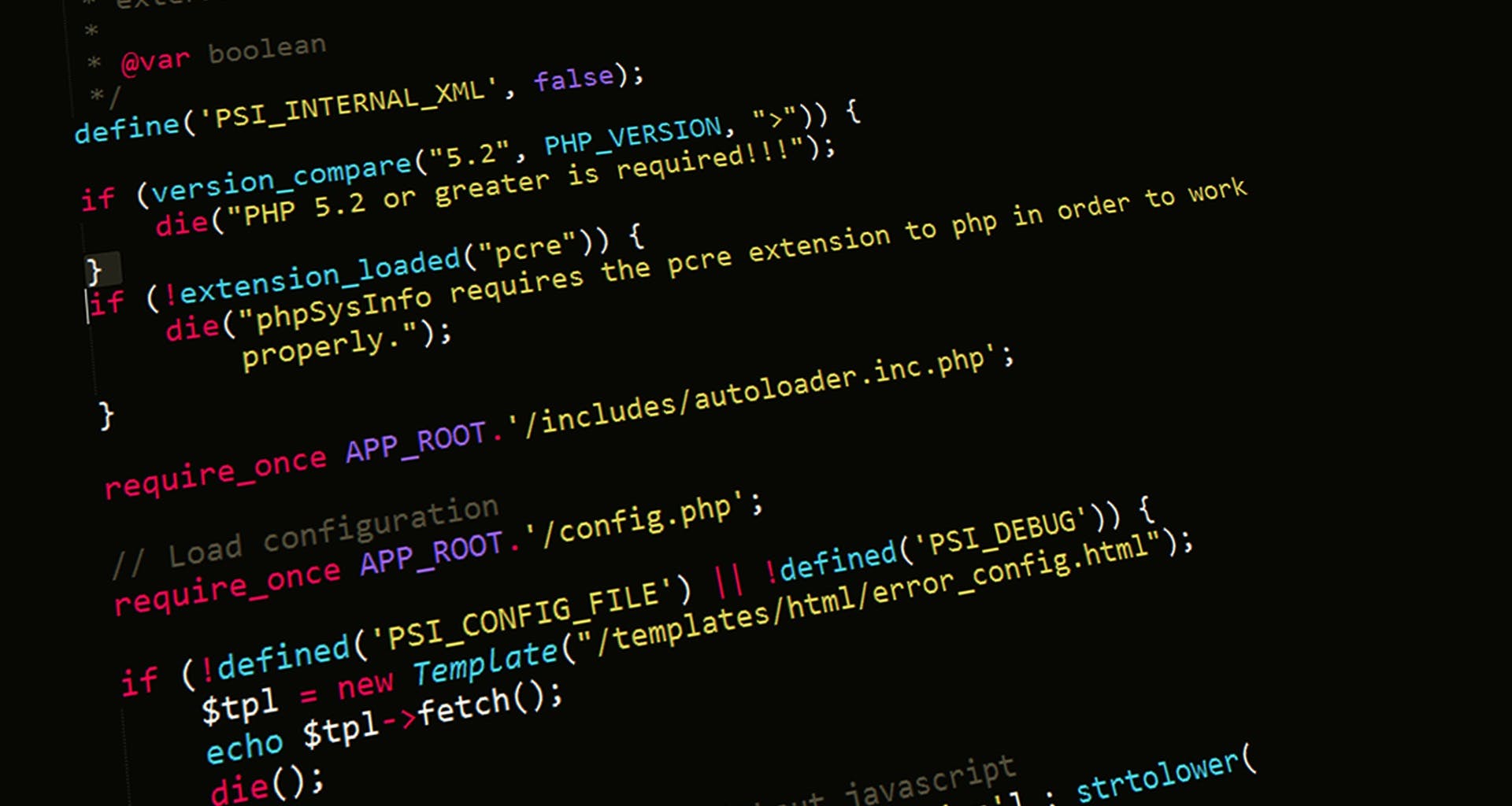
February 09, 2024
Creating a CRUD Application with Flask and SQLAlchemy
Share what you learn in this blog to prepare for your interview, create your forever-free profile now, and explore how to monetize your valuable knowledge.
Flask is a lightweight web framework for Python, while SQLAlchemy is a powerful SQL toolkit and Object-Relational Mapper (ORM). In this tutorial, we'll build a simple CRUD (Create, Read, Update, Delete) application using Flask and SQLAlchemy to interact with a SQLite database.
First, let's install the necessary libraries:
pip install Flask Flask-SQLAlchemy
Now, let's create our Flask application and define our models in app.py:
from flask import Flask, request, jsonify
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///crud.db'
db = SQLAlchemy(app)
class Item(db.Model):
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(100), nullable=False)
description = db.Column(db.Text)
@app.route('/items', methods=['GET'])
def get_items():
items = Item.query.all()
output = []
for item in items:
data = {
'id': item.id,
'name': item.name,
'description': item.description
}
output.append(data)
return jsonify({'items': output})
@app.route('/items', methods=['POST'])
def create_item():
data = request.get_json()
new_item = Item(name=data['name'], description=data['description'])
db.session.add(new_item)
db.session.commit()
return jsonify({'message': 'Item created successfully'}), 201
@app.route('/items/<int:item_id>', methods=['PUT'])
def update_item(item_id):
item = Item.query.get_or_404(item_id)
data = request.get_json()
item.name = data['name']
item.description = data['description']
db.session.commit()
return jsonify({'message': 'Item updated successfully'})
@app.route('/items/<int:item_id>', methods=['DELETE'])
def delete_item(item_id):
item = Item.query.get_or_404(item_id)
db.session.delete(item)
db.session.commit()
return jsonify({'message': 'Item deleted successfully'})
if __name__ == '__main__':
db.create_all()
app.run(debug=True)
With this setup, we have created a simple Flask application with endpoints for creating, reading, updating, and deleting items in the database.
To test our application, we can use tools like Postman or curl to make requests to our server.
This is just a basic example, but you can extend it by adding more endpoints, implementing authentication, validation, and other features to suit your needs.
381 views
Please Login to create a Question