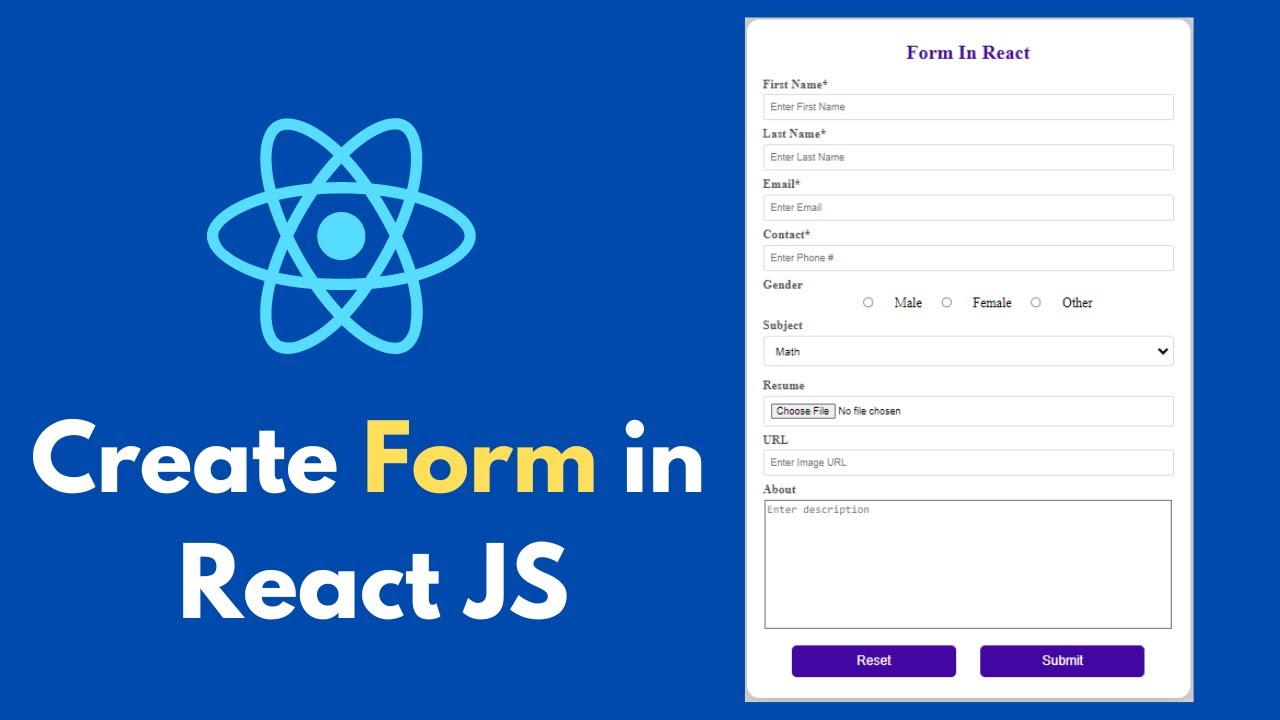
April 14, 2025
How to Create a Submission Form Using React
Forms are the most important part of any web app. Forms connect users to the features of your app, whether you are getting comments, taking orders, or setting up user accounts. It looked hard to me at first to build forms in React, but trust me, it is easy once you get the hang of it! This blog post will show you how to use React to make a submission form. Let's keep this easy, quick, and fun!
Setting Up the React Environment
Let's check that everything is okay before we start writing code. Put in Node.js and a package manager like npm if you have not already. Next, use the following code to start a React project:
npx create-react-app my-form-app
cd my-form-app
npm start
This makes a React app template that works perfectly. To continue, you will need a coding editor (I suggest Visual Studio coding).
Building the Basic Form Component
This is where the magic starts. To make the UI in React more modular, we use components. First, let's look at a simple form component.
Put React into your project and create a working component first:
import React, { useState } from "react";
function SubmissionForm() {
const [formData, setFormData] = useState({ name: "", email: "" });
const handleChange = (e) => {
setFormData({ ...formData, [e.target.name]: e.target.value });
};
return (
<form>
<label>
Name:
<input
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
</label>
<br />
<label>
Email:
<input
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
</label>
<br />
<button type="submit">Submit</button>
</form>
);
}
export default SubmissionForm;
Here, one input field is for the user's name, and the other is for their email address. Have you seen the useState hook? It tracks numbers as users input and changes the form's state with each click.
Adding Form Validation
No one likes inputs that are not valid, right? Before we send the form, let's make sure that all of the fields are filled out correctly. For example, we can check to see if the email has a "@" symbol and show an error message if it doesn't.
Here's how you can add simple validation logic:
const handleValidation = () => {
if (!formData.email.includes("@")) {
alert("Please enter a valid email address.");
return false;
}
return true;
};
Before handling the form submission, you can call this method to make sure everything is okay.
Handling Form Submission
In React, all you have to do to send a form is add a onSubmit function. Instead of letting the page restart by default, let's log the form data:
const handleSubmit = (e) => {
e.preventDefault();
if (handleValidation()) {
console.log("Form submitted:", formData);
}
};
Now, update your form tag to include the handler:
<form onSubmit={handleSubmit}>
Try it out! Fill out the form, then click "Submit" in your browser's console. Immediately, you will see the form data inserted.
Styling the Form
I do not know about you, but I hate forms that look too simple and basic. Let's give it some style to make it user-friendly to use.
Create a new CSS file (e.g., form.css) and add some basic styles:
form {
max-width: 400px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
input {
width: 100%;
margin-bottom: 10px;
padding: 8px;
border: 1px solid #ccc;
border-radius: 3px;
}
Import this CSS file into your form component using:
import "./form.css";
Advanced Features
Do you want to make your form look more attractive? No worries, for this you can add libraries like Formik and Yup. Using minimal code, these packages simplify form state management and verification. Using React you can also support file uploads and multi-step form wizards.
Conclusion
There you have it: a wonderful React submission form. We started from scratch and made a simple, styled, and checked form that was easy to use. Now it is your turn to customize this form to fit the goals of your project. You have the building blocks to make a user registration form or a feedback system work.
111 views