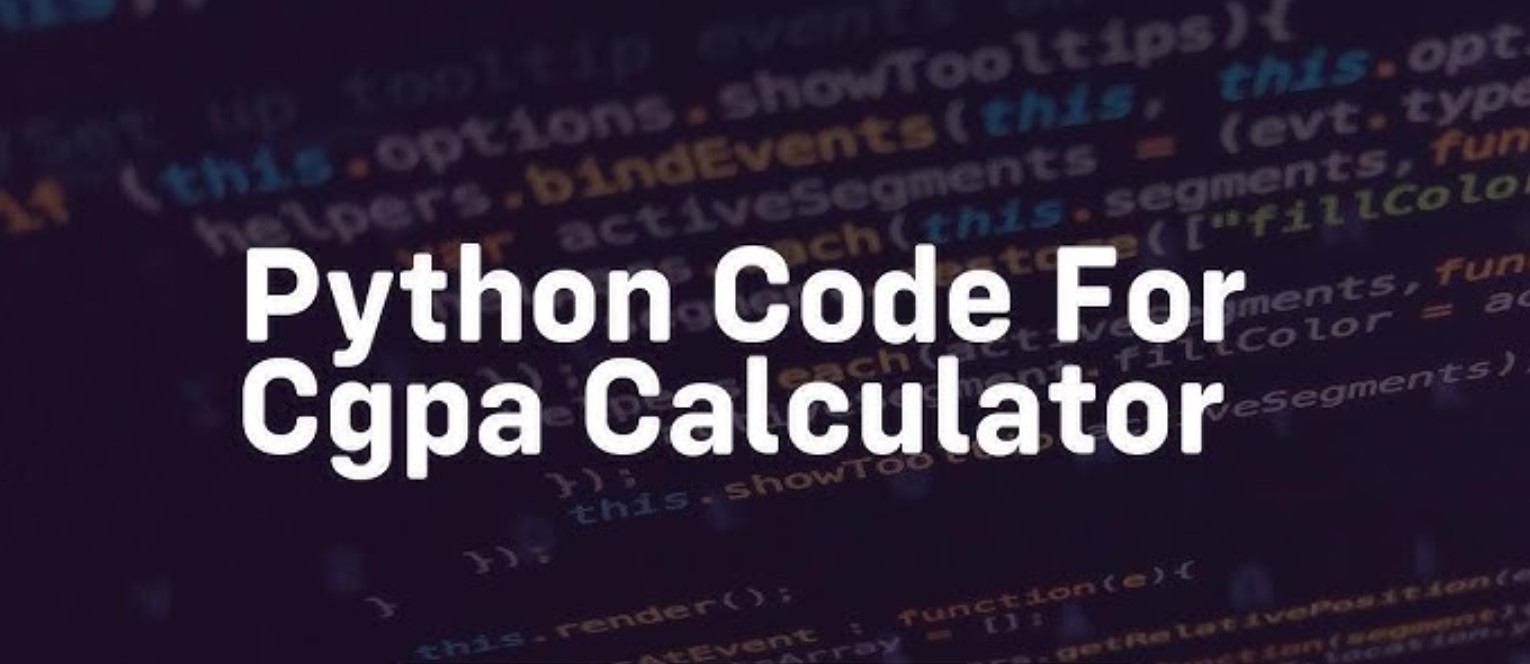
April 18, 2025
Building a Simple CGPA Calculator in C++ With Complete Code
Have you ever had trouble calculating your CGPA manually, especially when you had a lot of grades and credit hours to look at? I know what you mean; it is not fun! Due to this, I chose to make an easy CGPA tool in C++ to help me out. I will show you how to make your own calculator step by step in this post. You will have a tool that works and saves you time and work by the end. Let us jump right in!
Prerequisites for Building the CGPA Calculator
You will need to know the basics of C++ writing before we start. If you know how to use variables, loops, and arrays, you should be fine even if you are not an expert. You will also need a code tool such as Code::Blocks or Visual Studio Code to write and run your own code.
Understanding the CGPA Formula
Let's learn about the calculations behind the CGPA before we start writing code. CGPA stands for "cumulative grade point average." It tells you how well you did in studies generally.
Here's the formula:
CGPA = (Total Grade Points Earned) / (Total Credit Hours Attempted)
In this case, let's say you took three classes and got the following grades and credit hours:
Course 1: Grade = 4.0, Credit Hours = 3
Course 2: Grade = 3.5, Credit Hours = 4
Course 3: Grade = 3.0, Credit Hours = 2
The calculation would be:
CGPA = [(4.0 Ãâ 3) + (3.5 Ãâ 4) + (3.0 Ãâ 2)] / (3 + 4 + 2)
CGPA = 3.56
Structuring the Program
Now that we know the formula, let's make a strategy for the program. This is how it will work:
- Input: The user types in the amount of courses, grades, and credit hours.
- Processing: The program then multiplies the grades by credit hours, add them up, and divides that number by the total number of credit hours.
- Output: Finally the calculated CGPA will show.
Key ideas we will use include loops for math and arrays for calculating CGPA of multiple subjects at once.
Complete C++ Code for the CGPA Calculator
Here's the complete C++ code for the calculator:
#include <iostream>
using namespace std;
int main() {
int numSubjects;
cout << "Enter the number of subjects: ";
cin >> numSubjects;
float grades[numSubjects], creditHours[numSubjects], totalGradePoints = 0, totalCredits = 0;
for (int i = 0; i < numSubjects; i++) {
cout << "Enter grade for subject " << i + 1 << ": ";
cin >> grades[i];
cout << "Enter credit hours for subject " << i + 1 << ": ";
cin >> creditHours[i];
totalGradePoints += grades[i] * creditHours[i];
totalCredits += creditHours[i];
}
float cgpa = totalGradePoints / totalCredits;
cout << "Your CGPA is: " << cgpa << endl;
return 0;
}
Explanation
- Dynamic Input: To scale the program, we make use of arrays to record grades and credit hours for each subject.
- Calculation: A loop multiplies grades by credit hours and sums them.
- Error Handling: Verify non-negative grades and credit hours before calculating.
Testing the CGPA Calculator
Here's an example:
Input:
Number of subjects: 3
Grades: 4.0, 3.5, 3.0
Credit Hours: 3, 4, 2
Output:
Your CGPA is: 3.56
Conclusion and Next Steps
Good job on making your own CGPA calculator. It is a big step toward simplifying boring academic tasks. The code is simple but powerful, and you can make it even better by adding things like input checks or even a tool to change from GPA to CGPA.
Feel free to give it a try. You will be glad you did this the next time you need to figure out your CGPA.
290 views