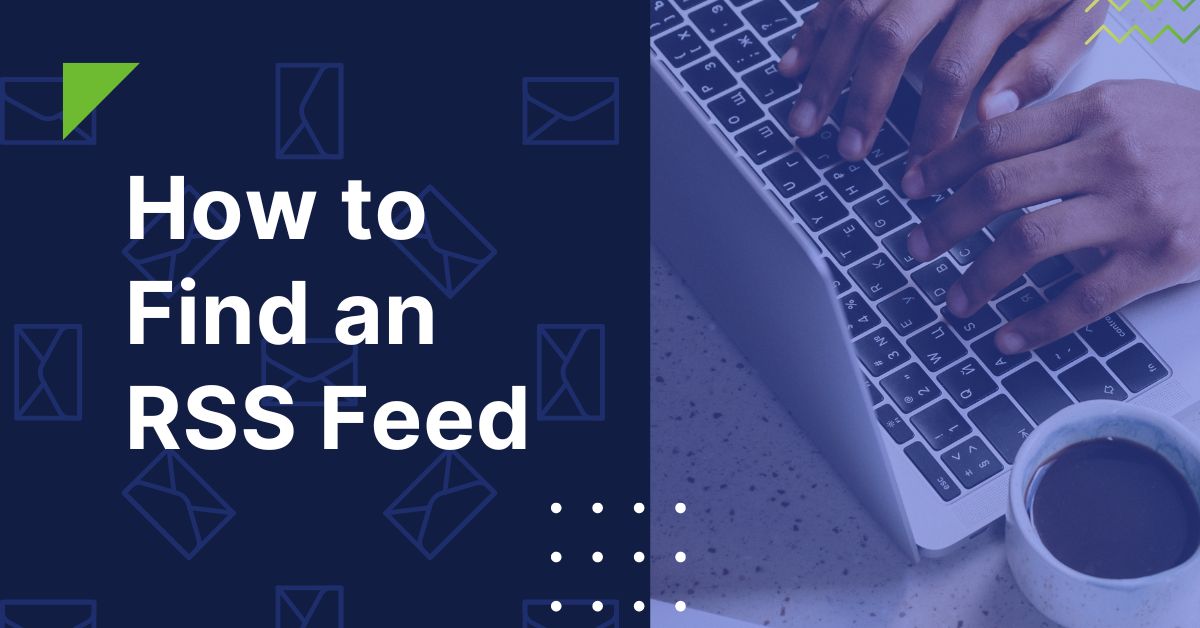
April 23, 2025
Quick Guide: RSS Feed Reader in Ruby in terminal
Have you ever opened many tabs to read your favorite blogs, news, or tech articles? Allow me to explain! We can develop a robust terminal RSS feed reader with a little Ruby.
Ruby makes RSS feed utilities easy to construct, and they are ageless. I will walk you through making a fun Ruby project or learning Ruby. Jump right in!
Setting Up the Environment
Let's get the basics before coding. Check your Ruby installation first. Run ruby -v in your terminal to verify. Visit Ruby's website for installation instructions.
The Ruby gems rss and open-uri will parse RSS feeds and get web data for this project. Install them with:
gem install rss
gem install open-uri
Building the RSS Feed Reader
Step 1: Import Required Libraries
Make a new Ruby file (for example, rss_reader.rb) and add the following libraries to it:
require 'rss'
require 'open-uri'
Step 2: Fetch RSS Feeds
Next, use open-uri to get the RSS feed from a URL. This is an example:
url = 'https://example.com/rss' # Replace with any RSS feed URL
open(url) do |rss|
feed = RSS::Parser.parse(rss)
puts "Feed Title: #{feed.channel.title}"
end
Step 3: Parse and Display Feed Items
The feed reader should read and describe each feed item to be more useful:
open(url) do |rss|
feed = RSS::Parser.parse(rss)
puts "Feed Title: #{feed.channel.title}"
feed.items.each do |item|
puts "Title: #{item.title}"
puts "Link: #{item.link}"
puts "-" * 40
end
end
Enhancements and Features
Even though the simple form works, it could always be enhanced.
One way to improve the reader's usability is to let users enter the RSS feed URL instead of hardcoding it. You can do this by putting in:
puts "Enter the RSS feed URL:"
url = gets.chomp
Another way to make the data easier to read is to format it. Adding line breaks or even gems like colorize can make the output from the terminal look good. As an example:
puts "Title: #{item.title}".colorize(:blue)
Finally, make your tool more robust by handling invalid URLs or network issues gracefully:
begin
open(url) do |rss|
feed = RSS::Parser.parse(rss)
# Processing feed
end
rescue StandardError => e
puts "Error: #{e.message}"
end
Complete Code Example
require 'rss'
require 'open-uri'
puts "Enter the RSS feed URL:"
url = gets.chomp
begin
open(url) do |rss|
feed = RSS::Parser.parse(rss)
puts "Feed Title: #{feed.channel.title}"
feed.items.each do |item|
puts "Title: #{item.title}"
puts "Link: #{item.link}"
puts "-" * 40
end
end
rescue StandardError => e
puts "Error fetching the RSS feed: #{e.message}"
end
Save it as rss_reader.rb and run it with:
ruby rss_reader.rb
Testing and Running the Application
Use well-known RSS feeds like these to test your feed reader:
- BBC News: http://feeds.bbci.co.uk/news/rss.xml
- Ruby Weekly: https://rubyweekly.com/rss
When asked, run the script and paste the URL. The feed information will then show up in your terminal.
Conclusion
You have used Ruby to make an RSS feed reader that works perfectly. This small project not only shows how powerful Ruby is, but it also gives you a useful way to keep up with your favorite topics.
What new things are you going to add? Please tell me how you change your RSS reader. I would love to hear your ideas and thoughts.
257 views