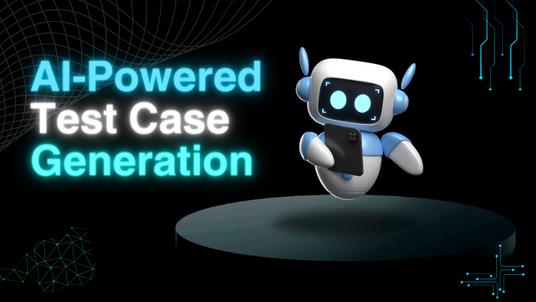
April 17, 2025
Developing an AI-Powered Test Case Generator with DeepSeek
Writing test cases is a vital but tiresome software development process. We all know how important they are for early bug detection, stability, and code reliability. Manually writing unit and integration tests requires time, effort, and patience.
Here comes AI-powered test case creation. Imagine an AI that reads your code, figures out what might go wrong, and makes test cases. DeepSeek can make this possible. AI from DeepSeek automates unit and integration testing across programming languages. This post will show you how DeepSeek can help you write tests, saving you hours and making sure you cover everything.
Understanding DeepSeek for Test Case Generation
AI-powered DeepSeek produces, refactors, and debugs code; as it checks the code's structure and includes logical test cases, it is useful for testing. DeepSeek understands context and adapts to Python, Java, JavaScript, and others.
DeepSeek performs unit and integration tests to ensure software module compatibility. If this works, our testing process will be much improved, and we will not miss any critical errors. Best part? DeepSeek handles recurring test cases for us.
Step 1: Setting Up DeepSeek for Test Generation
We must install DeepSeek before producing test cases. We will install dependencies and enable API access for DeepSeek.
Installing DeepSeek API and Dependencies
First, install the required Python libraries:
pip install requests pytest deepseek
Sign up on DeepSeek and get your API key for authentication. After that you can store it in an environment variable:
export DEEPSEEK_API_KEY="your_api_key_here"
You may also set the API key in your script:
DEEPSEEK_API_KEY = "your_api_key_here"
Step 2: Generating Unit Test Cases with DeepSeek
It is essential to verify individual functions using unit tests. Instead of making each test, we can use DeepSeek to automate this process.
Writing a Sample Function
A simple prime number checker would look like this:
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
Using DeepSeek to Generate Test Cases
Writing a script to submit our function to DeepSeek and obtain AI-generated test cases:
import requests
DEEPSEEK_API_URL = "https://api.deepseek.com/generate-test"
DEEPSEEK_API_KEY = "your_api_key_here"
def generate_test_cases(code_snippet):
headers = {"Authorization": f"Bearer {DEEPSEEK_API_KEY}"}
payload = {
"code": code_snippet,
"language": "python",
"test_type": "unit"
}
response = requests.post(DEEPSEEK_API_URL, headers=headers, json=payload)
if response.status_code == 200:
return response.json()["test_cases"]
else:
return f"Error: {response.text}"
# Send our function to DeepSeek
code_snippet = """
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
"""
test_cases = generate_test_cases(code_snippet)
print("Generated Test Cases:\n", test_cases)
Running the Generated Test Cases
Assume DeepSeek delivers these test cases:
import unittest
from my_module import is_prime
class TestPrimeFunction(unittest.TestCase):
def test_prime_numbers(self):
self.assertTrue(is_prime(7))
self.assertTrue(is_prime(13))
self.assertTrue(is_prime(29))
def test_non_prime_numbers(self):
self.assertFalse(is_prime(4))
self.assertFalse(is_prime(9))
self.assertFalse(is_prime(15))
def test_edge_cases(self):
self.assertFalse(is_prime(1))
self.assertFalse(is_prime(0))
self.assertFalse(is_prime(-5))
Save the generated test cases in a file and run:
pytest test_prime.py
Step 3: Generating Integration Test Cases with DeepSeek
Unit tests are wonderful for separated functions, but real-world applications contain several integrated components. Integration tests verify these components' interactions.
Writing a Sample Multi-Component System
Start with a two-function order processing system:
def calculate_total(prices):
return sum(prices)
def apply_discount(total, discount_percentage):
return total - (total * (discount_percentage / 100))
Using DeepSeek to Generate Integration Test Cases
Now, sending this code to DeepSeek using the same API method:
code_snippet = """
def calculate_total(prices):
return sum(prices)
def apply_discount(total, discount_percentage):
return total - (total * (discount_percentage / 100))
"""
test_cases = generate_test_cases(code_snippet)
print("Generated Integration Test Cases:\n", test_cases)
Running the Generated Integration Tests
Assume DeepSeek produces these tests:
import unittest
from order_system import calculate_total, apply_discount
class TestOrderProcessing(unittest.TestCase):
def test_order_with_discount(self):
total = calculate_total([10, 20, 30])
discounted_total = apply_discount(total, 10)
self.assertEqual(discounted_total, 54)
def test_order_without_discount(self):
total = calculate_total([15, 25])
self.assertEqual(total, 40)
def test_zero_discount(self):
total = calculate_total([100, 200])
discounted_total = apply_discount(total, 0)
self.assertEqual(discounted_total, 300)
Run the tests with:
pytest test_order_processing.py
Step 4: Optimizing AI-Generated Test Cases
DeepSeek generates test cases, but AI is not flawless. Best practices for improving its output:
- Always review AI-generated test cases for missing edge situations.
- Add important tests manually for odd cases that AI might miss in the real world.
- Create DeepSeek test cases automatically in CI/CD processes to test all the time.
Conclusion
DeepSeek excels in automating test case generation for developers. AI can automate the process of creating integration and unit tests. There is always room for improvement in AI-generated tests, but they do improve test coverage with little effort.
Experience the power of AI-driven test generation right now. While AI is testing, you may automate your test cases, set up DeepSeek, and create impressive code.
73 views