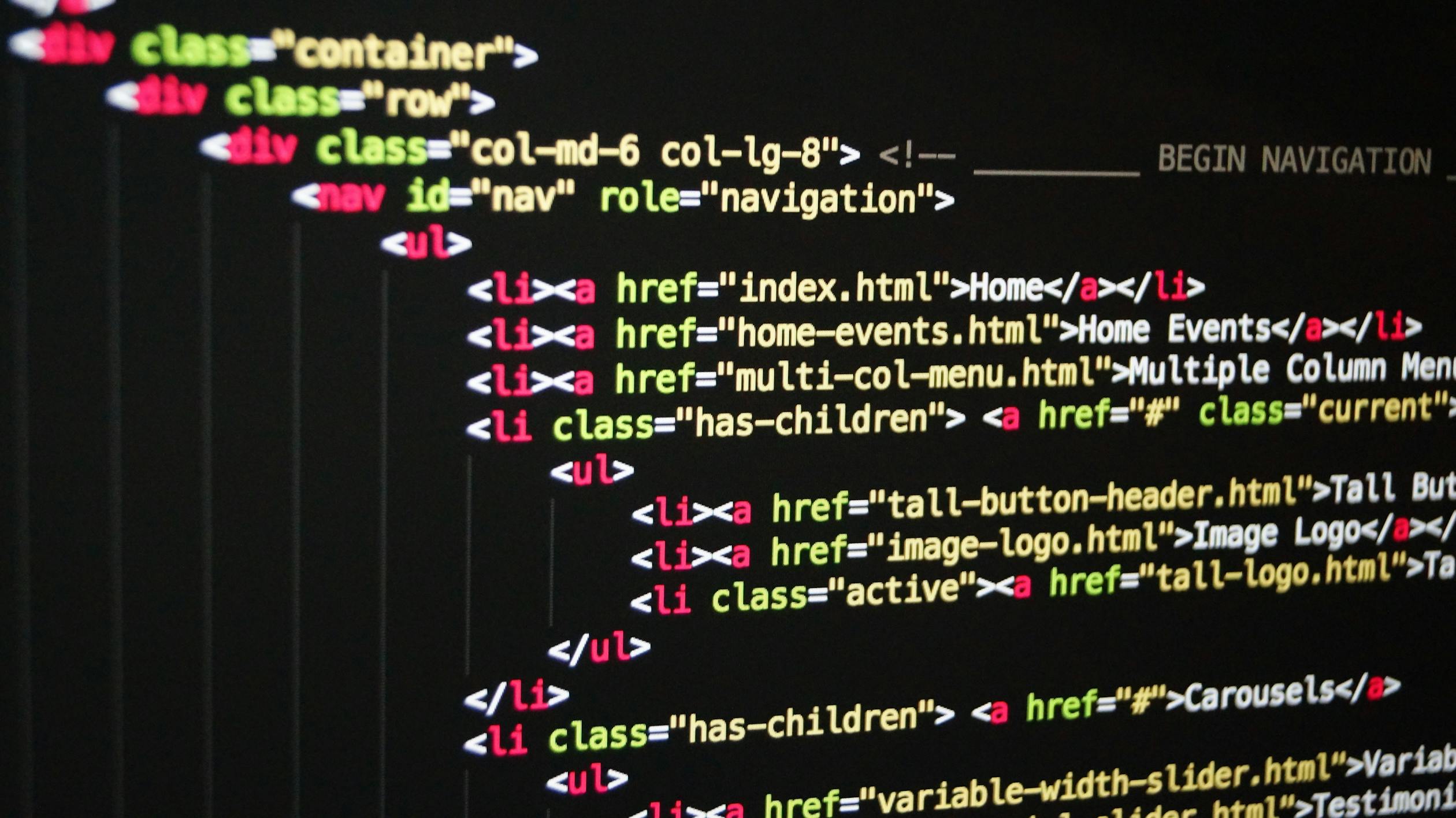
November 08, 2023
Getting Started with TypeScript: A Beginner's Guide
IntroductionTypeScript is a powerful, statically-typed superset of JavaScript that can help you write cleaner, safer, and more maintainable code. If you're new to TypeScript and want to learn the basics, you've come to the right place. In this beginner's guide, we'll take you through the fundamental concepts of TypeScript and get you started on your journey to becoming a TypeScript developer.
What is TypeScript?
TypeScript is a statically-typed programming language developed by Microsoft. It builds upon JavaScript by adding static types, which allow you to catch and prevent errors during development and improve code quality. TypeScript code is transpiled into plain JavaScript, making it compatible with all major web browsers and runtime environments.
Setting Up Your Development Environment
Before you can start writing TypeScript code, you need to set up your development environment. Follow these steps to get started:
- Node.js Installation: TypeScript uses Node.js for its development environment. Download and install Node.js from the official website (https://nodejs.org/), as it includes npm (Node Package Manager).
- Installing TypeScript: Open your command line or terminal and install TypeScript globally using npm with the following command:
npm install -g typescript
Text Editor or IDE: Choose a code editor or integrated development environment (IDE) to write your TypeScript code. Popular choices include Visual Studio Code, Sublime Text, and WebStorm.
Writing Your First TypeScript Code
Now that you have TypeScript installed and your development environment set up, let's write your first TypeScript code. Create a new file with a ".ts" extension, which is the file extension for TypeScript source files. Here's a simple example:
// hello.ts
function sayHello(name: string) {
console.log(`Hello, ${name}!`);
}
sayHello("TypeScript");
In this example, we define a function sayHello that takes a name parameter of type string and logs a greeting message. The last line calls the function with the argument "TypeScript". TypeScript's type annotations make it clear that name must be a string, preventing you from passing incompatible data types.
Compiling TypeScript
To convert your TypeScript code into JavaScript, you need to compile it. Open your terminal or command line and navigate to the directory containing your TypeScript file, then run the following command:
tsc hello.ts
This will generate a JavaScript file, hello.js, in the same directory. You can now run the JavaScript code using Node.js:
node hello.js
TypeScript's Compilation Process
TypeScript's compilation process performs type checking and produces JavaScript code. During compilation, TypeScript checks your code for type errors and compiles it to JavaScript. It also provides features like modern ECMAScript support, module loading, and more.
Conclusion
This beginner's guide is just the first step on your TypeScript journey. TypeScript offers many advanced features and concepts that can help you write cleaner and more maintainable code. In future blog posts, we'll explore TypeScript's type system, advanced features, and how to integrate it with various front-end and back-end frameworks.
Stay tuned for more TypeScript tutorials and start experimenting with TypeScript to see how it can enhance your web development projects. Happy coding!
356 views