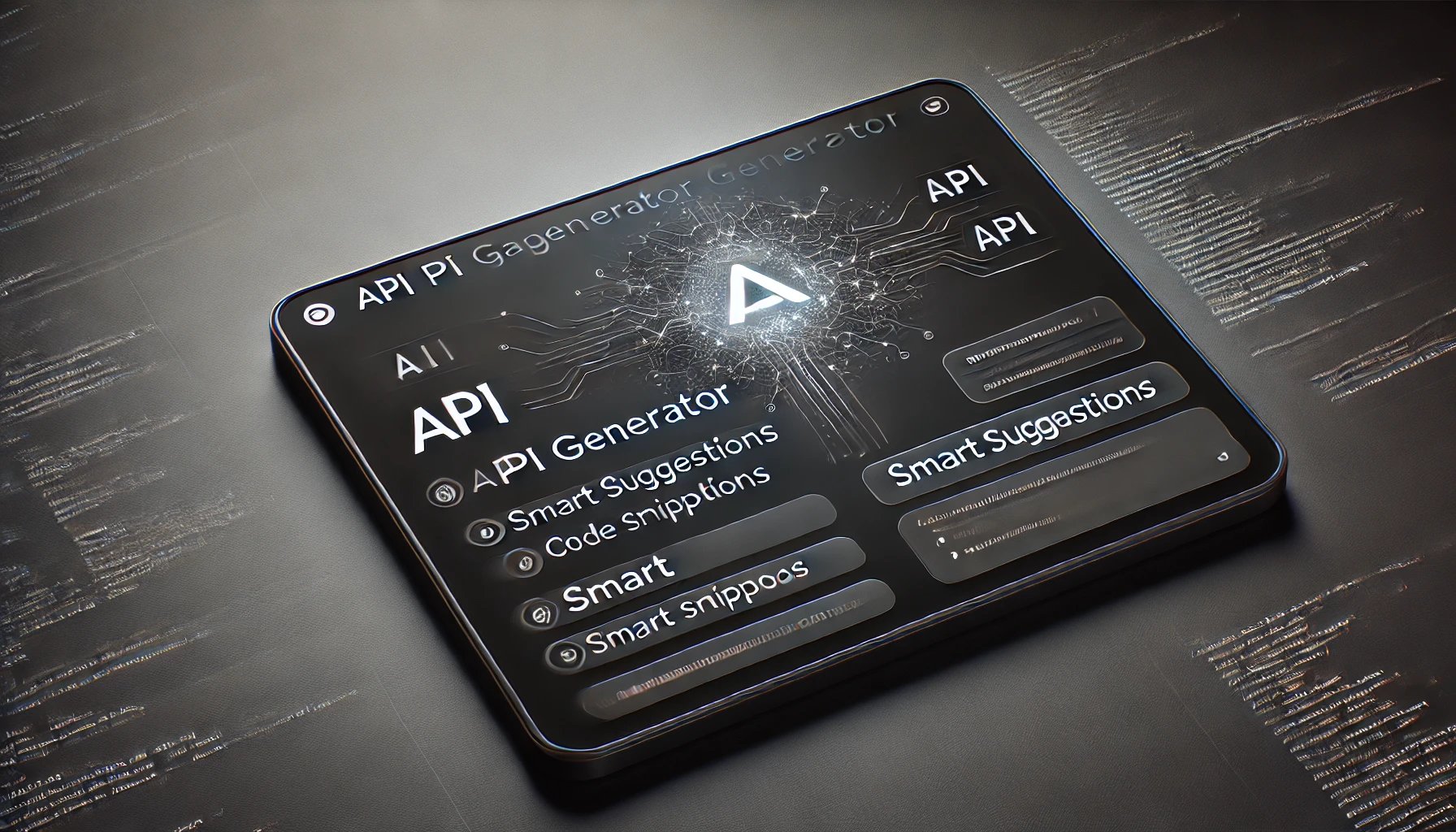
April 21, 2025
Building a Smart API Endpoint Generator with DeepSeek
APIs provide smooth service integration for modern web and mobile applications. However, manually designing, monitoring, and documenting RESTful API endpoints is difficult. It is easy to forget endpoints, documentation, or updates. Developers desire a better approach to automate API endpoint creation and documentation without spending hours writing repetitive code.
Here comes DeepSeek. AI can create a smart API endpoint generator that evaluates project structure and extracts routes and documents. Let's start from scratch to make this tool.
Understanding DeepSeek for API Generation
DeepSeek is ideal for automating repeating development processes as it can evaluate and understand structured data. We can teach DeepSeek to scan project files, discover route patterns, and construct API endpoints without human definition.
