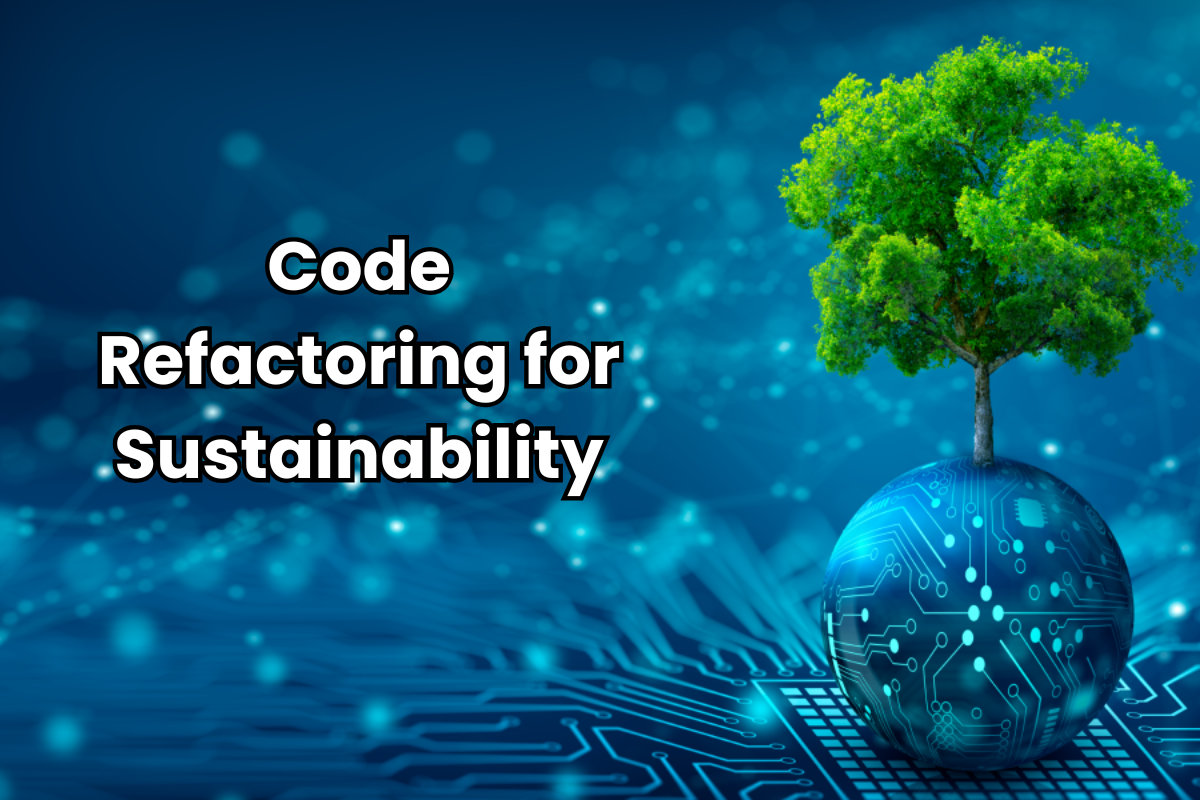
March 20, 2025
Code Refactoring for Sustainability: Writing Less Resource-Intensive Code
Ever glared at old code and thought, "What was I thinking?" I promise we have all been there. The codebase typically becomes a collection of instant fixes and old techniques as projects develop. Sustainable coding keeps code clean, efficient, and maintained for years. Let me teach you how to refactor your JavaScript projects for long-term sustainability.
Why Sustainable Code Matters
Sustainable coding is about creating something that works now and tomorrow. Inefficient or poorly organized code wastes resources, takes longer to debug, and is hard to scale. Inefficiency increases technological debt, wasting time and resources.
Sustainable techniques make projects healthy and future-proof, whether you operate alone or with others. Better practices today will save many headaches later.
Identifying Areas for Refactoring in JavaScript
We must find code violators before refactoring it. Let's see some red flags:
- Duplicate Code: Repetitive logic between functions.
- Monolithic Functions: Excessive code with several tasks.
- Inefficient File Organization: Lack of obvious structure or grouping of similar functions.
- Poor Loops: Nested or unoptimized iterations.
ESLint helps identify these problems. It checks your code for issues and suggests fixes. Try it now if you haven't.
Key Refactoring Techniques
1. Simplifying Functions and Reducing Nesting
Long, awkward functions are terrible. Make them smaller and more focused. This makes the code easier to read and fix.
Example Before Refactoring:
function processOrder(order) {
if (order && order.items) {
for (let i = 0; i < order.items.length; i++) {
if (order.items[i].stock > 0) {
console.log("Processing item:", order.items[i].name);
}
}
}
}
After Refactoring:
function isItemInStock(item) {
return item.stock > 0;
}
function processOrder(order) {
if (!order || !order.items) return;
order.items.filter(isItemInStock).forEach(item => console.log("Processing item:", item.name));
}
2. Eliminating Redundancy
Combine repetitious code into reusable functions or components.
Example: Refactor similar event listeners into one dynamic function:
["click", "mouseenter", "mouseleave"].forEach(event => {
element.addEventListener(event, handleEvent);
});
3. Optimizing Loops
Most of the time, traditional for loops are too hard to understand. Map, filter, and trim are some modern methods.
Example:
// Before
let total = 0;
for (let i = 0; i < numbers.length; i++) {
total += numbers[i];
}
// After
const total = numbers.reduce((sum, num) => sum + num, 0);
4. Using Modern JavaScript Features
Update your code to use ES6+ arrow functions, destructuring, and let/const.
Example:
// Before
var name = user.name;
var age = user.age;
// After
const { name, age } = user;
Measuring and Ensuring Long-Term Maintainability
Refactoring is just the beginning. To keep your code up to date, think about these tips:
- Automated Testing: Using unit tests to find mistakes early and keep functionality after rewriting is important.
- Documentation: Write down changes you made to make the code's goal clearer so that others can use it in the future.
- Monitoring Tools: Use monitoring tools like Lighthouse or WebPageTest to track performance.
Conclusion
Rewriting your code with sustainability in mind improves it in the short term, but it also positions you for success in the long run. By making methods easier to understand, reducing repetition, and using new JavaScript features, you can create a script that is clean, efficient, and easy to manage.
Why not explore an old project today? Start small, refactor a few lines, and watch it change. You will thank yourself later.
270 views