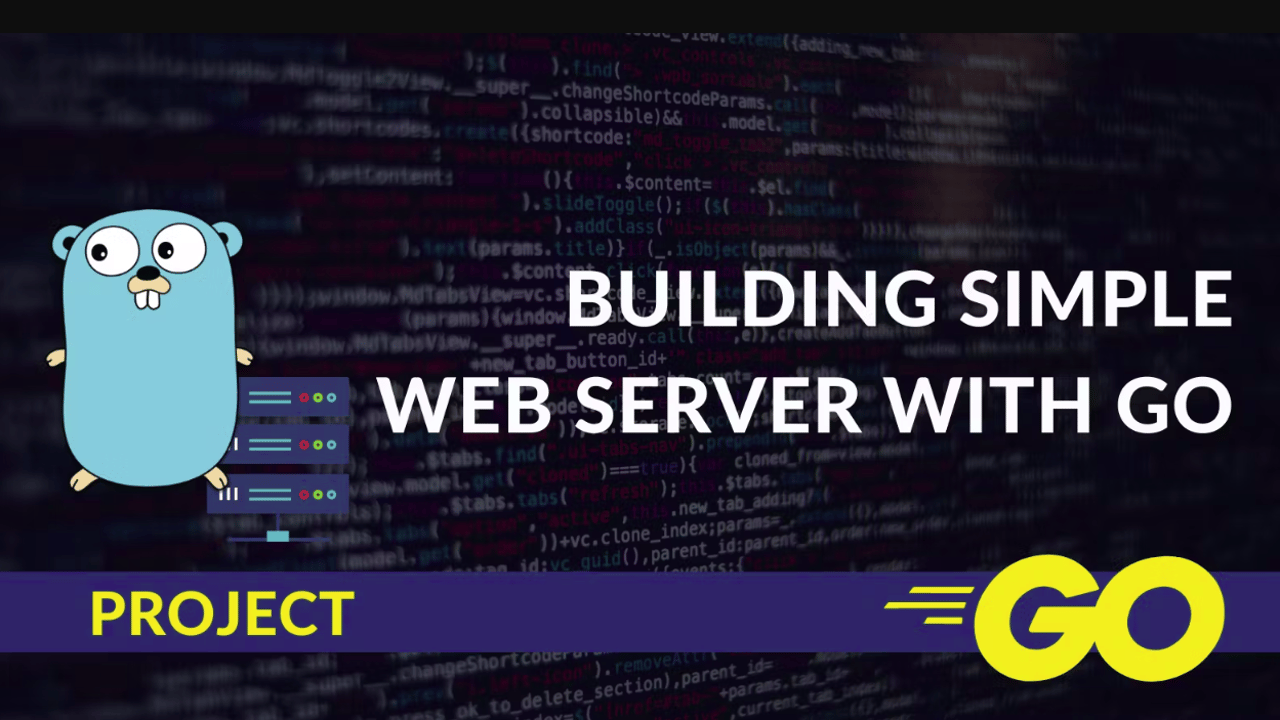
April 15, 2025
Building a Simple Web Server in Go: A Beginner's Guide
Have you ever thought about how websites really work? When I first started to be interested in web development, I liked how simple and quick Go (also called Golang) was. Go is a great language for making web servers because it is fast and can handle multiple tasks at once.
I will show you how to make your first Go web server in this blog. Not to worry if you are new to this; I will keep it easy for you and show you how to do each step. You will have a fully working web server that you can use for your own projects by the end.
Prerequisites and Setup
Make sure you know some simple things before we start. What you will need:
- Get Go installed on your system (you can get it from golang.org).
- You will need a code tool like Visual Studio Code or GoLand.
- You should know the basics of Go syntax, but I will help you as much as I can.
Make a new project directory to start. Start up your system, go to your workspace, and make a folder:
mkdir simple-web-server
cd simple-web-server
This will be our playground for building the web server.
Understanding Go's net/http Package
The strong net/http package in Go is at the heart of our web server. It handles both HTTP requests and replies. This package has everything you need to build web servers without having to rely on other packages.
These are the two main tasks we will do:
- https.HandleFunc: Connects a URL path to a handling code.
- http.ListenAndServe: This method starts the server and watches for requests on a certain port.
But why Go? It can handle many calls at once, even when resources are limited, because of its concurrency approach. Plus, Go's simple code makes things easy to understand, making it great for both new and experienced coders.
Building the Web Server
Let us move on! For the new file named main.go, open your preferred code editor. To make a simple Go web server, follow these steps:
1. Write a Handler Function
When HTTP requests come in, a handler function manages them and sends replies. First, let's look at a simple code that says "Hello, World!"
package main
import (
"fmt"
"net/http"
)
func helloHandler(w http.ResponseWriter, r *http.Request) {
fmt.Fprintln(w, "Hello, World!")
}
2. Set Up Routing
We use http.HandleFunc to link a specific URL path (e.g., "/") to our handler function.
3. Start the Server
Finally, call http.ListenAndServe to run the server:
func main() {
http.HandleFunc("/", helloHandler)
fmt.Println("Starting server on :8080...")
if err := http.ListenAndServe(":8080", nil); err != nil {
fmt.Println("Error starting server:", err)
}
}
Test Your Server
Use go run main.go to start the server. Now, launch a browser and visit http://localhost:8080. The message "Hello, World!" should show up.
Enhancements and Next Steps
There are many ways to improve your server once it is up and running. From the start, you can make a more dynamic application by adding more routes, each with its own set of responses. Adding middleware is a great next step as it makes jobs like logging, identification, and error handling easier to do.
When you are ready to move your server to the cloud, systems like AWS, Google Cloud, or Heroku make it easy to make your server available to everyone. Go frameworks like Gin and Echo can give you even more power and freedom. They make routing and middleware handling easier, which lets you make more complicated and scalable apps.
Conclusion
Go lets you do a lot of useful things with the web, and building a simple web server is the initial step. Going with Go is a good way to build fast and scalable web apps because it is efficient and easy to use. Try new things and learn about more advanced Go features. Soon you will be ready to work on bigger and more complicated projects and systems!
95 views