In Node.js and Express, routes are defined in routes/user.js or controllers/userController.js. DeepSeek can read these files, find route patterns (GET /users, POST /users), and provide a structured API list.
By using DeepSeek, we get:
- Automatic route tracking helps us to avoid personally monitoring endpoints.
- Real-time updates, so the API list is always up to date.
- We do not have to keep track of changes to project files because the documentation is always the same. No more API documentation that is out of date
Let's make a tool that will do this for us step by step.
Designing the API Endpoint Generator
We need to do the following to make our API generator:
- Read the project's path and pull out the necessary route files.
- You can read those files to find defined routes.
- Put the data you extracted into an organized file for API documentation.
We will use Node.js, Express, and DeepSeek to do this.
Step 1: Setting Up the Project
To start, let's set up our Node.js project and add the files we need:
mkdir api-endpoint-generator
cd api-endpoint-generator
npm init -y
npm install express fs deepseek
We will also make a few basic files:
mkdir routes controllers
touch index.js generator.js
Step 2: Extracting API Endpoints
We are going to make a tool in generator.js that will automatically look through the routes/ directory and extract endpoints.
Example routes/user.js file:
const express = require('express');
const router = express.Router();
router.get('/users', (req, res) => {
res.send('Get all users');
});
router.post('/users', (req, res) => {
res.send('Create a user');
});
router.get('/users/:id', (req, res) => {
res.send(`Get user with ID ${req.params.id}`);
});
module.exports = router;
Now, it's time to write a script that can extract routes automatically.
We are going to read files from the routes/ folder, figure out what they mean, and use generator.js to find endpoints.
const fs = require('fs');
const path = require('path');
function extractRoutes() {
const routesDir = path.join(__dirname, 'routes');
const routeFiles = fs.readdirSync(routesDir);
let endpoints = [];
routeFiles.forEach((file) => {
const filePath = path.join(routesDir, file);
const content = fs.readFileSync(filePath, 'utf-8');
const routeRegex = /router\.(get|post|put|delete)\(['"](.+?)['"]/g;
let match;
while ((match = routeRegex.exec(content)) !== null) {
endpoints.push({ method: match[1].toUpperCase(), path: match[2] });
}
});
return endpoints;
}
module.exports = extractRoutes;
Automating API Documentation
We need to turn the API endpoints into organized API documentation after we have extracted them. Swagger (OpenAPI) is popular for this.
Step 3: Generating Swagger Docs
Let's add the following dependencies to our Swagger project:
npm install swagger-jsdoc swagger-ui-express
After that, we will add Swagger to index.js:
const express = require('express');
const swaggerJsDoc = require('swagger-jsdoc');
const swaggerUi = require('swagger-ui-express');
const extractRoutes = require('./generator');
const app = express();
const PORT = 5000;
// Extract API routes dynamically
const endpoints = extractRoutes();
// Generate Swagger JSON dynamically
const swaggerOptions = {
definition: {
openapi: '3.0.0',
info: {
title: 'Auto-Generated API Docs',
version: '1.0.0',
},
},
apis: [],
};
// Convert extracted endpoints to Swagger format
swaggerOptions.paths = endpoints.reduce((acc, route) => {
acc[route.path] = {
[route.method.toLowerCase()]: {
description: `Auto-generated endpoint for ${route.method} ${route.path}`,
responses: {
200: { description: 'Success' },
},
},
};
return acc;
}, {});
const swaggerDocs = swaggerJsDoc(swaggerOptions);
app.use('/api-docs', swaggerUi.serve, swaggerUi.setup(swaggerDocs));
// Start server
app.listen(PORT, () => console.log(`Server running on http://localhost:${PORT}`));
Running our application will automatically document all discovered routes in Swagger.
node index.js
Check out the appealing auto-generated API documentation at http://localhost:5000/api-docs!
Implementing the Generator in a Real Project
The generator fits well into existing projects. Insert generator.js and edit index.js. Apply it in real life:
- After adding generator.js to your server code, you can drop the script into your project.
- Run the generator to scan the routes/directory and build endpoints.
- You can automate CI/CD documentation changes by running node generator.js before release.
For every new API, this method changes the documentation instantly.
Conclusion
DeepSeek automates repetitious activities, speeding and legitimizing API endpoint generator development. We looked through project files, extracted routes, and made Swagger documentation on the fly. This prevents errors, ensures consistency, and changes API documentation.
Future upgrades may include GraphQL, AI-powered validation, and real-time monitoring. But for now, we have a strong technology that saves hours of laborious effort!
Do you want this generator for Django or Laravel? Let me know!
286 views