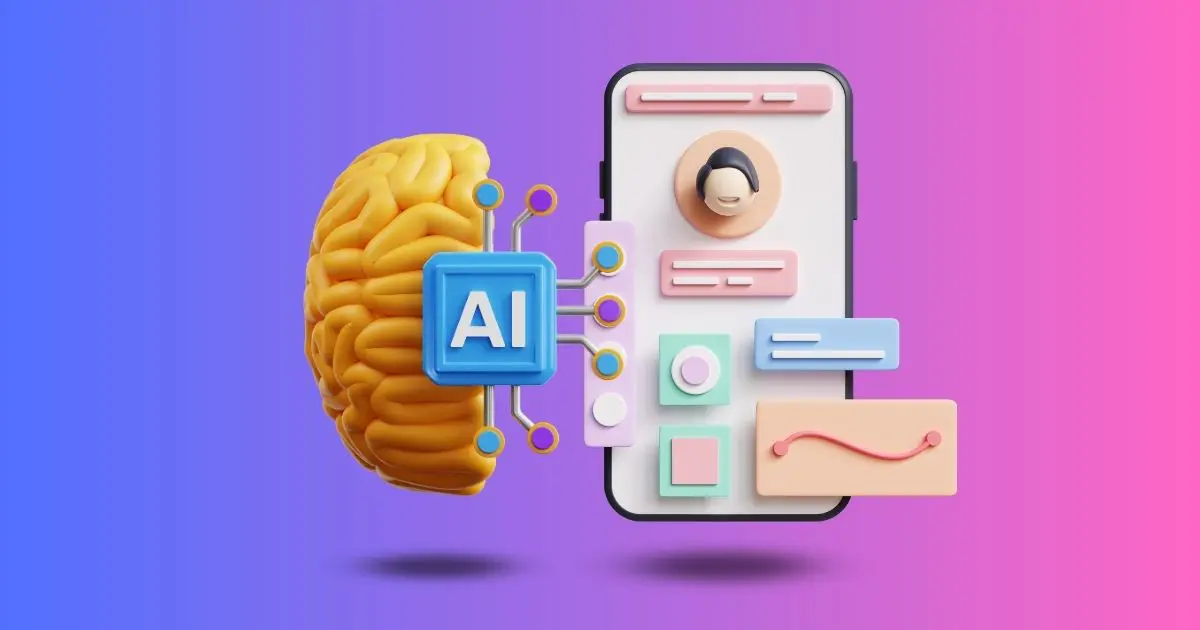
April 16, 2025
AI-Assisted UI Component Generator with DeepSeek
Do you ever think that making UIs could be easier and less repetitive? UI design, tweaking, and improvement are something I do all the time as a coder, and I have to do it all over again for the next task. DeepSeek creates UI components from minimal design guidelines using AI.
You might enter "A sleek, responsive button with smooth sides and a hover effect" and receive the correct React, Vue, or Tailwind component immediately. No more manual or repeated design changes, DeepSeek handles it all.
This article will explain why AI-driven UI generation is a big deal, how DeepSeek works, and how you can use it to make your development process faster. I will also give you a fully functional React implementation so you can start using DeepSeek now.
Why Use AI for UI Component Generation?
Anyone who has built UI elements from the start knows how long it can take. First, you write HTML. Then, you add CSS to style it and write JavaScript to make it work with other programs. It takes a lot of trial and error to get everything right, even with tools like React or Vue.
Here comes AI. Based on design cues, AI can swiftly construct UI components without coding. This saves time and standardizes tasks. DeepSeek generates optimized, best-practice code without mismatched styles or wasteful CSS classes.
Another big benefit is that it is easy to access. AI components may fulfill accessibility criteria, reducing the need for further inspections. AI lets us focus on logic and user experience rather than UI details, which helps on tight deadlines.
Understanding DeepSeek's Capabilities
DeepSeek is not only a code creator but also a smart AI that knows how designs work. It does not just outputs HTML and CSS; instead, it understands what the prompts mean and makes parts that work with current design systems.
If I tell DeepSeek, "Give me a card component with a shadow, rounded sides, and a button inside," it will not just give me a simple div. Instead, it will make organized, adaptable code that makes sure the card looks great on all screen sizes.
