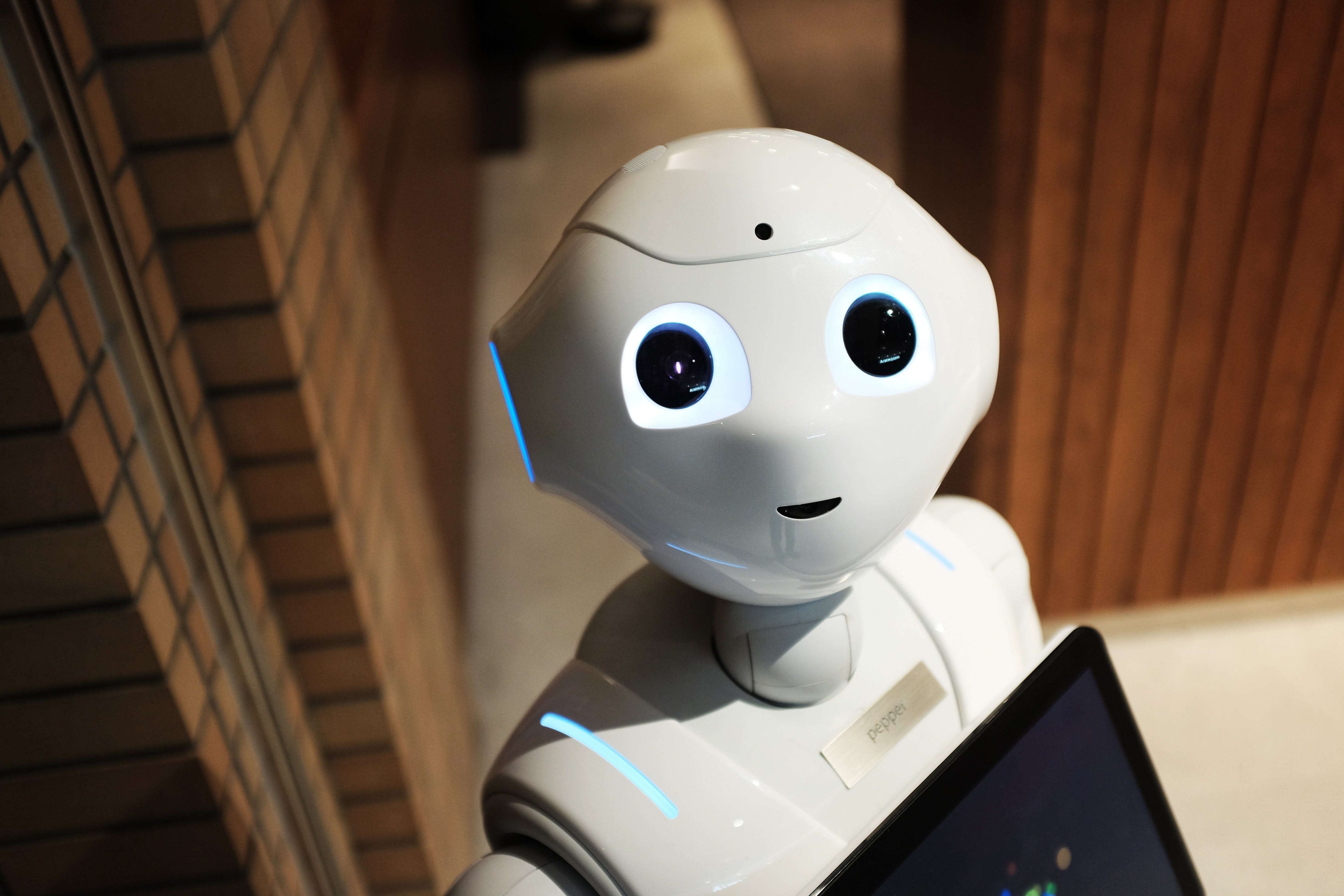
February 09, 2024
Introduction to Machine Learning with Python and scikit-learn
Machine learning is a powerful field that enables computers to learn from data and make predictions or decisions without being explicitly programmed. In this tutorial, we'll introduce the basics of machine learning using Python and scikit-learn, a popular machine learning library.
First, let's install the necessary libraries:
pip install scikit-learn numpy pandas matplotlib
Now, let's create a simple machine learning model to predict the species of iris flowers based on their sepal and petal measurements:
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load the iris dataset
iris = load_iris()
X, y = iris.data, iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create and train the K-Nearest Neighbors classifier
knn = KNeighborsClassifier(n_neighbors=3)
knn.fit(X_train, y_train)
# Make predictions on the test set
y_pred = knn.predict(X_test)
# Calculate the accuracy of the model
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
In this script, we load the iris dataset, split it into training and testing sets, create a K-Nearest Neighbors classifier, train it on the training data, make predictions on the test data, and calculate the accuracy of the model.
To run the script, simply execute it:
python script.py
This is just a simple example of machine learning with scikit-learn, but there are many other algorithms and techniques you can explore to solve different types of problems. Experiment with different datasets, algorithms, and parameters to gain a deeper understanding of machine learning concepts and techniques.
272 views