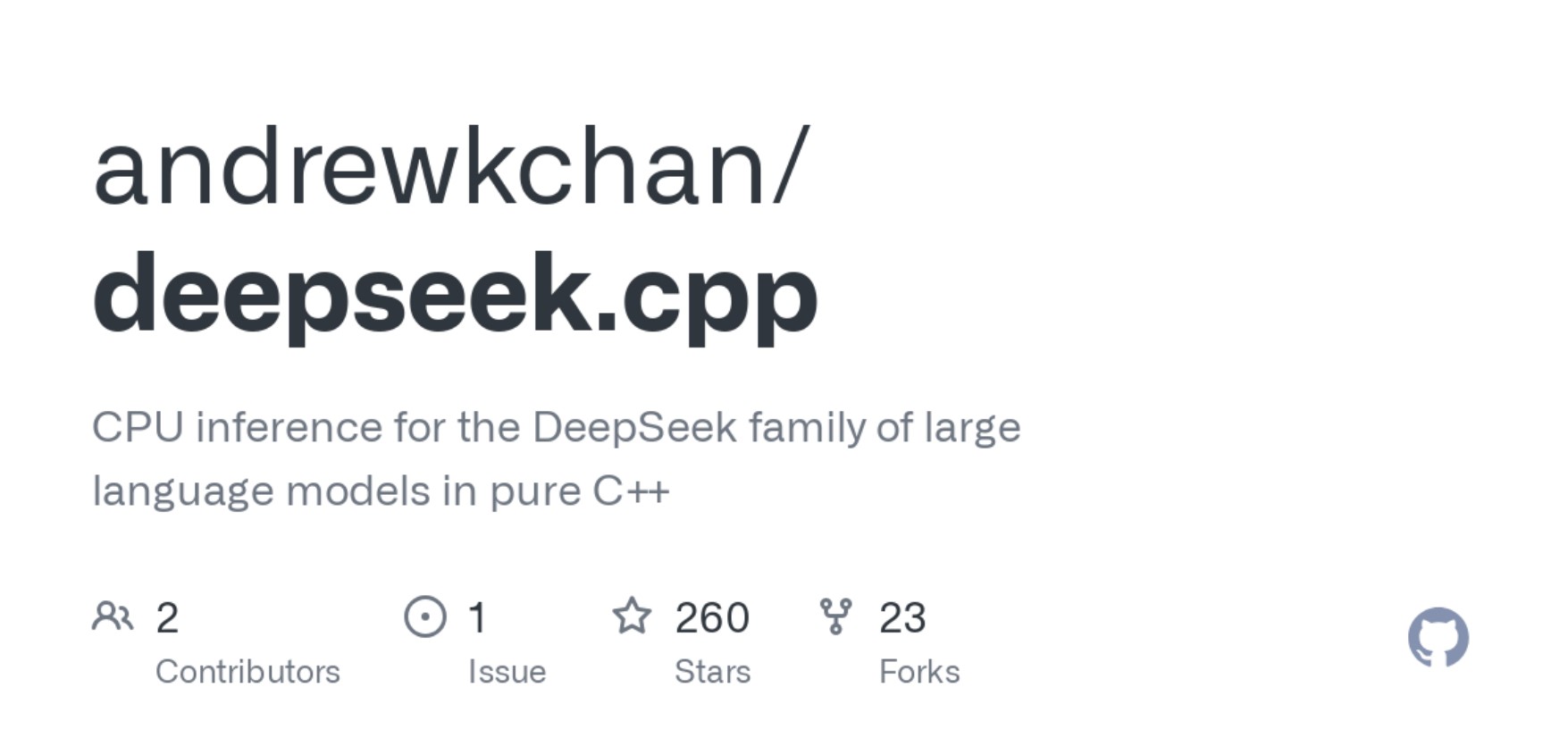
March 19, 2025
DeepSeek.cpp: Running DeepSeek LLMs on CPU with C++ for Efficient Inference
Ever wondered whether you could run complex AI models without a GPU? Here comes DeepSeek.cpp. The C++-based CPU-only inference engine for the DeepSeek series of large language models is based on Yet Another Language Model. You can deploy and execute LLMs effectively on regular hardware without costly graphics cards.
