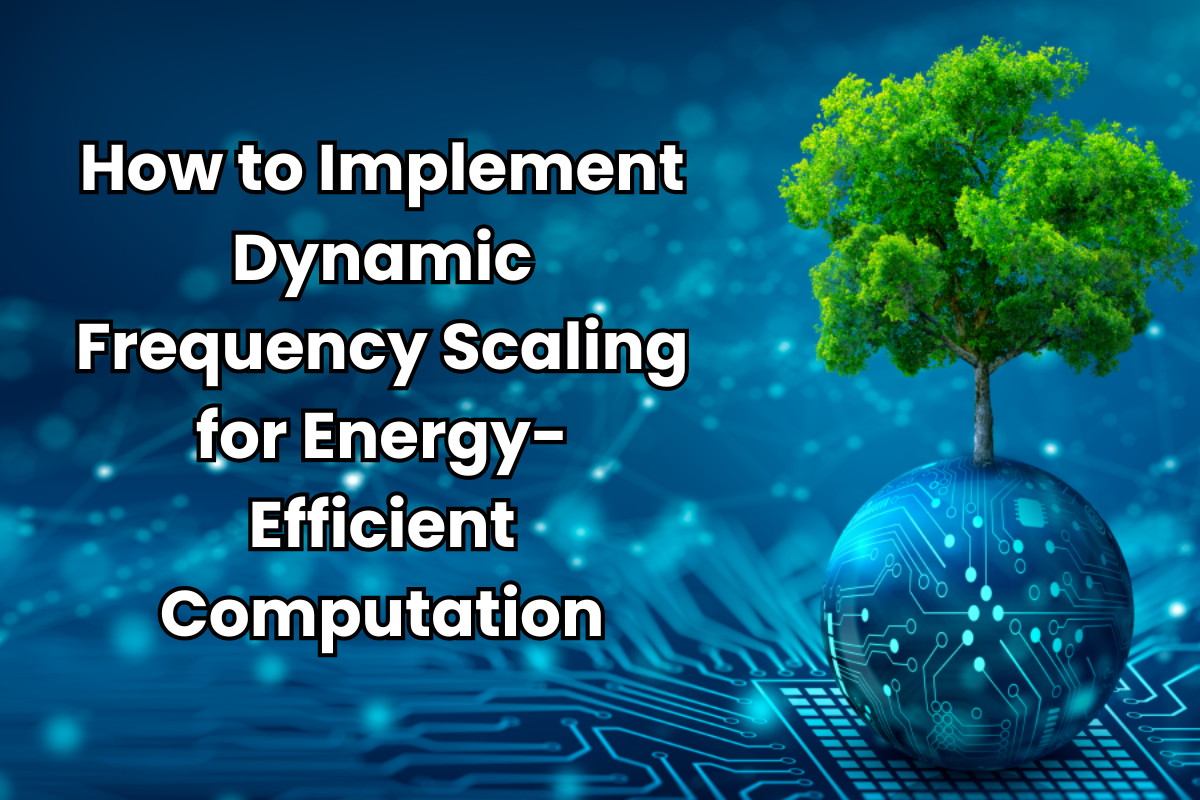
March 19, 2025
How to Implement Dynamic Frequency Scaling for Energy-Efficient Computation
Have you considered how computers and cellphones keep cool, speedy, and battery-friendly? Dynamic Frequency Scaling (DFS), changing CPU speed dynamically is crucial. DFS has benefits, and this blog post will teach you how to create it in C++. Let us find out how to optimize the CPU to work better without slowing it down.
What is Dynamic Frequency Scaling?
Dynamic Frequency Scaling (DFS) lets you change your CPU's frequency and speed based on how much work it needs to do. CPUs slow down to conserve power while not in use. It accelerates when tasks need more energy. Mobile devices and energy-efficient systems require this technology to save power and respond.
How DFS Works?
DFS adjusts CPU frequency based on workload. Low-demand times lower CPU frequency to minimize energy and heat. In contrast, intense jobs need higher frequency to guarantee smooth execution. These changes are controlled by governors, like "performance" (set top speed) or "powersave" (lowest frequency). Understanding how these systems work helps you tune your system for performance and power.
Implementing DFS in C++
Programmatic control of DFS is wonderful. This section shows how to use Dynamic Frequency Scaling in C++ to modify CPU frequency on-the-fly. This Linux cpufreq subsystem sample sets a target frequency.
Here's a simple example:
#include <iostream>
#include <fstream>
void set_cpu_frequency(int frequency) {
std::ofstream cpu_freq_file("/sys/devices/system/cpu/cpu0/cpufreq/scaling_setspeed");
if (cpu_freq_file.is_open()) {
cpu_freq_file << frequency;
cpu_freq_file.close();
std::cout << "CPU frequency set to " << frequency / 1000 << " MHz." << std::endl;
}
}
int main() {
int target_frequency = 1800000; // 1.8 GHz
set_cpu_frequency(target_frequency);
return 0;
}
In this code, we open scaling_setspeed and write the desired frequency in kHz immediately. This simple method dynamically adjusts CPU speed. This is a good way to directly manage how fast the CPU works and save power when needed.
Best Practices and Considerations
Even though DFS is a useful tool, there are some things you should always do. It is best to pick the frequency scaling method carefully and set the right times because making changes too often can add extra work. Keep in mind that hardware and operating system settings may limit CPU speed changes. Find a balance between work performance and power utilization to be efficient. Use lesser rates for easier jobs and ramp up when required.
Conclusion
Dynamic Frequency Scaling is a simple but useful way to control how fast and how much power your CPU uses. Optimizing performance and power consumption is as easy as adjusting your CPU speed using C++ code. Experimenting with DFS may improve the performance of your applications, devices, or systems. Are you ready to play DFS? Find out what works by further experimenting with the code!
263 views