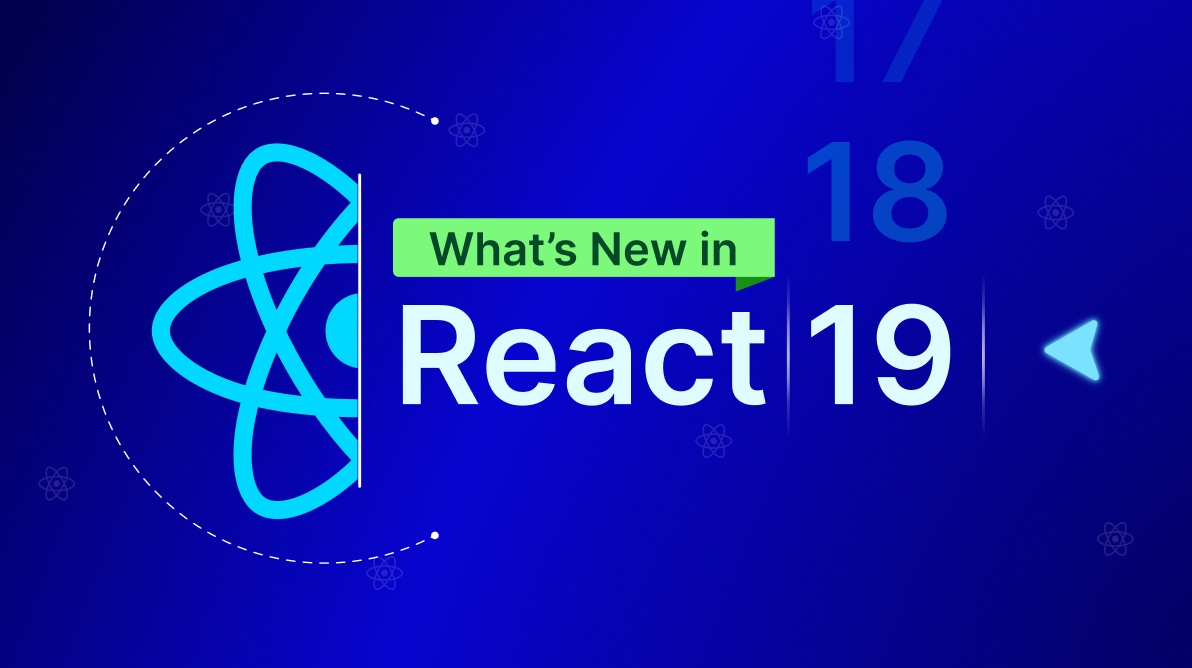
December 10, 2024
React 19: Updates on Server Components, Server Actions, and Improvements
React Server Components
Server Components enable rendering parts of your React app on the server side, either at build time or per request. These components operate in isolation from the client-side application and SSR (Server-Side Rendering) environment, giving developers more flexibility in rendering strategies.
Key Features
Build-Time or Per-Request Execution:
Server Components can run during CI/CD for static generation.
Alternatively, they can execute on-demand for each user request.
Stability:
React Server Components are now stable in React 19, allowing libraries to target React 19 with the react-server export condition.
Guidance for Frameworks:
Frameworks supporting Full-Stack React Architecture can leverage Server Components.
However, the APIs for bundlers or frameworks are not fully stable and may change within the React 19.x version range.
Important Notes:
Server Components do not use the use server directive (this is specific to Server Actions).
For more details, see the React Server Components documentation.
Server Actions
Server Actions allow async server-side function execution from Client Components. They simplify interaction between client and server for specific tasks without full page reloads.
Key Features:
use server Directive:
Indicates a Server Action, enabling frameworks to generate a reference for invocation from the client.
Usage:
Server Actions can be imported and used directly in Client Components.
They can also be defined in Server Components and passed as props to Client Components.
Example:
// Server Component
export async function getUserData(userId) {
return await fetchUserFromDatabase(userId);
}
// Client Component
import { getUserData } from './server-actions';
function UserProfile({ userId }) {
const fetchUser = getUserData(userId);
return <button onClick={fetchUser}>Fetch User Data</button>;
}
Efficiency:
By executing server logic only when needed, Server Actions reduce unnecessary client-side computation.
Improvements in React 19
1. ref as a Prop
Function components now accept ref as a standard prop, removing the need for forwardRef.
A codemod will be available to automate migration.
Example:
function MyInput({ placeholder, ref }) {
return <input placeholder={placeholder} ref={ref} />;
}
2. Hydration Error Improvements
React 19 introduces enhanced error reporting for hydration mismatches, displaying detailed diffs for easier debugging.
Old Logging: Multiple vague warnings with little actionable detail.
New Logging: A single error message with a clear diff of mismatched server and client states.
Provides specific reasons for mismatches, such as differences caused by dynamic content (e.g., Date.now()).
Hydration Error Examples:
Incorrect HTML nesting.
Server/client code divergence (e.g., conditional branches based on window).
Why These Changes Matter
Server Components: Enable greater scalability and performance by offloading logic to the server.
Server Actions: Streamline client-server interactions with server-side async function calls.
ref as a Prop: Simplifies component development by removing the need for extra boilerplate.
Better Error Reporting: Helps developers debug SSR issues efficiently.
React 19 pushes the boundaries of whatââ¬â¢s possible in modern web development, making React applications more robust and developer-friendly. For full documentation, visit the React Dev website.
625 views