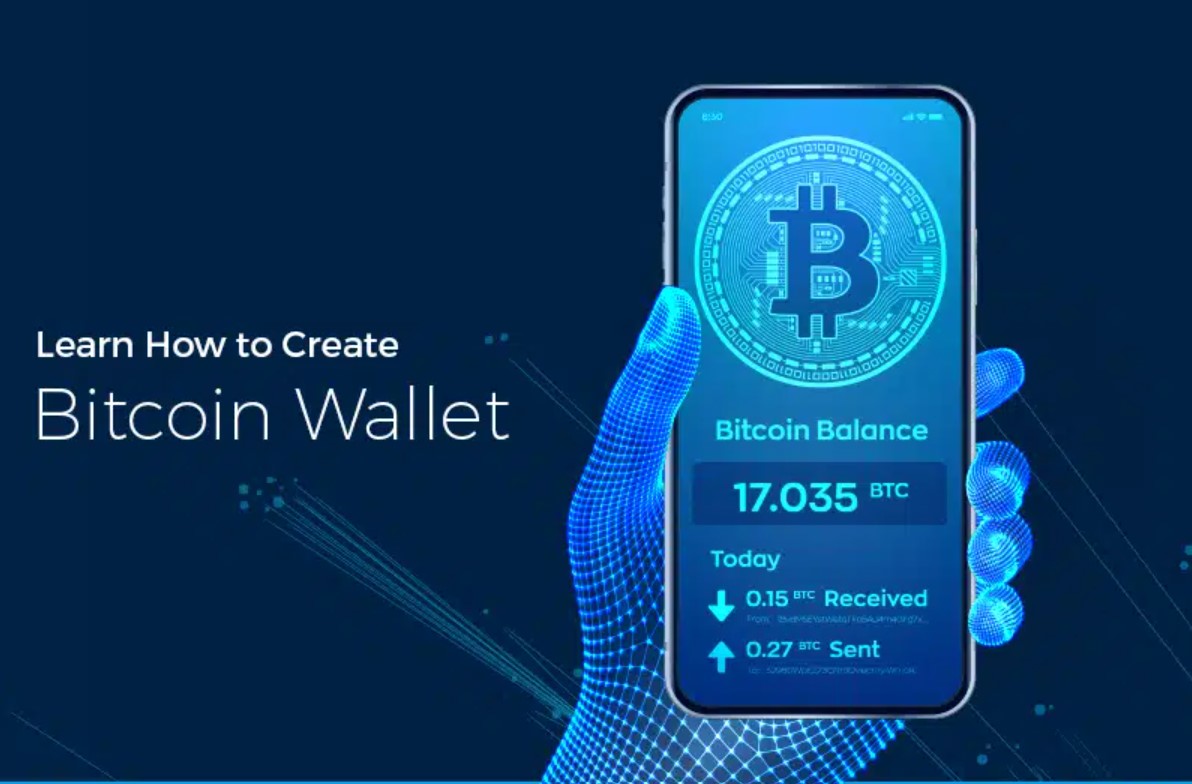
March 11, 2025
Building a Bitcoin Wallet with Python
Have you attempted connecting to Bitcoin using Python? Making your own Bitcoin wallet can be exciting, whether you want to be a blockchain developer or simply like cryptocurrency. You will learn how to make a Bitcoin wallet with Python in this blogpost. You will learn how to create private keys, manage transactions, and code your wallet.
Prerequisites
Please make sure you understand basic Python ideas and how Bitcoin and the blockchain work before we start writing code. We will use libraries for Python, such as bitcoinlib or bit, to connect to the Bitcoin system for this project. You can use pip to load the necessary libraries if you have not already:
pip install bitcoinlib
Setting Up Your Bitcoin Wallet
Making a Bitcoin wallet is the first thing you need to do. You can sign transactions using your private key and receive Bitcoin with your public key.
In Python, you can make a Bitcoin wallet like this:
from bitcoinlib.wallets import Wallet
# Create a new wallet
wallet = Wallet.create('MyBitcoinWallet')
# Print the private and public keys
private_key = wallet.get_key().wif # WIF - Wallet Import Format
public_key = wallet.get_key().address
print(f"Private Key: {private_key}")
print(f"Public Key: {public_key}")
Prints out the private and public keys and makes a new wallet with this code. Remember to protect your Bitcoin private key to avoid losing access to your funds!
Handling Bitcoin Transactions
After setting up your wallet, let's send and receive Bitcoin! There is an input (where the funds comes from) and an output (where the funds goes) in a Bitcoin exchange. To send money, you need to use your private key to sign the transmission and send it to the network.
Here's one way you can use your wallet to send Bitcoin:
from bitcoinlib.wallets import Wallet
# Load an existing wallet
wallet = Wallet('MyBitcoinWallet')
# Specify the recipient address and the amount to send
recipient_address = '1A1zP1eP5QGefi2DMPTfTL5SLmv7DivfNa'
amount = 0.001 # Amount in BTC
# Send Bitcoin
transaction = wallet.send_to(recipient_address, amount)
print(f"Transaction Sent: {transaction.info()}")
In this case, we load our wallet, enter the address and amount of the Bitcoin we want to send, and then send it. The send_to() method creates, signs, and sends the transaction for us.
Security Considerations
When you use Bitcoin, security is very important because the private key gives you full power over the funds in your wallet. If you want to keep your wallet safe, you might want to use a hardware wallet for cold storage. This will keep your secret keys offline and away from hackers. Using a strong password to secure your private keys before saving them is also very important. This adds another level of security. Also, make regular copies of your keys and keep them in several safe places to make sure you can get your money back if you need to. You can keep your Bitcoin wallet safe from theft and loss by taking these steps.
Conclusion
Using Python to make a Bitcoin wallet is a fun way to start learning about cryptocurrencies and blockchain development. It is easy to make your own wallet, send Bitcoin, and start dealing with the Bitcoin network if you follow these simple steps. You can go even further by adding features like checking your balance, connecting to platforms, or even making a full-fledged blockchain app.
171 views