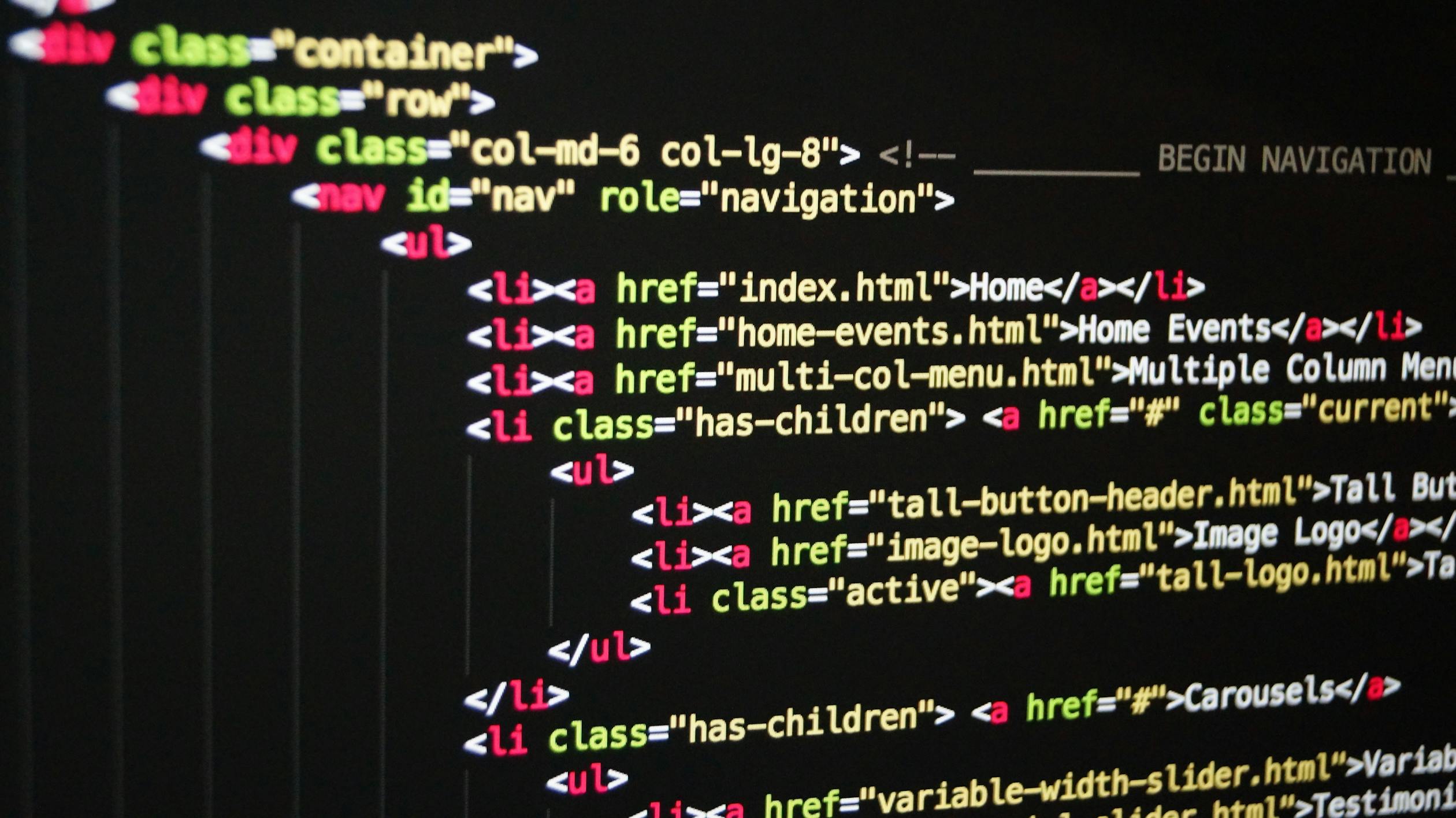
Share what you learn in this blog to prepare for your interview, create your forever-free profile now, and explore how to monetize your valuable knowledge.
Array.prototype.map() is a built-in method in JavaScript that allows you to create a new array by applying a provided function to each element of an existing array. It's a powerful tool for transforming and manipulating data within an array.
Syntax:
const newArray = originalArray.map(callback(element[, index[, array]]) {
// Your transformation logic here
return transformedElement;
});
- originalArray: The array you want to transform.
- callback: A function that is applied to each element in the array.
- element: The current element being processed.
- index (optional): The index of the current element being processed.
- array (optional): The array map was called upon.
Example 1: Doubling Numbers
Let's say we have an array of numbers, and we want to create a new array with each number doubled.
const originalArray = [1, 2, 3, 4, 5];
const newArray = originalArray.map(function(element) {
return element * 2;
});
console.log(newArray); // Output: [2, 4, 6, 8, 10]
In this example, we:
- Define the originalArray, which contains our initial data.
- Use to create a new array (newArray).
- Inside the map() method, we provide a callback function that doubles each element (element * 2).
Example 2: Uppercasing Strings
Now, let's consider an array of strings, and we want to create a new array with each string converted to uppercase.
const originalArray = ["apple", "banana", "cherry", "date"];
const newArray = originalArray.map(function(element) {
return element.toUpperCase();
});
console.log(newArray); // Output: ["APPLE", "BANANA", "CHERRY", "DATE"]
In this example, we:
- Define the originalArray, which contains an array of strings.
- Use map() to create a new array (newArray).
Inside the map() method, we provide a callback function that converts each string to uppercase using toUpperCase().
Using Arrow Functions (Optional):
You can use arrow functions for more concise code:
const originalArray = [1, 2, 3, 4, 5];
const doubledArray = originalArray.map(element => element * 2);
const stringArray = originalArray.map(element => element.toUpperCase());
Additional Notes:
- The original array remains unchanged. map() returns a new array with the transformed values.
- You can use the index and array parameters in your callback function for more advanced transformations.
- The result of the map() method can be stored in a new variable, as shown in the examples.
464 views
Please Login to create a Question