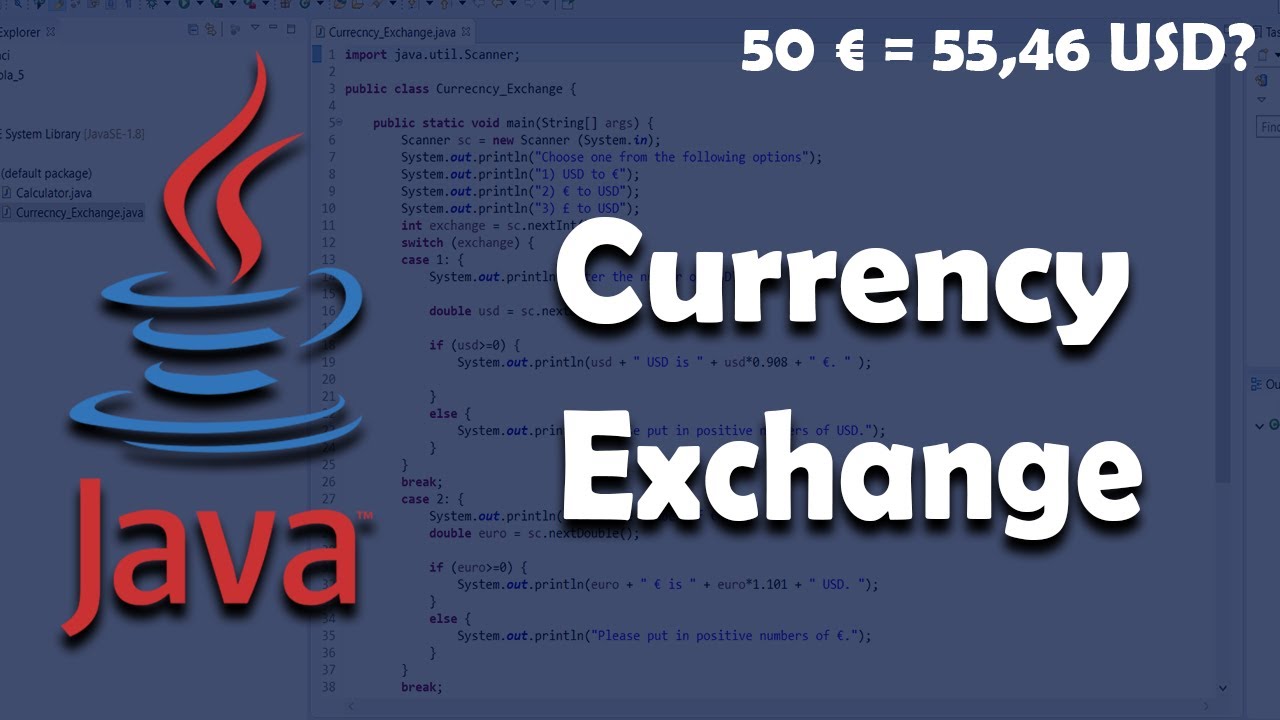
April 16, 2025
How to Build a Robust Currency Converter Application in Java
Imagine converting currencies with a few clicks on the spot. Traveling overseas, shopping online, or just wondering about conversion rates all benefit from a currency converter. I will teach you how to develop a basic but robust Java currency converter application today. To make the application functional and simple to use, we will learn how to get real-time exchange rates using APIs and develop an intuitive user interface. Let's jump in!
Setting Up the Development Environment
Let's check everything before coding. Install the Java Development Kit (JDK) first. Oracle's website offers a download and installation guide. Code completion and debugging capabilities in an IDE like Eclipse or IntelliJ IDEA make coding simpler.
Once you have your JDK and IDE, we will need libraries for HTTP requests and JSON parsing. An API needs these for real-time currency exchange rates. HttpURLConnection will get the data and org.json will parse the return. After setting up, you can start code!
Getting Real-Time Exchange Rates
Let's discuss how to get exchange rates now. We will use a public API like ExchangeRate-API or CurrencyLayer, which give us the most up-to-date exchange rates for many different currencies.
This simple code utilizes HttpURLConnection to get the latest exchange rates in JSON format:
import java.net.*;
import java.io.*;
public class CurrencyConverter {
public static void main(String[] args) throws Exception {
String url = "https://api.exchangerate-api.com/v4/latest/USD";
URL obj = new URL(url);
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
}
}
This code performs a GET call to the API URL to display the current currency rates. We must sort this data to determine the exchange rates, which is the next step.
Designing the Currency Conversion Logic
After getting the JSON data, we need to change it to a format that we can use. The API returns an object with currency maps to their exchange rates relative to the base currency (USD). Let's write a method that can do conversion between two different currencies:
public double convertCurrency(double amount, String fromCurrency, String toCurrency, JSONObject rates) {
double fromRate = rates.getDouble(fromCurrency);
double toRate = rates.getDouble(toCurrency);
return (amount / fromRate) * toRate;
}
You can call this method with the amount you want to change, the currency names (like "USD", "EUR", etc.), and the rates JSON object. The code then takes the amount and splits it by the fromCurrency's exchange rate. It then doubles that number by the toCurrency's exchange rate to get the changed value.
Building the User Interface
Not only does a great app need to work well, but it also needs to be easy for people to use. The next step is to make our currency calculator easy to use by adding a graphical user interface (GUI). We will use Java's JFrame to make a simple interface with a button that will convert the amount and text boxes for choosing the currency.
Here;s a simple GUI setup:
import javax.swing.*;
import java.awt.event.*;
public class CurrencyConverterApp {
public static void main(String[] args) {
JFrame frame = new JFrame("Currency Converter");
JTextField amountField = new JTextField();
JButton convertButton = new JButton("Convert");
JLabel resultLabel = new JLabel("Converted Amount:");
convertButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
// Conversion logic here
}
});
frame.add(amountField);
frame.add(convertButton);
frame.add(resultLabel);
frame.setSize(300, 200);
frame.setLayout(new FlowLayout());
frame.setVisible(true);
}
}
This code makes a window with a text box where you can enter the amount, a button that will convert it, and a label that will show the result. You can easily make this better by giving the user more options for currencies and making the result look nice by adding drop-down lists.
Conclusion
Making a Java currency converter is a fun and useful job. You can make a useful tool that changes currencies with just a few clicks by adding an API for real-time data. Setting up the working environment, building the core features, and making a simple GUI are all things we have talked about. Now it is your turn to make the app better and try out the more complicated features!
457 views