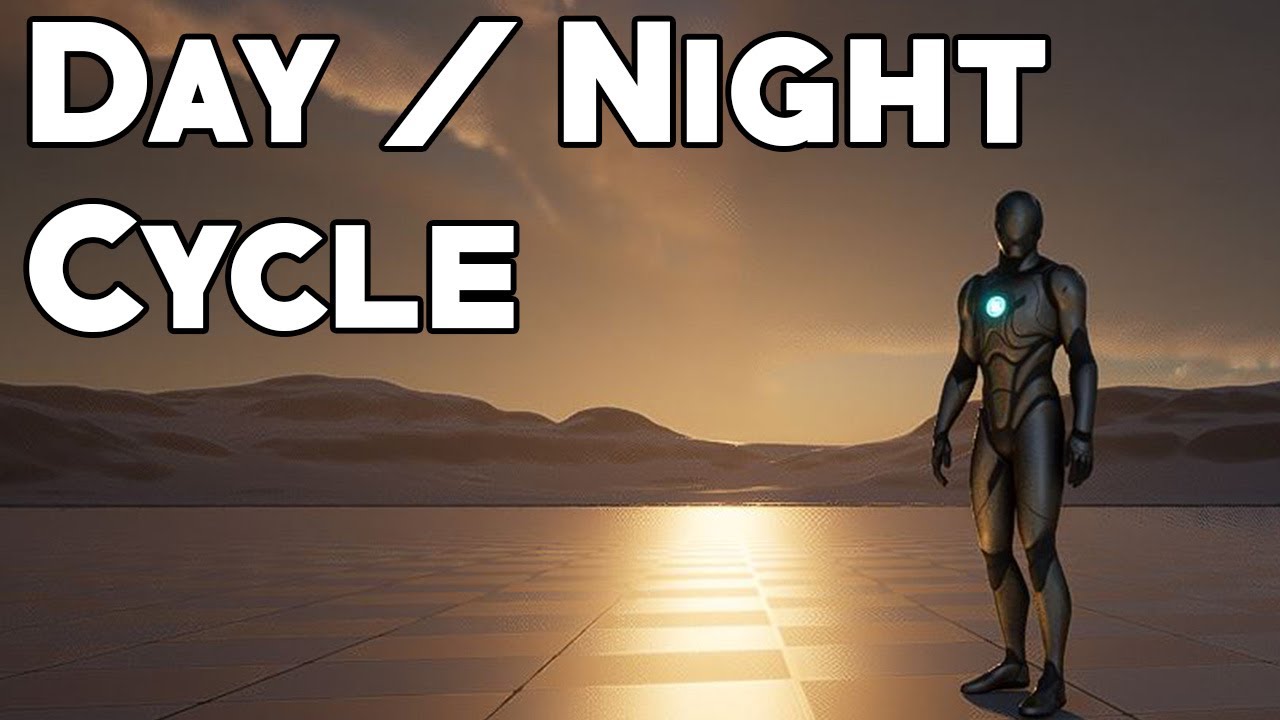
January 01, 2025
Creating a Dynamic Day/Night Cycle in Unity or Unreal Engine
An impressive characteristic of open-world games is their flawless day-to-night transition. Has this crossed your mind? A dynamic day/night cycle is vital for games to engage players and simulate time passing. Even though it looks hard, Unity and Unreal Engine can create a day/night cycle using minimal code. Here's how to add this feature to your game.
Setting Up the Environment
You should have the environment ready for the cycle before you create it. Unity and Unreal Engine provide features for directional lighting, the main light source.
Select Directional Light from Unity's GameObject menu, then Light. This light will imitate the sun if placed where you want it to rise. Create a similar effect in Unreal Engine using the Directional Light actor.
This set-up lets light spin in a way that looks like the sun moving across the sky, creating a realistic change from day to night.
Creating the Time of Day System
After setting up the infrastructure, let us create a time system to govern day and night. Both engines will employ a "time" variable that increases with each frame to simulate a day.
Here's how to set this up in Unity with C#:
public class DayNightCycle : MonoBehaviour
{
public Light sun;
public float timeMultiplier = 1f;
private float currentTime = 0f;
void Update()
{
currentTime += Time.deltaTime * timeMultiplier;
sun.transform.rotation = Quaternion.Euler(new Vector3((currentTime / 24f) * 360f - 90f, 170f, 0));
}
}
CurrentTime tracks time, and timeMultiplier controls this script's cycle. Sun's spin (directed light) varies as currentTime climbs, making it seem to move.
You can use Blueprints or C++ to create this Unreal Engine effect. Use Blueprints' Event Tick node to increment a time variable and update the sun's rotation.
Coding the Light Rotation for Day/Night Transition
Regularly updating the directed light's rotation creates a smooth day/night cycle. The Unity code above spins light based on currentTime.
In Unreal Engine, if you prefer C++, here's a quick example:
void ADayNightCycle::Tick(float DeltaTime)
{
Super::Tick(DeltaTime);
CurrentTime += DeltaTime * TimeMultiplier;
DirectionalLight->SetActorRotation(FRotator((CurrentTime / 24.f) * 360.f - 90.f, 0.f, 0.f));
}
Both methods adjust light rotation to simulate time. Adjust timeMultiplier to alter day/night cycle speed.
Enhancing the Cycle with Skybox and Ambient Effects
With skybox modifications and ambient effects, a dynamic cycle becomes more immersive. Unity lets you change skybox materials or use the Procedural Skybox at various times of day. Adjust your directed light's strength and color for dawn, noon, sunset, and night.
Sky Atmosphere and Exponential Height Fog provide richness to Unreal Engine scenes. You can make it look like it is really that time of day by gently changing the color and brightness of the light.
Testing and Fine-Tuning
After setting up your cycle, run your game and watch how the day and night changes. It takes time for smoother changes and more reality.Change the multiplier, the color of the light, and its strength.
Conclusion
Dynamic day/night cycles boost player immersion and bring your environment to life. Your game world will seem more realistic if you mimic time with a few lines of code in Unity or Unreal Engine. Now its your turn, try various settings and effects to customize your day/night cycle!
676 views