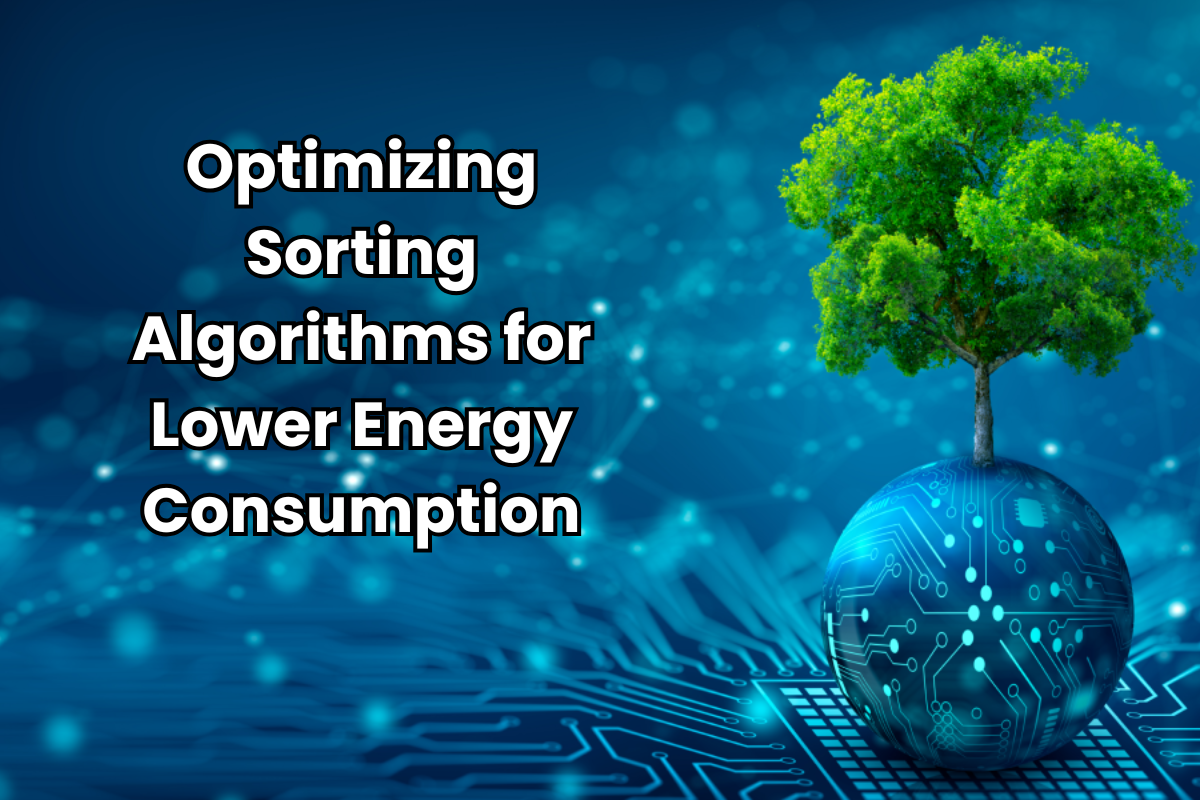
March 17, 2025
Optimizing Sorting Algorithms for Lower Energy Consumption
Do you know that your data-sorting algorithms can be wasting energy? In an era where energy efficiency is a primary technological objective, even small code optimizations can make a difference. Software developers can improve sorting algorithms for speed and CPU efficiency. I'll discuss how to write code for energy-efficient sorting algorithms that minimize CPU load and make software more sustainable below in this blog post.
What Makes a Sorting Algorithm Energy-Efficient?
CPU power and memory use determine sorting algorithm energy efficiency. Time complexity and memory management impact an algorithm's computational steps (or cycles), which affect CPU usage. Sorting algorithms with O(n^2) time complexity are CPU-intensive and use more power than those with O(n log n) complexity.
A simple method like Bubble Sort (O(n^2)) uses more CPU power due to the many comparisons and swaps needed for huge datasets. However, techniques like Merge Sort (O(n log n)) decrease operations, making them more efficient even with enormous datasets.
Choose the Right Sorting Algorithm
The sorting algorithm considers dataset size and real-time sorting. Let's analyze the energy-efficiency of below sorting algorithms:
1. Merge Sort
Merge Sort is a fast sorting algorithm with O(n log n) complexity. Its steady speed is good for large datasets. It takes more memory for the left and right sub-arrays, but it uses less energy since it reduces comparisons and operations.
2. Quick Sort
Another efficient algorithm with an average complexity of O(n log n). However, poor pivot selection (e.g., in a virtually sorted array) might result in O(n^2) performance and increased CPU use. Due to in-place sorting, Quick Sort is usually quicker.
3. Insertion Sort and Selection Sort
These two algorithms take O(n^2) time, which means they are not good for working with big datasets. However, its simplicity can make up for their scalability issues for small datasets. These algorithms use greater CPU power as input size increases.
Merge Sort is ideal for big datasets, although Insertion Sort can be more energy-efficient for smaller datasets or with memory restrictions.
Writing an Energy-Efficient Sorting Algorithm
Now that we know which algorithms are energy-efficient, let's write one with low CPU utilization. Merge Sort works well in many situations.
Avoid repetitive comparisons and swaps while creating an energy-efficient sorting algorithm. In-place sorting algorithms that use less memory save energy.
Optimization of Merge Sort in Python:
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left_half = arr[:mid]
right_half = arr[mid:]
merge_sort(left_half)
merge_sort(right_half)
i = j = k = 0
while i < len(left_half) and j < len(right_half):
if left_half[i] < right_half[j]:
arr[k] = left_half[i]
i += 1
else:
arr[k] = right_half[j]
j += 1
k += 1
while i < len(left_half):
arr[k] = left_half[i]
i += 1
k += 1
while j < len(right_half):
arr[k] = right_half[j]
j += 1
k += 1
return arr
Recursively sorting and merging subarrays makes this approach efficient. The result is fewer operations than standard methods like Bubble Sort.
Performance and Optimization Considerations
An algorithm can have a theoretically superior time complexity, but memory utilization, processor cache efficiency, and input data properties might affect performance. Big-O analysis can estimate the algorithm's worst-case performance, but profiling and actual testing are essential for CPU utilization inefficiencies.
Another consideration is memory efficiency. Optimizing your algorithm to employ in-place sorting (like Quick Sort) or decrease memory allocations (like Merge Sort) can save energy. Python's cProfile can detect CPU-intensive programs.
Conclusion
As greener computing options become more popular, energy efficiency is as important as speed when choosing a sorting algorithm. Developers can choose or improve sorting algorithms to save energy by knowing how time complexity and memory utilization affect CPU cycles. Using Merge Sort for bigger datasets or simpler techniques for smaller ones adds up to total power consumption savings.
You can optimize code performance and contribute to a sustainable technology environment by profiling and evaluating sorting algorithm energy usage.
85 views