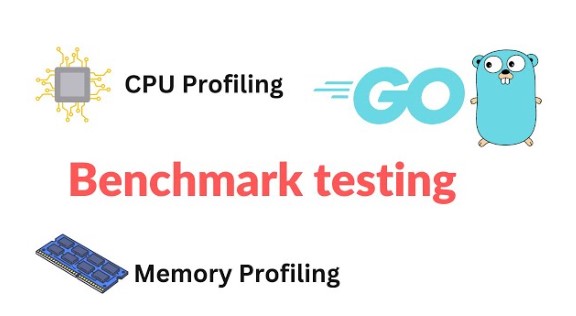
December 25, 2024
Optimizing Go Performance with Profiling and Benchmarking
Want to speed up your Go app? Applications that process vast amounts of data in real time must optimize performance. Profiling and benchmarking in Go help find bottlenecks and boost performance. This article shows how to use Go's built-in tools to find and repair performance problems, improving code efficiency.
Understanding Profiling and Benchmarking in Go
Benchmarking and profiling analyze performance. Profiling CPU and memory usage during execution tends to find resource-intensive regions. To evaluate efficiency, benchmarking examines code segment speed. The built-in testing package and pprof tool in Go make it simple to gather performance statistics and assess how to speed up and optimize your code.
Setting Up a Basic Benchmark
The Go testing package simplifies code benchmarking. To make a benchmark, you need to write a function that starts with "Benchmark" and uses "b.N," which is the number of times Go runs the function to find the average processing time.
Here's an example of a basic benchmark:
package main
import (
"testing"
)
func ConcatenateStrings(a, b string) string {
return a + b
}
func BenchmarkConcatenateStrings(b *testing.B) {
for i := 0; i < b.N; i++ {
ConcatenateStrings("hello", "world")
}
}
Using go test -bench=. to run this benchmark, it runs the code many times and gives you an average speed. Benchmarking lets you quantify optimizations over time by tracking performance gains following code modifications.
Profiling Go Applications
The pprof package simplifies Go profiling by providing CPU and memory usage data. Import net/http/pprof to allow profiling endpoints in web applications or use it directly in CLI applications.
To profile CPU usage in a Go application, add the following:
import (
"os"
"runtime/pprof"
)
func main() {
f, _ := os.Create("cpu.prof")
pprof.StartCPUProfile(f)
defer pprof.StopCPUProfile()
// Your application code here
}
To make a profile file (cpu.prof), run the application. After that, you can use the go tool pprof command to look at the file:
go tool pprof cpu.prof
This opens an interactive interface to analyze CPU-intensive functions. In the same way, you can use memory profiling to find areas that are taking up too much space. You can identify optimizable functions via profiling.
Tips for Performance Optimization Based on Profiling Data
Find high-resource functions and make them better. Here are some well-known strategies to follow:
- Improve Loops: Loops that do not work well can make apps run much slower. Think about making them easier.
- Reduce Memory Allocations: Reuse memory to reduce unnecessary allocations.
- Use Efficient Data Structures: Selecting slices over arrays may save overhead.
Conclusion
So, now you know, Go's profiling and benchmarking tools let you find speed problems in your application and fix them to make it run faster. Here's a simple way to make your Go program work better and more quickly. Start checking its performance right away to improve your Go coding. Start profiling right away to make your Go code better!
482 views