DeepSeek works with a lot of different systems. It changes based on your needs, whether you need a working React component, a single-file Vue component, or code in the Tailwind way. These features make it a useful addition to any UI process.
Setting Up DeepSeek for UI Code Generation
DeepSeek works best with a basic working setup that includes Node.js and a front-end framework like React or Vue. The process of setting up is simple:
Step 1: Install Dependencies
Install Node.js first, and then make a React project if you do not already have one:
npx create-react-app deepseek-ui
cd deepseek-ui
npm install axios
Step 2: Create a DeepSeek API Utility File
The deepseekService.js file will handle API requests. Make it in the src folder.
import axios from "axios";
// Replace with your DeepSeek API Key
const API_KEY = "YOUR_DEEPSEEK_API_KEY";
const API_URL = "https://api.deepseek.com/generate";
/**
* Function to generate UI components from DeepSeek
* @param {string} prompt - The design prompt
* @returns {Promise<string>} - Generated React component code
*/
export const generateUIComponent = async (prompt) => {
try {
const response = await axios.post(
API_URL,
{ prompt }, // Sending the user prompt
{
headers: {
"Content-Type": "application/json",
"Authorization": `Bearer ${API_KEY}`, // Authenticate request
},
}
);
return response.data.code; // Return AI-generated component code
} catch (error) {
console.error("Error generating UI component:", error);
return null;
}
};
Generating UI Components with DeepSeek
DeepSeek's real power comes from how well it knows what the designer meant. I do not have to code components; I can just tell DeepSeek what I need, and it will find the best match.
Now, make changes to App.js so that it calls DeepSeek's API and shows the created UI component.
import React, { useState } from "react";
import { generateUIComponent } from "./deepseekService";
const App = () => {
const [prompt, setPrompt] = useState("");
const [generatedCode, setGeneratedCode] = useState("");
const [Component, setComponent] = useState(null);
// Handle API call when user submits a prompt
const handleGenerate = async () => {
if (!prompt) return;
const code = await generateUIComponent(prompt);
if (code) {
setGeneratedCode(code);
// Convert the generated code into a usable React component
const ComponentModule = new Function(`return ${code}`)();
setComponent(() => ComponentModule);
}
};
return (
<div style={{ padding: "20px", fontFamily: "Arial" }}>
<h1>AI UI Component Generator</h1>
<textarea
rows="3"
cols="50"
placeholder="Enter your UI description..."
value={prompt}
onChange={(e) => setPrompt(e.target.value)}
></textarea>
<br />
<button onClick={handleGenerate} style={{ marginTop: "10px" }}>
Generate Component
</button>
<h2>Generated UI Component:</h2>
<div style={{ border: "1px solid #ddd", padding: "10px", marginTop: "10px" }}>
{Component ? <Component /> : "No component generated yet."}
</div>
<h2>Generated Code:</h2>
<pre style={{ backgroundColor: "#f4f4f4", padding: "10px", overflow: "auto" }}>
{generatedCode || "No code generated yet."}
</pre>
</div>
);
};
export default App;
Refining AI-Generated Components
Components made by AI are not always perfect when they come out of the box. To improve aesthetics, accessibility, or design system match, DeepSeek needs manual adjustment.
To make my button look like it fits with Material Design, I might need to change the shadow level, the border radius, or add movements. In the same way, I can change class names to match current styles if my project has its design system.
What is the main point? DeepSeek does not replace coders; instead, it makes our work easier. AI gives us a good place to start, but human imagination and skill are still needed to finish the task.
Conclusion
DeepSeek is changing front-end development by making it easier, faster, and more efficient to make UI components. Simply define what I need, and DeepSeek generates clean, production-ready code without hours of design.
AI will improve UI generating tools that understand complete design systems, offer real-time modification, and integrate straight into Figma or Sketch processes. Front-end development has arrived at its future, and AI-powered UI creation is just the beginning.
If you are a developer who wants to get things done faster, you should definitely try DeepSeek. You can spend the time you save on routine UI code on more important things, like making great user experiences.
86 views