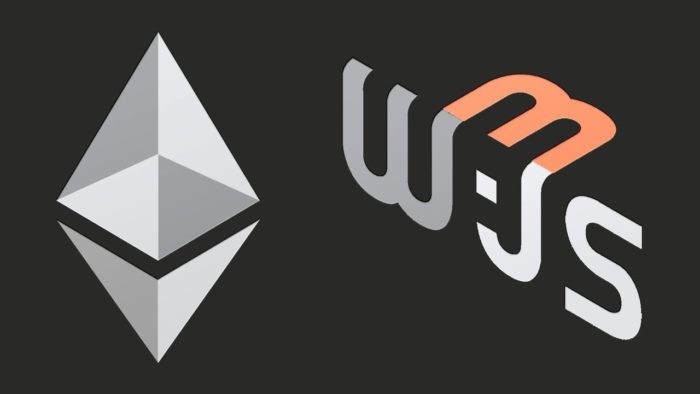
March 12, 2025
Tracking Ethereum Transactions with Web3.js
Have you considered tracking real-time Ethereum transactions? You need real-time transaction tracking to keep up with a smart contract, dApp, or Ethereum blockchain exploration. Web3.js, a sophisticated JavaScript tool, and this blog will let us connect to Ethereum and view live events.
What is Web3.js?
JavaScript utilities called Web3.js allow developers deal with Ethereum. From a web application, it simplifies direct communication with the Ethereum network without the need for advanced blockchain procedures. Web3.js lets programmers send transactions, connect to smart contracts, ask blockchain data inquiries, and monitor real-time events like new blocks and transactions.
Setting Up Web3.js
To use Web3.js, you've to installing the library and set up an Ethereum connection. Let's see the process:
Install Web3.js:
So, to start the process you first have to add Web3.js into your project using npm:
npm install web3
Set Up the Ethereum Connection:
After you install Web3.js, you can connect your JavaScript app to an Ethereum node. Another option is to use Infura, a cloud-based Ethereum node service, if you do not have your own local Ethereum node.
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
When you connect Web3.js to an Ethereum node, you can start to use the Ethereum blockchain and keep track of transactions.
Tracking Transactions with Web3.js
You can begin keeping track of deals once your connection is ready. Applications that need real-time information on smart contract exchanges, token trades, or general blockchain activity must be able to keep an eye on transactions.
Monitoring Transactions:
Web3.js lets you wait for new blocks and get transaction info from them. You can, for instance, sign up to receive new blocks and sort transactions by certain criteria, like those that involve a certain address.
How to subscribe to fresh blocks and fetch transaction details:
web3.eth.subscribe('newBlockHeaders', async (error, blockHeader) => {
if (!error) {
console.log("New block received: ", blockHeader);
const block = await web3.eth.getBlock(blockHeader.hash, true);
console.log("Transactions in this block: ", block.transactions);
}
});
With each block, Ethereum gets sender, recipient, and value data.
Handling Real-Time Updates:
Web3.js can handle real-time updates by listening for certain events like token transfers and smart contract executions. Web3.eth.subscribe lets you subscribe to events in real time, making it simple to follow transactions for specific addresses or contracts.
You may monitor incoming transactions at a certain address:
web3.eth.subscribe('logs', {
address: '0xYourEthereumAddressHere'
}, function(error, result){
if (!error) {
console.log(result);
}
});
Track particular addresses or Ethereum smart contract occurrences in real time.
Conclusion
Ethereum developers must be able to track transactions in real time. Web3.js makes tracking transactions, token transfers, and contract events easy. This makes it useful for dApps and other blockchain initiatives. Web3.js lets you develop smarter, more responsive apps using Ethereum's decentralized network.
140 views