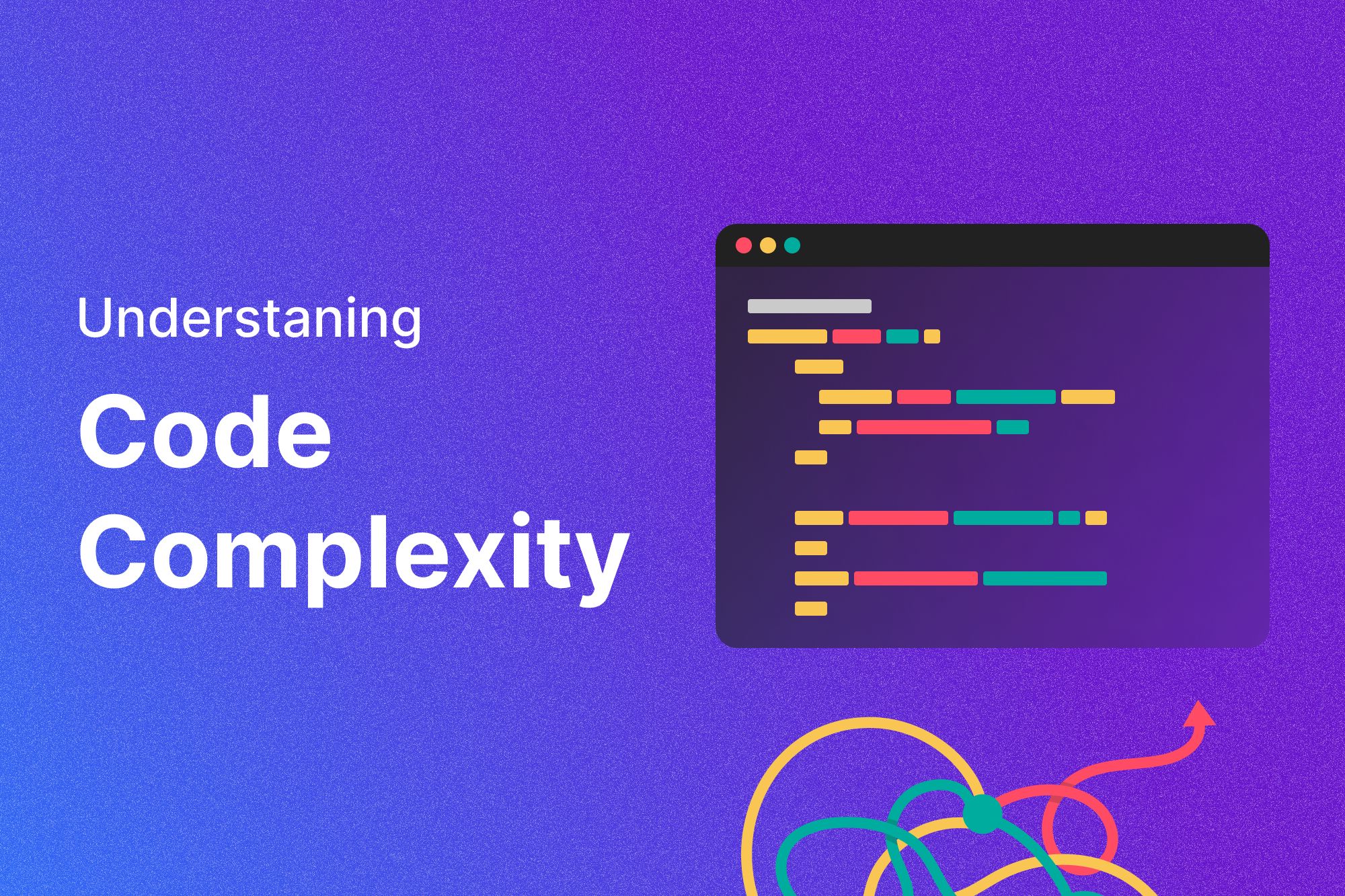
April 11, 2025
AI-Powered Code Complexity Analyzer Using DeepSeek-Code
Have you ever tried to analyze a mess of code like a detective? I am here for you. Software developers struggle with complex code, which affects performance and troubleshooting. Build efficient, clean, and manageable code for long-term flexibility and lesser technical complexity.
Developers often use static analysis tools or check the code manually to find out how complex it is to understand. But what if AI did all the hard work? A new AI model called DeepSeek-Code looks at how complex code is and suggests ways to make it better on its own. This article will show how code complexity impacts development, how DeepSeek-Code simplifies analysis, and how to write smarter and faster.
