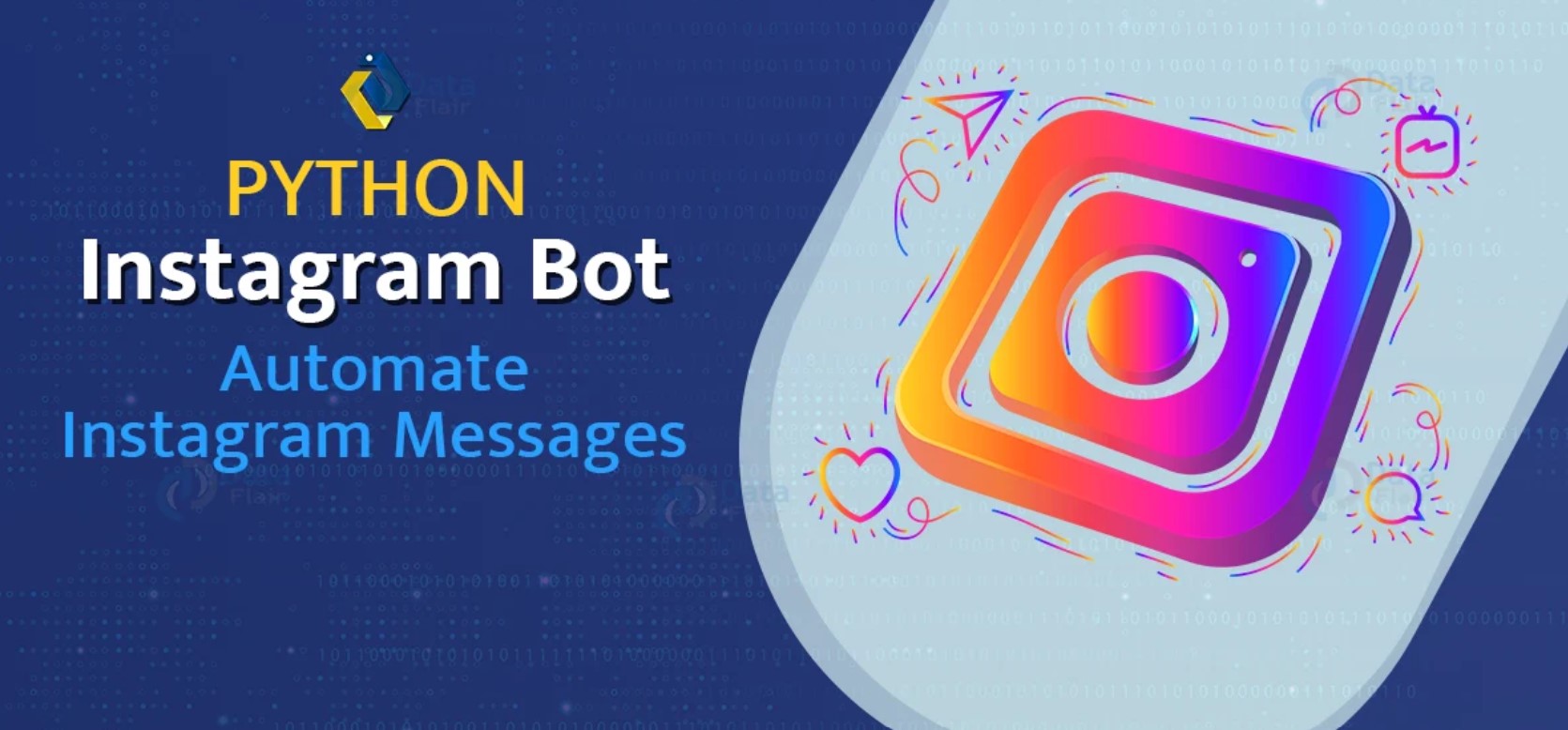
April 17, 2025
Automate Your Instagram DMs with Python: A Step-by-Step Guide
Managing your Instagram DMs might seem endless. Right? Manually processing messages can be challenging for influencers, company owners, and anyone with a busy social media presence.
Is it possible to automate this procedure and simplify your life? Well yes. Python can automate Instagram DMs, saving hours each week. This post will help you put up a basic yet powerful automation script. Finally, you will have an assistant that works for you!
Tools and Libraries Needed
Before digging into the code, get the basics:
- Selenium: An advanced web automation tool.
- Credentials for Instagram: Login and password (ideally for a test account).
- Python: Download the latest version if necessary.
- ChromeDriver: Automates Chrome tasks using Selenium.
A virtual environment for organizing dependencies is optional but beneficial. Remember to use automation carefully to follow Instagram's TOS!
Setting Up the Environment
- Install Python: Get the most latest version of Python from its official website if you have not already.
- Install Selenium: Open a shell or command prompt and run the following:
pip install selenium
- Set up ChromeDriver: Download the version of ChromeDriver that works with your browser. Therefore, add it to your system's PATH so that Selenium can use it.
- Project Folder: Make a new folder to keep your project in order.
Tip: To avoid putting your real Instagram account at risk while setting up your new one, use a fake one for trying.
Step-by-Step Code Implementation
Step 1: Import the Required Libraries
Start by importing the essential libraries:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
import time
Step 2: Log in to Instagram
The first step of automation is logging into your Instagram account.
# Initialize ChromeDriver
driver = webdriver.Chrome()
driver.get("https://www.instagram.com/")
# Wait for the page to load
time.sleep(5)
# Enter username and password
username = driver.find_element(By.NAME, "username")
password = driver.find_element(By.NAME, "password")
username.send_keys("your_username")
password.send_keys("your_password")
password.send_keys(Keys.RETURN)
# Wait for login to complete
time.sleep(5)
Step 3: Navigate to the DM Section
After logging in, head to the Direct Messages section.
driver.get("https://www.instagram.com/direct/inbox/")
time.sleep(5)
Step 4: Select Recipients and Send Messages
Now, let's send a message.
# Search for a user
search_box = driver.find_element(By.XPATH, '//input[@placeholder="Search..."]')
search_box.send_keys("recipient_username")
time.sleep(3)
# Select the user from results
user = driver.find_element(By.XPATH, '//div[contains(text(), "recipient_username")]')
user.click()
# Type and send the message
message_box = driver.find_element(By.XPATH, '//textarea[@placeholder="Message..."]')
message_box.send_keys("Hello! This is an automated message.")
message_box.send_keys(Keys.RETURN)
# Wait for the message to send
time.sleep(3)
Step 5: Handle Errors and Exceptions
To make your script more efficient you can add error handling:
try:
# Your automation steps here
except Exception as e:
print(f"An error occurred: {e}")
finally:
driver.quit()
Tips for Effective Automation
- Avoid spamming: You should personalize your texts and try to not send them too often.
- Random delays: Use time.sleep() with random intervals to mimic human behavior.
- Recipient tracking: Keep track of the people you have already sent messages to.
Testing and Debugging
Test your script with a small group of people to find and fix any bugs. XPath keywords that do not work right or browser support are common problems. It is very helpful to have debugging tools like Selenium's live debugger.
Conclusion
There you have it, a full, step-by-step guide on how to use Python to automate your Instagram direct messages! Automation does more than just save you time; it also frees you up to work on bigger things, like growing your business or building relationships.
You can change the script to make it your own. You can even use it for bulk messages as long as you do it in a right way. Are you ready to try it?
341 views