DeepSeek.cpp supports DeepSeek-V2-Lite, V2, V2.5, V3, and R1. This approach enables LLMs simple to use in AI-driven apps, chatbots, and automation tools without GPU limitations. Let's discover why this tool is worth examining.
Why Use DeepSeek.cpp?
AI models run on CPUs instead of GPUs are frequently considered a compromise, but DeepSeek.cpp challenges that. Developers seeking a lightweight, scalable, and accessible inference solution should consider it since it is fast and efficient.
Its C++ code allows lower-level optimizations, making it quicker and less memory-intensive than Python-based competitors. Most crucially, it does not need CUDA or GPU acceleration, making it appropriate for devices without GPUs.
Since it is open-source, DeepSeek.cpp is customizable. You have complete code control to change its design, incorporate it into a bigger system, or try new optimization methods.
Setting Up DeepSeek.cpp
Simply follow these steps to start using DeepSeek.cpp. For starters, you need to get a C++ compiler (like GCC, Clang, or MSVC) and CMake to set up the build. If you are using Linux, you might need to add libraries like OpenBLAS or Eigen.
To install, just clone the repository and build it from scratch:
git clone https://github.com/deepseek-ai/deepseek.cpp.git
cd deepseek.cpp
mkdir build && cd build
cmake ..
make -j$(nproc)
Run a sample inference after compiling to verify installation.
Using DeepSeek.cpp for Inference
Now comes the fun: LLM-generated text using DeepSeek.cpp.
Loading a Model
So, let's first load a DeepSeek model:
#include "deepseek.h"
int main() {
DeepSeekModel model;
if (!model.load("models/deepseek-v2.bin")) {
std::cerr << "Failed to load model" << std::endl;
return -1;
}
std::cout << "Model loaded successfully!" << std::endl;
return 0;
}
This checks model loading before reasoning.
Running an Inference Query
With the model loaded, we can generate prompt-based text:
std::string input_text = "What is DeepSeek?";
std::string output_text = model.generate(input_text);
std::cout << "Model Output: " << output_text << std::endl;
Show the answer or use it elsewhere in your program.
Optimizing Performance
Using batch processing to handle many queries at once can speed things up:
std::vector<std::string> batch_inputs = {"What is AI?", "How does deep learning work?"};
auto results = model.batch_generate(batch_inputs);
for (const auto& res : results) {
std::cout << res << std::endl;
}
Multi-threading helps optimize CPU core use for high-query workloads. Even though DeepSeek.cpp does not include threading, you can parallelize workloads using C++ standard threading or OpenMP.
Comparing DeepSeek.cpp with Other Implementations
Some may compare DeepSeek.cpp to other inference algorithms. Low-level optimizations make DeepSeek.cpp faster on CPUs than PyTorch-based inference. PyTorch is great for prototypes and GPU acceleration, however DeepSeek.cpp is better for lightweight, independent solutions.
Using ggml-based models will also show discrepancies. Quantized models work well with ggml, although DeepSeek.cpp enables full precision inference, which may enhance accuracy but use more memory.
DeepSeek.cpp allows GPU-free generation of AI models quickly and realistically.
Future Improvements & Community Support
The DeepSeek.cpp evolves. Quantization to save memory utilization, multi-threading for parallel processing, and model compatibility may be optional.
Best part? You can help! Developers may optimize, report bugs, and enhance the open-source project on GitHub.
Conclusion
CPU-based DeepSeek LLMs are strong and efficient using DeepSeek.cpp. C++ optimizations reduce the need for costly GPUs while giving decent performance. DeepSeek.cpp lets you run novel artificial intelligence on accessible hardware for chatbots, automation tools, and embedded system language models.
DeepSeek.cpp lets you deploy big language models without hardware constraints. Try it, play with the code, and find how far CPUs can push AI!
307 views