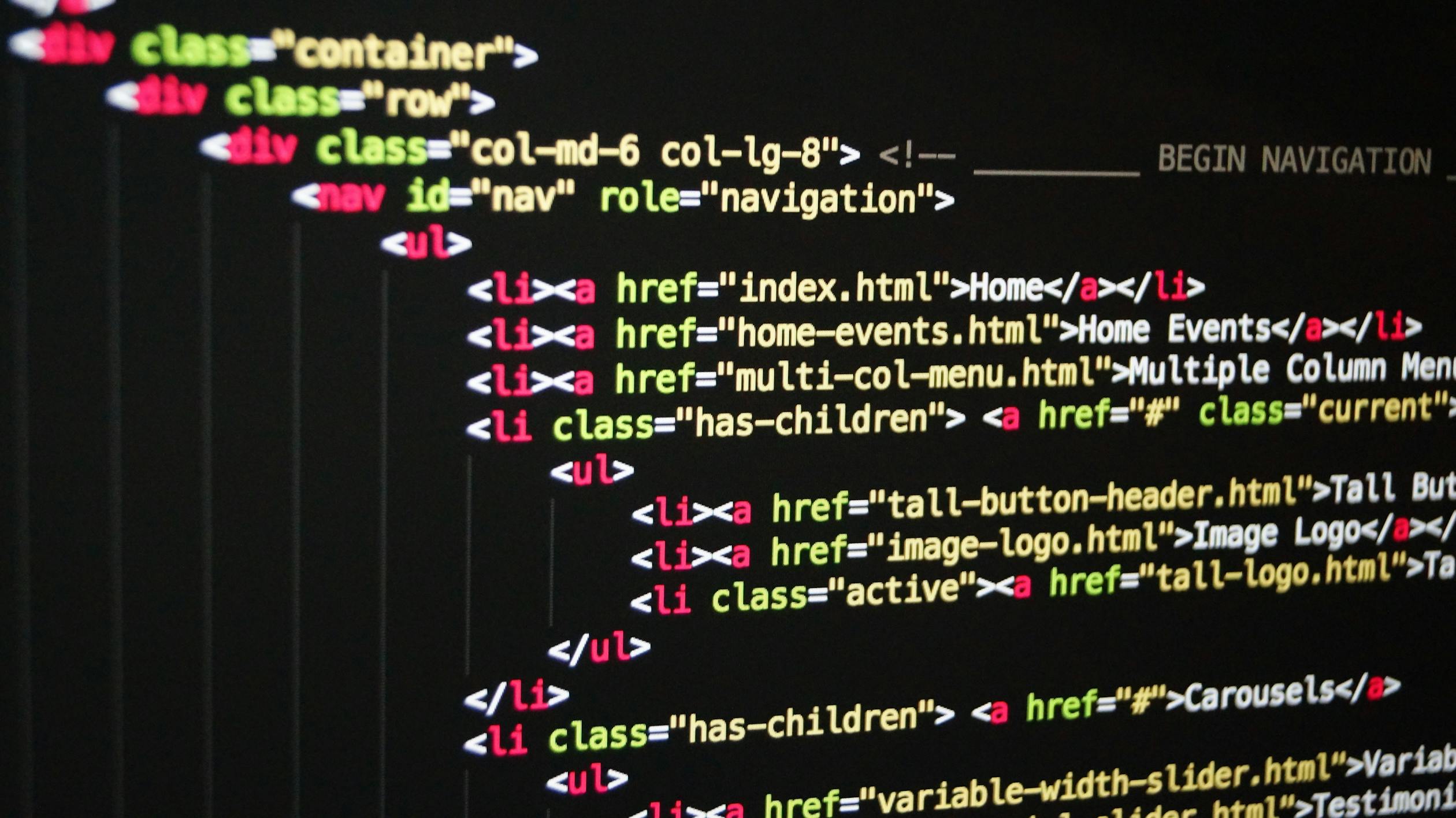
November 28, 2024
Understanding O(n) Time Complexity: A Guide to Linear Efficiency
Introduction
When evaluating the efficiency of algorithms, it is important to understand how they scale with input size. One of the most common time complexities you will encounter in computer science is O(n), also known as linear time complexity. In this article, we will dive deep into what O(n) means, how to identify it, and why it is an important concept for writing efficient code.
What Is O(n)?
O(n) is a notation from Big O notation, which is used to describe the performance of an algorithm as the input size grows. Specifically, O(n) indicates that the algorithms runtime grows linearly with the size of the input, typically denoted by n
.
This means if you double the size of the input, the time it takes to execute the algorithm will also roughly double. It is a direct relationship between the input size and the number of operations the algorithm performs.
