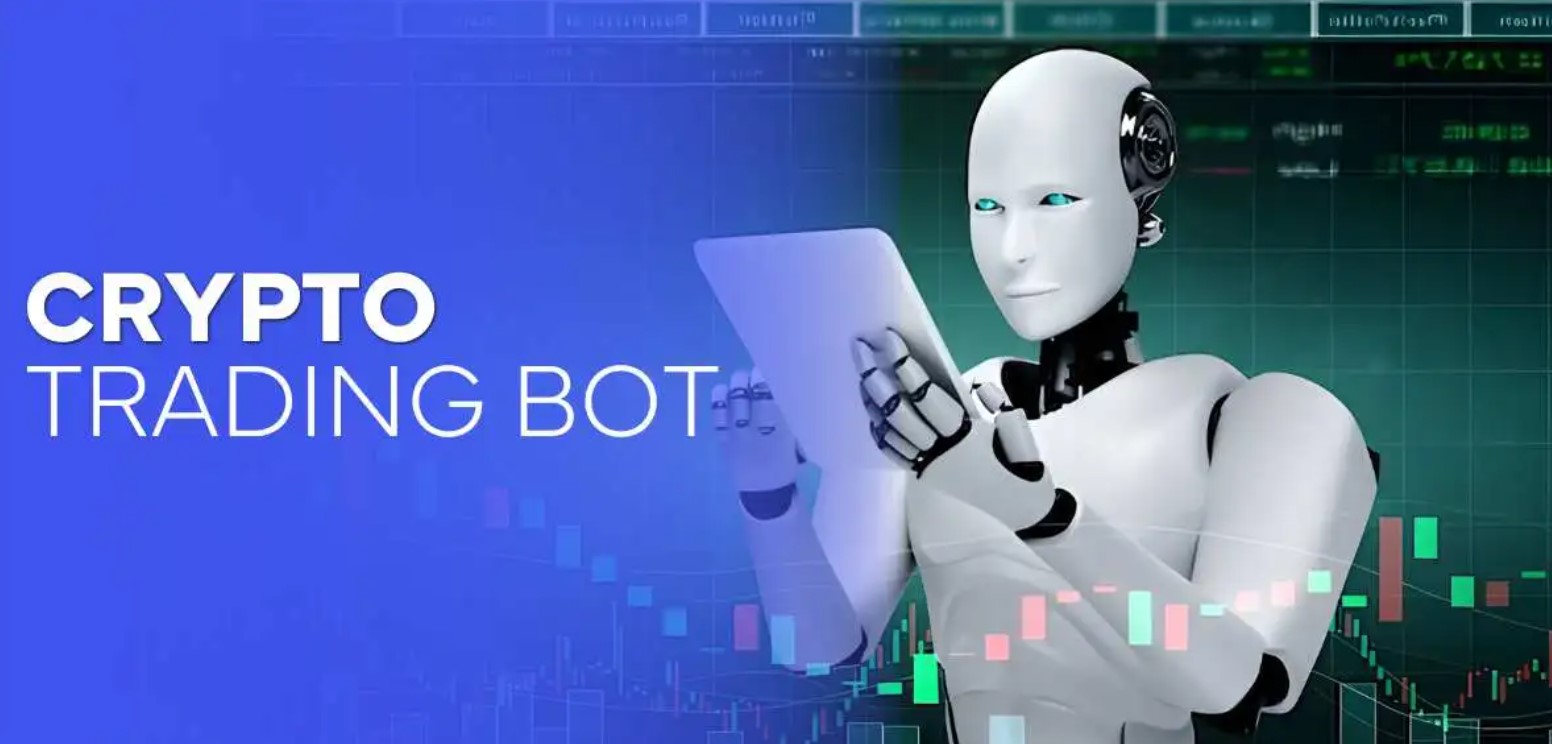
March 14, 2025
Automating Crypto Trading Bots with Python: A Beginner’s Guide
Have you ever thought about how crypto traders can make trades all the time without staying in front of their screens? Cryptocurrency trading bots are the key! These systems can make trades, look at market trends, and handle your account without you having to keep an eye on them all the time. We will show you how to use Python to make your own simple crypto trading bot that will help you trade automatically on sites like Binance and Coinbase Pro.
What You Need to Build a Crypto Trading Bot
Before we start coding, let's look at what you will need to build your trading bot. Python's simple interface and rich libraries make it the most popular automation tool. You need a Binance or Coinbase Pro API key to trade and view live market data.
We will utilize the ccxt library for our project since it connects to over 100 cryptocurrency exchanges. You will use pandas, numpy, and time to track transactions.
Setting Up the Environment
Make sure you have Python installed before you start. Following the above command will install the needed libraries:
pip install ccxt pandas numpy
After that, sign up for Binance or Coinbase Pro and make your API keys. Make sure to keep these keys safe because they will let your bot connect to the exchange. If you do not want to chance real money, it is best to use a demo account or switch the exchange to test mode.
Writing the First Python Script
Let's get to the fun part: writing code for your bot! To start off, we will use the ccxt library to connect to an exchange. This is a simple example of how to sign in to Binance:
import ccxt
exchange = ccxt.binance({
'apiKey': 'your_api_key',
'secret': 'your_secret_key',
})
Connected users may get market data. Now you've to find the latest Bitcoin (BTC) price in USDT (Tether):
ticker = exchange.fetch_ticker('BTC/USDT')
print(ticker)
This shows market price, 24-hour volume, and more. Enter this code to buy 0.001 BTC at market price:
order = exchange.create_market_buy_order('BTC/USDT', 0.001)
print(order)
To this easy and simple buy order, you can add more conditions or set it up to automatically make moves according to the market patterns.
Basic Trading Strategies
After setting up the bot, add some simple trade methods. The best plan is to buy when the price goes down and sell when it goes up. If the price is less than $30,000 or $35,000, your bot can buy and sell Bitcoin.
It is very important to include risk control. With automatic stop-loss or take-profit orders, you can lock in gains and cut down on losses. Trend lines and RSI might help your bot make better choices.
Conclusion and Next Steps
A Python crypto trading bot may be fun and rewarding. This blogpost covers the fundamentals, but there is more to learn, from complex trading methods to bot optimization. Start basic, test in demo mode, then improve your bot as you gain coding and trading strategy confidence.
248 views