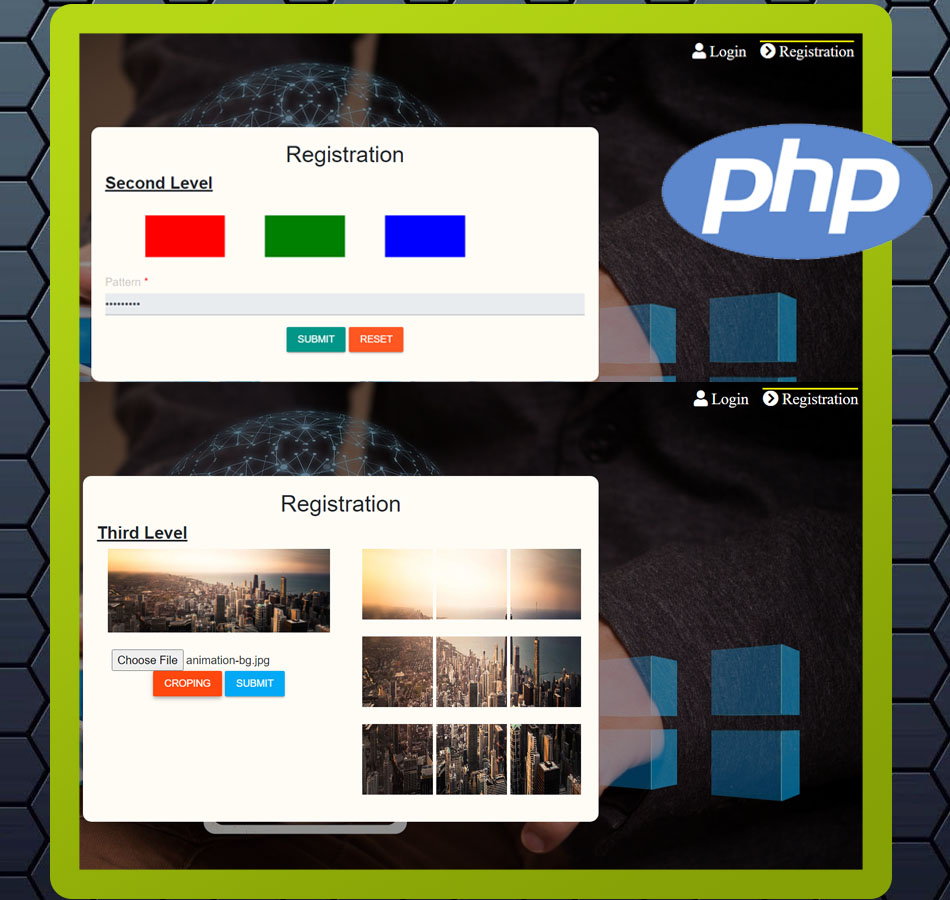
April 22, 2025
Enhancing Web Security with Three-Level Image Password Authentication in PHP
Instead of typing a long password, you can choose images and click on certain areas to connect to your favorite website. Sounds futuristic? Been here! Rising cybersecurity dangers make passwords insufficient. Image-based password authentication is intuitive, engaging, and secure.
This article explains three-level image password authentication and how to build it in PHP. After reading, you will understand the advantages and have a functioning code to test.
Understanding Three-Level Image Password Authentication
Three-level image password authentication? This unique login method uses photos for various verification stages. How it works:
- First Level: Users choose a pre-defined picture grid during registration. This grid is their initial login key.
- Second Level: As their password, users click on certain image areas in a specified order.
- Third Level: Users choose a captcha-style image for final verification.
Why bother? Because hacking becomes tougher. Brute force, phishing, and keylogging work less without text-based passwords.
Step-by-Step Implementation
Let us discuss the process. Create a secure and interactive PHP three-level image password system using these methods.
Setting Up the Environment
Make sure you have these tools ready to go:
- A local server for PHP and MySQL, such as XAMPP or WAMP.
- You should know the basics of PHP, JavaScript, HTML, and CSS.
After setting up the environment, now you can start making the image grids.
Designing the Image Grids
Use the and tags in HTML to make an image grid. Put these images in your database and give each one a unique ID. This grid will show up when the person signs up or logs in so they can make their choices.
Capturing User Input
You must continually capture user grid interactions. JavaScript can detect image clicks. Without reloading the page, AJAX may communicate these selections to your PHP server.
Let's see an example:
<img src="grid.jpg" usemap="#gridmap">
<map name="gridmap">
<area shape="rect" coords="34,44,270,350" href="#" onclick="captureClick(1)">
<area shape="rect" coords="290,44,540,350" href="#" onclick="captureClick(2)">
</map>
<script>
function captureClick(areaId) {
// Send areaId to the server using AJAX
console.log("Area clicked: " + areaId);
}
</script>
Implementing Backend Authentication
Check the server for valid user input. Your database should include the user's grid ID and registration clicks. When a person logs in, compare what they typed with what they saved.
Use secure hashing techniques to store sensitive data:
$selectedGrid = $_POST['grid_id'];
$clickSequence = json_encode($_POST['clicks']);
// Save to database with hashing
$hashedSequence = password_hash($clickSequence, PASSWORD_BCRYPT);
$query = "INSERT INTO users (grid_id, click_sequence) VALUES ('$selectedGrid', '$hashedSequence')";
mysqli_query($conn, $query);
Complete Code Example
Here's a simplified version of the complete code for the three-level image password system:
Database Schema:
CREATE TABLE users (
id INT AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50),
grid_id INT,
click_sequence TEXT
);
PHP Authentication:
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$gridId = $_POST['grid_id'];
$clicks = json_encode($_POST['clicks']);
$query = "SELECT click_sequence FROM users WHERE grid_id = '$gridId'";
$result = mysqli_query($conn, $query);
$row = mysqli_fetch_assoc($result);
if (password_verify($clicks, $row['click_sequence'])) {
echo "Login successful!";
} else {
echo "Authentication failed.";
}
}
JavaScript for Capturing Input: (as shown earlier).
Benefits and Challenges
Benefits
- Enhanced security: brute-force attacks are almost impossible to do.
- User-friendly: It is a fun alternate to text-based passwords.
Challenges
- Difficulty: Needs careful development and planning.
- Learning curve: People who use image-based tools need to get used to them.
Conclusion
Safety cannot be an option in this day and age. Three-level image password security is not just an improvement; it changes the way we keep user data safe. You can make a safe and easy-to-use system that works much better than passwords with PHP and some creativity.
Are you ready to protect your web app better? Do this and let me know how it goes!
238 views