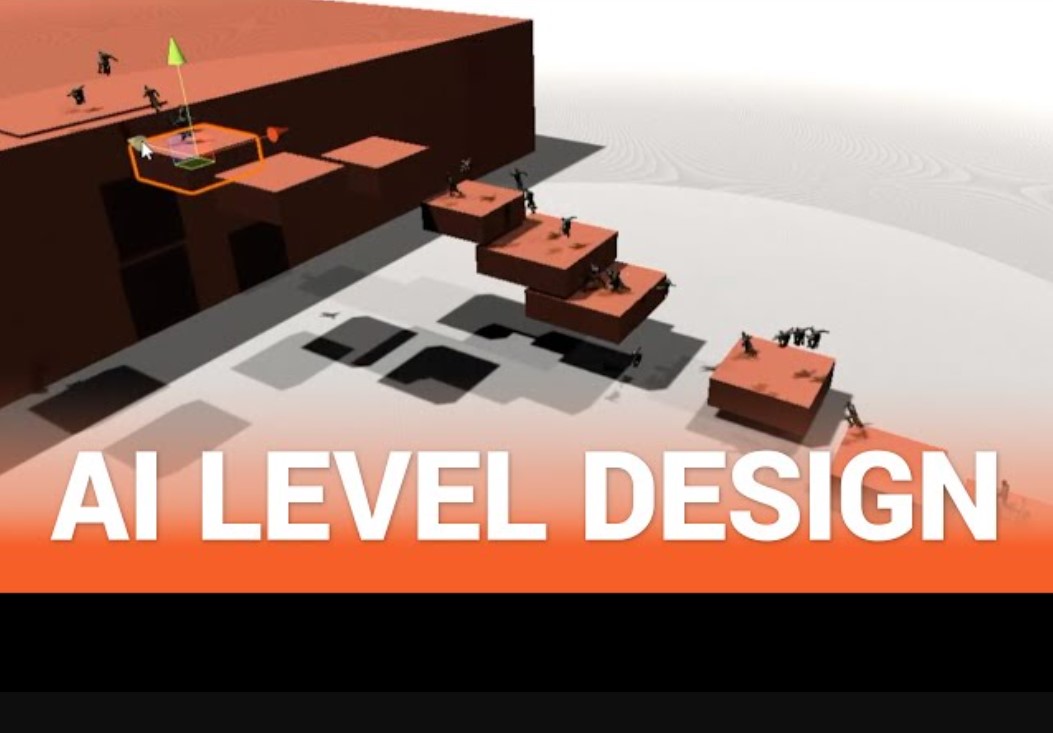
April 22, 2025
Developing an AI-Powered Game Level Generator with DeepSeek
Procedural generation allows huge, dynamic environments without manual design, changing game development. Randomly generated levels are overly easy, challenging, or boring. AI helps here. Consider a level generator that learns from player behavior, dynamically changes difficulty, and makes every replay fresh and interesting. We will construct it using DeepSeek.
My fascination with AI's ability to produce immersive gaming experiences led me to use DeepSeek to construct an AI-powered game level generator. This post will teach you how to create a level generator that generates well-balanced, playable levels without hours of human tinkering. Dive in.
Understanding DeepSeek for Procedural Generation
DeepSeek is an AI model for text production, code completion, and creative content creation. Its ability to parse patterns and create structured data makes it ideal for procedural level production. Routines like Perlin noise or cellular automata may generate random yet organized levels. But they are not adaptable enough.
With DeepSeek, we can train an AI model to comprehend level design concepts, guaranteeing that created levels are playable settings with the proper mix of challenges, rewards, and pace. Instead of conventional approaches, our AI will learn from previous levels to create dynamic, engaging experiences.
Designing an AI-Powered Level Generator
Before coding, let's explain our level generator. We need it to make a 2D platformer with terrain, adversaries, obstacles, and power-ups. We will train DeepSeek with sample levels to learn level structures and produce new ones dynamically instead of hardcoding rules.
We need a basic level array dataset. Different numbers will represent items in 2D grid levels:
- 0: Empty space
- 1: Ground
- 2: Enemy
- 3: Platform
- 4: Power-up
Training will use a basic dataset of handmade levels. First work on our AI model.
import torch
from transformers import AutoModelForCausalLM, AutoTokenizer
# Load DeepSeek model
model_name = "deepseek-ai/deepseek-coder-6.7b"
tokenizer = AutoTokenizer.from_pretrained(model_name)
model = AutoModelForCausalLM.from_pretrained(model_name)
# Example level dataset (each level is a 5x10 grid)
training_data = [
"1111111111\n0000000000\n0030002000\n0000000000\n1111111111",
"1111111111\n0003000000\n0000000200\n0000000000\n1111111111",
"1111011111\n0000030000\n0000020000\n0000000000\n1111111111"
]
# Tokenize dataset
inputs = tokenizer(training_data, return_tensors="pt", padding=True, truncation=True)
# Fine-tune the model (simplified)
outputs = model(**inputs)
loss = outputs.loss # We would optimize this loss function in a real training loop
This helps our model understand level patterns. To find common structures and level layouts, we would train DeepSeek on a larger dataset.
Balancing Difficulty and Playability
Randomly generated levels may be too easy or hard. Dynamic difficulty balance fixes this. The AI will assess player performance and establish subsequent levels. Using player success, we may add a difficulty score to opponent positioning and platform gaps.
def adjust_difficulty(level, player_skill):
new_level = []
for row in level.split("\n"):
new_row = ""
for tile in row:
if tile == "2" and player_skill < 5: # Remove enemies for new players
new_row += "0"
elif tile == "3" and player_skill > 8: # Add more platforms for skilled players
new_row += "3"
else:
new_row += tile
new_level.append(new_row)
return "\n".join(new_level)
# Simulate player skill level (1-10)
player_skill = 7
new_level = adjust_difficulty(training_data[0], player_skill)
print(new_level)
This maintains levels difficult for experts and avoids impossible levels for beginners.
Coding a Basic Level Generator with DeepSeek
Now that DeepSeek knows level structures, we can construct levels on demand. A prompt-based strategy will provide DeepSeek a partial level to finish.
def generate_level(prompt):
input_ids = tokenizer(prompt, return_tensors="pt").input_ids
output = model.generate(input_ids, max_length=50, pad_token_id=tokenizer.eos_token_id)
return tokenizer.decode(output[0], skip_special_tokens=True)
# Prompt DeepSeek with a partial level
prompt = "1111111111\n0000000000\n00"
generated_level = generate_level(prompt)
print("Generated Level:\n", generated_level)
DeepSeek creates levels dynamically, providing diversity and logic.
Testing and Refining the AI-Generated Levels
No AI system starts perfect. We must test our levels' fun and playability. Simulations may expose dead ends, unreachable stages, and unfair difficulty spikes.
def validate_level(level):
rows = level.split("\n")
ground_found = any("1" in row for row in rows) # Ensure at least some ground exists
if not ground_found:
return False, "No ground detected"
return True, "Level is playable"
is_valid, message = validate_level(generated_level)
print("Validation Result:", message)
This basic check prevents DeepSeek from creating broken levels. To validate completion rates, AI-driven playtesting is possible.
Conclusion
An AI-powered level generator using DeepSeek was fun to build. We used AI to recognize trends and change dynamically to understand design principles and player skill levels in our procedural generator.
This method might construct game environments in real time based on user response with further training. AI-driven procedural generation is the future of game creation, and DeepSeek is helping ensure it.
If you are a game creator considering AI, try DeepSeek. Let AI-generated content replace static level design.
285 views