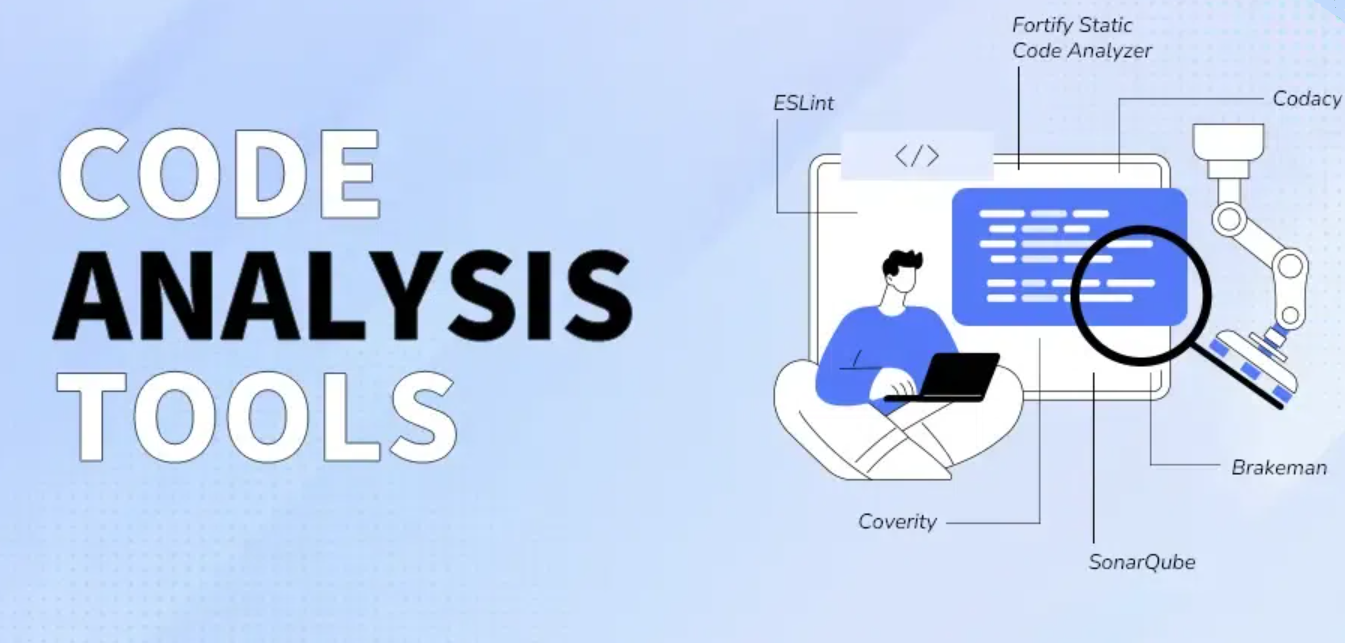
April 23, 2025
Automating Code Version Diff Analysis with DeepSeek
Automating Code Version Diff Analysis with DeepSeek
I recall carefully comparing hundreds of lines of code to detect version differences. Complex, difficult, and prone to overlooking major changes. Every codebase version matcher knows what I mean.
While helpful, traditional git diff commands lack insight. They emphasize every tiny change, even ones that do not affect functioning, making it impossible to focus on major changes. AI tools like DeepSeek make code diff analysis painless and automatic.
DeepSeek recognizes differences, not simply shows them. It differentiates between critical refactors and small formatting changes, letting developers emphasize what is important. This article shows how to create an AI-powered code diff analysis system using DeepSeek.
Challenges in Code Version Comparison
Although limited, Git version control systems monitor changes effectively. Simple git diff will show all character changes, including indentation corrections. Manually examining diffs and determining code purpose is time-consuming for big projects.
Let's see a simple Python function which finds the factorial of a number:
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
Consider someone at work optimizing the function:
from functools import lru_cache
@lru_cache(maxsize=None)
def factorial(n):
if n == 0:
return 1
return n * factorial(n - 1)
The git diff command shows the changes without explaining them or what they mean. Did things get better? Did you need it? DeepSeek uses thoughts that are aware of the context to tell the difference between big and small changes.
How DeepSeek Enhances Code Diff Analysis
Instead of dumping raw diffs, DeepSeek analyzes changes using AI. It may detect bug fixes, optimizations, or enhancements. More importantly, it provides natural language explanations to assist engineers in comprehending improvements without coding.
According to DeepSeek, optimizing the factorial function using LRU caching may enhance speed by reducing unnecessary recursive calls.
This is better than manually scanning code!
Let's use DeepSeek to create a basic automated code diff analysis system.
Building an Automated Code Diff System with DeepSeek
Step 1: Setting Up DeepSeek for Code Analysis
First we have to install DeepSeek and arrange our project surroundings. Install DeepSeek by applying:
pip install deepseek
After that, let's make a Python script to analyse code differences using DeepSeek and Git.
Step 2: Integrating with Version Control (Git)
Between Git commits, we must feed DeepSeek modifications. Python script obtains latest and previous commit diffs:
import subprocess
def get_git_diff():
result = subprocess.run(["git", "diff", "HEAD~1", "HEAD"], capture_output=True, text=True)
return result.stdout
diff_output = get_git_diff()
print(diff_output)
Here, this will provide a raw Git diff, but we need more.
Step 3: Generating AI-Powered Summaries
For analysis, send DeepSeek this diff data:
from deepseek import DeepSeek
deepseek = DeepSeek(api_key="your_api_key")
diff_output = get_git_diff()
analysis = deepseek.analyze_code_diff(diff_output)
print("AI-Powered Code Analysis:")
print(analysis["summary"])
At this step, DeepSeek tells the difference in human language.
Step 4: Filtering Significant Changes
Not all changes matter. AI filters formatting adjustments and tiny refactors.
def filter_significant_changes(analysis):
significant_changes = [change for change in analysis["changes"] if change["impact"] != "low"]
return significant_changes
filtered_changes = filter_significant_changes(analysis)
print("Filtered Changes:")
for change in filtered_changes:
print(f"- {change['description']} (Impact: {change['impact']})")
Step 5: Automating Reports and Notifications
Send Slack AI summaries following code upgrades to enhance productivity.
import requests
def send_to_slack(message):
webhook_url = "your_slack_webhook_url"
payload = {"text": message}
requests.post(webhook_url, json=payload)
summary = analysis["summary"]
send_to_slack(f"Code Update Detected:\n{summary}")
Practical Example
Suppose a developer optimizes a database query:
Old Code:
cursor.execute("SELECT * FROM users")
New Code:
cursor.execute("SELECT id, name, email FROM users WHERE active=1")
Benefits of AI-Powered Code Diff Analysis
DeepSeek automatic code diff analysis has transformed my code review. I no longer spend time on minor adjustments or strain to understand them. AI aids in prioritizing critical changes over minor refactors.
- Reduce human work in examining complicated diffs.
- Improve team cooperation with automated pull request summaries.
- Improve code quality by recognizing subtle but significant changes.
Conclusion
Reviewing code diffs manually is laborious, particularly in big projects. However, AI-powered systems like DeepSeek can automate the whole process, making it smarter, quicker, and more efficient.
We used DeepSeek and Git to evaluate code changes, filter noteworthy changes, and notify teams. This saves time, improves code, and allows cooperation.
If you use git diff alone, improve your process. Use DeepSeek to let AI analyze code diffs professionally!
333 views