Real-World Analogy
Imagine you have a stack of books, and you want to find a particular book by reading through the titles. If the books are in no particular order, you will have to look at each book one by one. The more books you have, the longer it will take to find the one you are searching for. This is an example of O(n) time complexity: the time it takes to complete the task increases linearly with the number of books.
Formal Definition
In Big O notation:
n
represents the size of the input (e.g., the number of elements in an array, the number of nodes in a tree, etc.).- O(n) means that the algorithm performs nbasic operations in the worst case, where the amount of work done increases in direct proportion to the size of the input.
Characteristics of O(n) Algorithms:
- Linear Growth: The number of operations grows linearly with the size of the input. This makes O(n) algorithms quite efficient for moderate input sizes.
- Direct Relationship: Each additional unit of input increases the time taken by a constant amount.
Identifying O(n) Time Complexity
To recognize O(n) time complexity, look for algorithms that process each element of the input exactly once, or a constant number of times for each element. These algorithms typically contain single loops that iterate through the input or perform operations on each element one by one.
Example 1: Iterating Through an Array
function printArray(arr) {
for (let i = 0; i < arr.length; i++) {
console.log(arr[i]);
}
}
In this example, the for
loop runs n
times, where n
is the length of the array. For each iteration, it performs one constant-time operation: printing an element. Since the loop runs once for each element, the total time complexity is O(n).
Example 2: Finding the Maximum Value in an Array
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
In this example, the algorithm checks every element of the array to find the maximum value. It iterates through the entire array once, making the time complexity O(n).
Example 3: Counting Characters in a String
function countCharacters(str) {
let count = 0;
for (let i = 0; i < str.length; i++) {
count++;
}
return count;
}
Here, the algorithm runs a single loop through each character in the string and increments a counter. Again, the loop runs n
times where n
is the length of the string, so the time complexity is O(n).
When Is O(n) Time Complexity Considered Efficient?
O(n) time complexity is typically considered efficient, especially for moderately sized inputs. Here is why:
- Linear Growth: Although O(n) algorithms scale with input size, they do so in a linear fashion, meaning the growth rate is predictable and manageable.
- Real-World Feasibility: For many real-world applications, O(n) time complexity is fast enough to handle input sizes commonly encountered in practice. For example, traversing a list of 10,000 items may still complete in fractions of a second on modern hardware.
However, for extremely large input sizes, even O(n) may become inefficient, especially when more optimized algorithms (e.g., O(log n)) are available.
O(n) vs Other Time Complexities
To appreciate how O(n) compares with other time complexities, let us briefly look at some common ones:
- O(1) (Constant Time):
The algorithm runs in the same amount of time regardless of the size of the input. This is the fastest time complexity. - O(n) (Linear Time):
The time taken grows linearly with input size. For every new unit of input, the runtime increases by a constant factor. - O(log n) (Logarithmic Time):
The time grows logarithmically with the input size. Binary search is a good example of an O(log n) algorithm. - O(nò) (Quadratic Time):
The runtime grows quadratically, often seen in algorithms that involve nested loops. For example, a double loop over an array results in O(n²) time complexity, which is much slower than O(n) for large input sizes.
Visual Comparison:
Input Size (n)O(1)O(log n)O(n)O(nò)1013101001001710010,0001,0001101,0001,000,000
In this table, you can see how O(n) time grows significantly more slowly than O(n2) but more quickly than O(1) and O(log n).
Common Scenarios Where O(n) Is Used
O(n) time complexity is common in many algorithms and real-world problems. Here are a few scenarios where you will often find O(n):
- Array Traversals: Any algorithm that involves processing or scanning every element in an array or list typically runs in O(n) time.
- Linear Search: When you search for a specific element in an unsorted list, you will likely end up using a linear search, which runs in O(n).
- Finding Min/Max: To find the minimum or maximum value in a list, you often need to iterate through all elements, which requires O(n) time.
- Sum of Elements: If you need to compute the sum of elements in an array or list, you will traverse the array once, resulting in O(n) time complexity.
Conclusion
O(n) time complexity, or linear time complexity, is one of the most commonly encountered complexities in algorithm design. It represents an efficient way to solve problems where every element needs to be processed, and it is particularly useful for tasks like searching, counting, or traversing arrays and lists. While it is not the fastest possible complexity (compared to O(1) or O(log n)), O(n) is often sufficient for handling moderately large datasets.
Understanding when and how to use O(n) algorithms is crucial for optimizing your code and improving performance, especially as input sizes grow. By identifying patterns in problem statements and recognizing scenarios where O(n) time is suitable, you can write efficient solutions that perform well in both interviews and real-world applications.
481 views