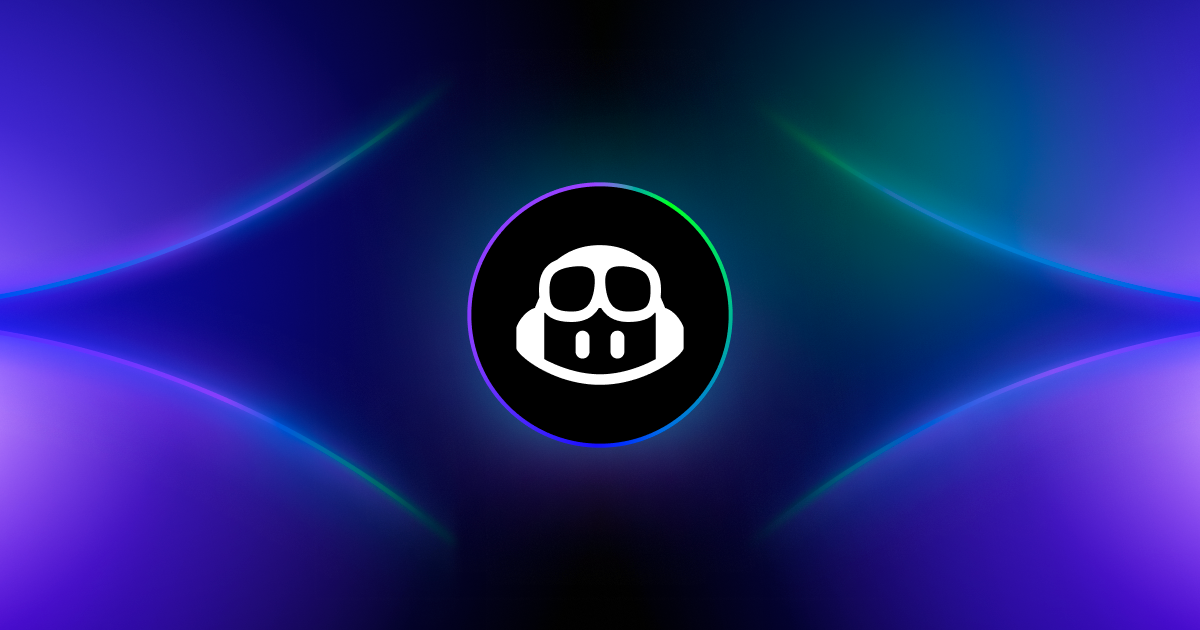
May 16, 2025
How to Debug Code with GitHub Copilot
How to Debug Code with GitHub Copilot
Did you ever spend hours looking at your system trying to figure out why your code didn't work and then your find a minor error or missing semicolon? So exhausted, right? Debugging is tedious, time-consuming, and occasionally soul-crushing.
What if you had an AI-powered coding assistant that could spot problems, offer fixes, and explain the problem? GitHub Copilot helps here.
Most people know Copilot for autocompleting code, but it is also a great debugger. Copilot speeds up syntax mistakes, logic issue fixes, and pull request reviews without stressing you out. This blogpost will teach you how to easily debug code using GitHub Copilot and why it may become your new best friend.
Getting Started with GitHub Copilot for Debugging
Ensure you have GitHub Copilot before debugging. Simply install the GitHub Copilot extension in VS Code and login in with your GitHub account. Once running, it will provide real-time recommendations while you code.
Copilot can debug as well as write code. Real magic occurs with Copilot Chat, which lets you express an issue in simple English and get AI-powered suggestions. Use /fix to suggest changes or /explain to see your code's errors.
After setting up everything, let's debug!
Debugging in Your IDE with Copilot
I have written functions that returned non-logical error messages many times. Copilot's real-time code recommendations and fixes in your IDE simplify troubleshooting.
Imagine forgetting a Python function argument:
def add_numbers(a, b):
return a + b
print(add_numbers(5)) # Oops! Missing argument
Copilot understands the issue and recommends adding the missing parameter. So, stop checking out Stack Overflow for solutions from now on!
Not only for Python. If you neglect to end a JavaScript loop, Copilot can help there too:
let numbers = [1, 2, 3, 4, 5];
numbers.forEach(num => {
console.log(num * num); // Copilot suggests fixing missing semicolon
});
Like having an additional set of eyes analyzing your code for bugs, but without tiring.
Using Copilot Chat for Debugging
It becomes cool here. Do not guess what is wrong with your code, ask Copilot via chat.
Suppose you've written a JavaScript function that fails and is showing you an error:
function greet(name) {
return "Hello, " + name;
}
console.log(greet()); // Oops! Undefined input
Instead of manually verifying what went wrong, type:
"Copilot, why is this function undefined?"
Copilot will notify you if you forgot a name default value after analyzing the code. It may even offer a fix:
function greet(name = "Guest") {
return "Hello, " + name;
}
Copilot mentors you through debugging, minimizing needless Googling.
Debugging on GitHub.com
Team projects sometimes need debugging via GitHub rather than your local IDE.
If a pull request (PR) appears odd, you can use Copilot Chat on GitHub to examine the code and offer fixes.
Function test cases needed? You may get them from Copilot. Suppose you created a Python function:
def square(n):
return n * n
Ask Copilot for test cases and it may provide this:
assert square(0) == 0
assert square(-3) == 9
assert square(2) == 4
This speeds up code review with colleagues, making it extremely helpful.
Best Practices for Debugging with Copilot
Let's discuss about optimizing GitHub Copilot debugging.
Make context clear first. Rather than typing (fix this), be specific. Say, (This function returns undefined when the input should be valid). What's wrong? Copilot's suggestions improve with additional details.
Refine and try again, second. The initial suggestion from Copilot may not be ideal. Ask for numerous solutions or approaches to a problem. You may also use /explain + /fix to diagnose the issue before fixing it.
Finally, carefully check fixes. Though strong, Copilot is still AI and not flawless. You should always test recommended fixes to avoid adding issues.
Conclusion
Do not fear debugging. The smart AI assistance in GitHub Copilot lets you troubleshoot quicker, resolve issues easily, and build test cases to avoid bugs.
Copilot makes troubleshooting in your IDE, using Copilot Chat, and evaluating pull requests on GitHub easier and less frustrating.
Do not worry if you get caught on a bug. Just ask Copilot. Who knows? You may save hours debugging!
Ready to try? Start debugging with Copilot in your IDE and experience how much simpler coding is.
239 views