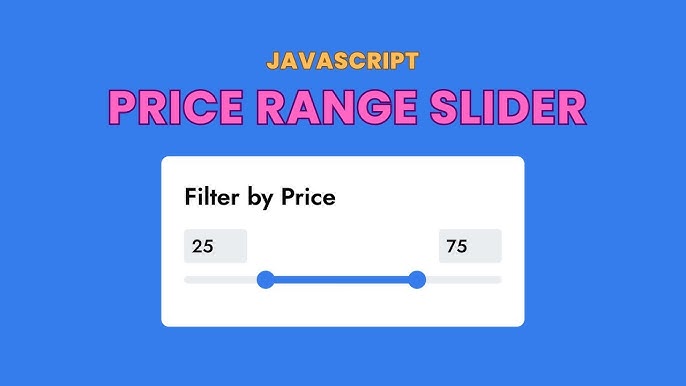
April 21, 2025
Building a Dynamic Price Range Slider with Min-Max Inputs in JavaScript
Imagine going to an online store, looking at the items, and then finding a handy slider that lets you quickly set your budget. It is not necessary to type numbers over and over or restart the page; just one quick slide filters the results. That is how a price range slider works! This guide will teach you how to use JavaScript to make a price range slider that works perfectly with min-max input fields. Trust me, it is not as hard as it sounds, and you will love the end result.
Features of the Dynamic Price Range Slider
Let us talk about why you would want to make a price range slider first.
- Dynamic interaction: The slider reflects input changes without issues.
- Updates in real time: You can connect the slider with API with which the price range will change right away and will make your experience better.
- Customizable design: You can customize its look to match any website theme.
Setting Up the HTML Structure
First, build the layout. The HTML code following is simple and consists of three key parts: The first and second parts are two input fields for the minimum and maximum price, and the third one is a range slider to control the values.
Here's what the HTML looks like:
<div class="price-slider">
<input type="number" id="min-price" placeholder="Min" />
<input type="number" id="max-price" placeholder="Max" />
<input type="range" id="price-slider" min="0" max="1000" step="1" />
</div>
This is the skeleton of your price range slider. Simple, right? Now, let's give it some style!
Adding Styles with CSS
For a UI to be great, it needs to look clean and efficient. You can make the slider look good and work on all devices by using CSS. Here is a short piece of code:
.price-slider input {
margin: 10px;
padding: 5px;
width: 100px;
font-size: 14px;
}
.price-slider #price-slider {
width: 300px;
cursor: pointer;
}
Changing the sizes, colors, and spaces is fine. The slider is not only useful, but it also looks good due to its smooth look.
Implementing Functionality with JavaScript
Linking the Slider and Inputs
The slider should update input fields in real time and vice versa. This JavaScript does that:
const minInput = document.getElementById('min-price');
const maxInput = document.getElementById('max-price');
const slider = document.getElementById('price-slider');
slider.addEventListener('input', () => {
minInput.value = slider.value - 100;
maxInput.value = slider.value;
});
When you slide, the min and max values update automatically.
Input Validation
What if someone inputs an invalid value? Setting the minimum higher than the maximum? We will manage!
minInput.addEventListener('change', () => {
if (parseInt(minInput.value) >= parseInt(maxInput.value)) {
minInput.value = maxInput.value - 100;
}
});
maxInput.addEventListener('change', () => {
if (parseInt(maxInput.value) <= parseInt(minInput.value)) {
maxInput.value = parseInt(minInput.value) + 100;
}
});
This ensures logical price ranges and prevents errors.
Dynamic Updates
Want the slider to adjust dynamically when inputs change? Here's the code:
minInput.addEventListener('input', () => {
slider.min = minInput.value;
});
maxInput.addEventListener('input', () => {
slider.max = maxInput.value;
});
Testing and Debugging
It is time to test the slider once it works!
- Get Chrome, Firefox, and Safari to see how it works on those browsers.
- Make sure it works on mobile devices by testing it there.
- To fix any problems, like mouse lag or actions that do not work, use tools like Chrome DevTools.
Tip: Look out for ranges that overlap; this is a typical problem that can be fixed quickly by changing the logic behind the validation.
Enhancements and Customizations
Do you want to improve your slider? Here are some ideas:
- To make things clearer, put a currency sign next to the entries.
- Get real-time price info from APIs and show it next to the bar.
- Make the slider move so that it is easier to use. It is amazing how much CSS can do!
Conclusion
That is it! An HTML, CSS, and JavaScript-based price range slider that works perfectly and changes based on the price range. This not only makes your website easier to use, but it also makes it look a little more classy.
Are you ready to try it? Share what you have made or any changes you have made. I would love to see how you have changed your slider. Let's keep making cool things!
58 views