Understanding Code Complexity
Code complexity is not just about large functions or complicated loops; it measures how hard code is to interpret, edit, and maintain. Deeply layered structures, duplicated logic, and unnecessary dependencies increase complexity.
Cyclomatic Complexity, which counts program-independent pathways, is a popular metric. When things are complex then there are chances mistakes happen more often and take longer to fix. Halstead Complexity measures the number of operators and operands, while the Maintainability Index measures how easy it will be to make changes in the future.
High complexity slows development, introduces errors, and makes collaboration difficult. SonarQube and ESLint provide basic complexity analysis but insufficient contextual awareness. AI technologies like DeepSeek-Code excel there.
DeepSeek-Code: An AI-Powered Solution
DeepSeek-Code does more than just look at code. It looks at code, finds trends, and uses AI to make smart ideas. DeepSeek-Code analyses logic rather than rules, providing insights matched to your coding approach.
This AI-reviewer tool finds complex reasoning and suggests ways to make it easier to understand. DeepSeek-Code optimizes refactoring ideas for deeply nested functions and unnecessary computations, making code lighter and more legible.
Building an AI-Powered Code Complexity Analyzer
Integrating DeepSeek-Code into your development routine is amazingly easy. A simple Python-based AI-powered code complexity analyzer:
Step 1: Setting Up the Environment
We need to set up our project and load libraries before we can start. If DeepSeek-Code has an API or a local model, we must install its SDK.
Installing Dependencies
pip install deepseek-code requests
Requests send out DeepSeek-Code API queries hosted in the cloud. Installing a DeepSeek type may change depending on your location.
Step 2: Writing Code for Complexity Analysis
Write a script to rate Python functions' difficulty.
Simple Code Complexity Analyzer
import deepseek_code
def analyze_code_complexity(code):
"""
Sends the given code to DeepSeek-Code for complexity analysis.
"""
response = deepseek_code.analyze(code) # Assuming DeepSeek has an analyze function
return response
# Sample code for analysis
sample_code = """
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
"""
# Analyze the sample code
complexity_report = analyze_code_complexity(sample_code)
print(complexity_report)
Step 3: Processing DeepSeek-Code Output
DeepSeek-Code may respond as follows:
{
"cyclomatic_complexity": 5,
"maintainability_index": 65,
"optimization_suggestions": [
"Consider using an iterative approach instead of recursion for factorial function.",
"Reduce function call depth to improve performance."
]
}
Modify our script to process and show this information clearly.
Processing and Displaying Complexity Results
def display_complexity_report(report):
"""
Formats and prints the complexity report in a readable way.
"""
print("\nCode Complexity Report:")
print(f"Cyclomatic Complexity: {report['cyclomatic_complexity']}")
print(f"Maintainability Index: {report['maintainability_index']}")
print("\nOptimization Suggestions:")
for suggestion in report["optimization_suggestions"]:
print(f"- {suggestion}")
# Analyze and display results
complexity_report = analyze_code_complexity(sample_code)
display_complexity_report(complexity_report)
Step 4: Refactoring the Code Using AI Suggestions
DeepSeek Suggestion: Replace Recursion with Iteration
The AI suggests iterating the recursive factorial algorithm for greater performance.
Before Optimization (Recursive Approach)
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
After Optimization (Iterative Approach)
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Removing recursion accelerates performance and lowers function call overhead.
Step 5: Automating the Refactoring Process
AI can automate code editing. Build a function that applies DeepSeek-Code's ideas and basic transformations.
Automating Code Refactoring with AI
def apply_ai_suggestions(code, suggestions):
"""
Uses AI suggestions to refactor the code automatically.
"""
if "Consider using an iterative approach" in suggestions[0]:
code = """
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
"""
return code
# Apply AI-powered optimization
optimized_code = apply_ai_suggestions(sample_code, complexity_report["optimization_suggestions"])
print("\nOptimized Code:\n", optimized_code)
How It Works
- Automatically processes AI ideas and implements modifications.
- This substitutes iteration based on the AI report for recursion.
Final Output: Full Workflow
A whole script run would look like this:
Code Complexity Report:
Cyclomatic Complexity: 5
Maintainability Index: 65
Optimization Suggestions:
- Instead of recursion, use iteration for factorial function.
- Reduce the depth of function calls to increase effectiveness.
Optimized Code:
def factorial_iterative(n):
result = 1
for i in range(1, n + 1):
result *= i
return result
Conclusion
DeepSeek-Code automates code complexity analysis, interprets AI-powered optimization recommendations, and refactors code based on intelligent insights. AI can help us in code writing and reduce bug fixing and improvement time. Future writing will involve using AI to write better code. Let me know if you want me to develop an analyzer that uses a graphical user interface.
101 views