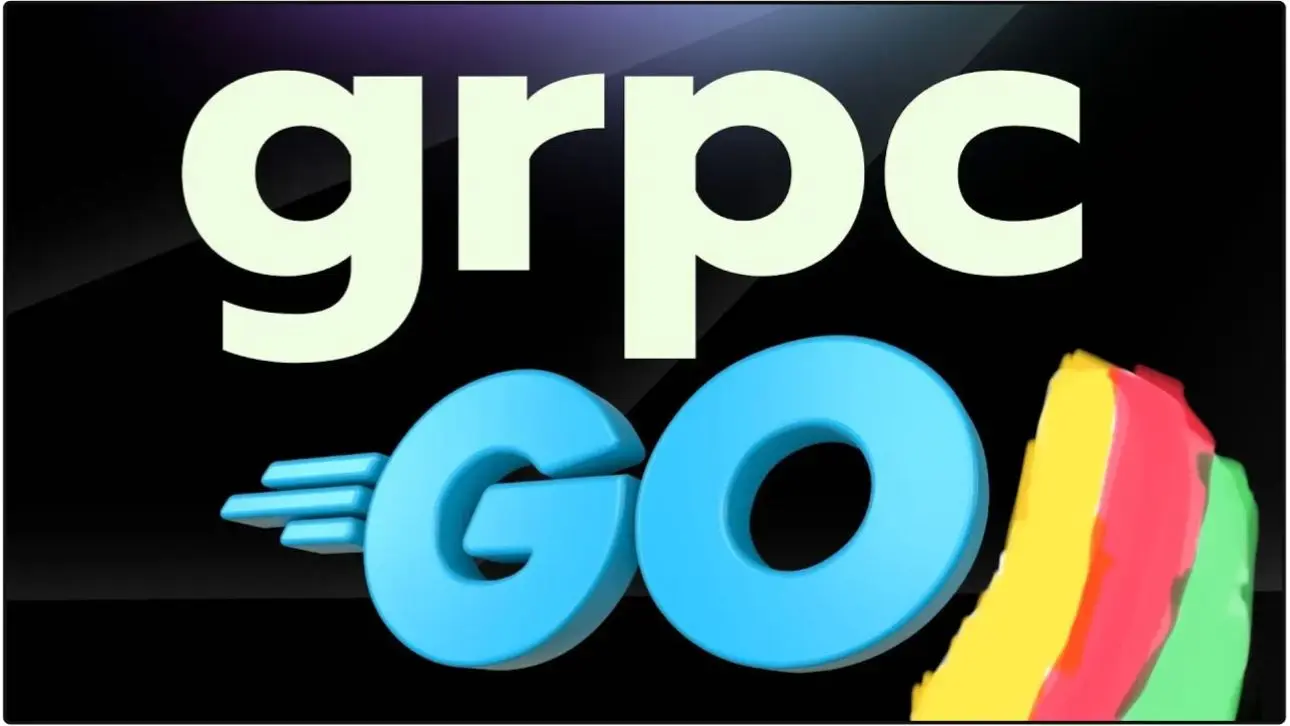
Want to build a fast, scalable microservice with real-time client-server communication? You may need GRPC for this! Google's gRPC accelerates microservice communication by making remote procedure calls (RPCs) efficient. Setting up a simple gRPC service in Go utilizing Protocol Buffers for data serialization and studying unary and streaming RPCs with a file storage server is the focus of this article.
Setting Up Protocol Buffers
We must specify our data and services using Protocol Buffers (protobufs), Google's language-neutral serialization standard, before constructing a gRPC service. Protocol Buffers enable structured data definition and compilation in several languages, including Go.
- Put the Protocol Buffers Compiler (protoc) and the Go plugin (protoc-gen-go) on your computer.
- Create a.proto file specifying our service and messages. A file storage service may feature a file details message and upload option.
Example .proto definition:
syntax = "proto3";
package filestorage;
service FileService {
rpc UploadFile (FileRequest) returns (FileResponse);
rpc ListFiles (FileRequest) returns (stream FileResponse);
}
message FileRequest {
string file_name = 1;
bytes file_data = 2;
}
message FileResponse {
string message = 1;
bool success = 2;
}
It sets up a FileService with two methods: ListFiles (server-streaming RPC) and UploadFile (unary RPC).
Building a gRPC Server in Go
We can now make a gRPC server in Go since our protobuf definitions are ready.
Use protoc to turn the .proto file into Go code:
protoc --go_out=. --go-grpc_out=. filestorage.proto
Put the FileService interface into the Go code. Here's a simple example of how to set up the server and use the UploadFile method:
package main
import (
"context"
"log"
"net"
"google.golang.org/grpc"
pb "path/to/protobufs/filestorage"
)
type server struct {
pb.UnimplementedFileServiceServer
}
func (s *server) UploadFile(ctx context.Context, req *pb.FileRequest) (*pb.FileResponse, error) {
log.Printf("Received file: %s", req.FileName)
return &pb.FileResponse{Message: "File uploaded successfully", Success: true}, nil
}
func main() {
lis, _ := net.Listen("tcp", ":50051")
grpcServer := grpc.NewServer()
pb.RegisterFileServiceServer(grpcServer, &server{})
grpcServer.Serve(lis)
}
This simple service records the file name and returns a success message using the UploadFile method on port 50051.
Client Communication with gRPC
Let us create a Go gRPC client that calls UploadFile to communicate with the server.
- Create client code from the .proto file.
- Connect to the server and perform an RPC call using the client:
package main
import (
"context"
"log"
"google.golang.org/grpc"
pb "path/to/protobufs/filestorage"
)
func main() {
conn, _ := grpc.Dial("localhost:50051", grpc.WithInsecure())
defer conn.Close()
client := pb.NewFileServiceClient(conn)
response, _ := client.UploadFile(context.Background(), &pb.FileRequest{FileName: "test.txt", FileData: []byte("content")})
log.Printf("Response: %s", response.Message)
}
This code connects to gRPC, executes UploadFile, and displays the response.
Implementing Streaming RPCs
By transmitting huge data in smaller bits, streaming RPCs improve efficiency.
- Server-Streaming RPC: Our example's ListFiles method returns file information to the client. How the server would do this:
func (s *server) ListFiles(req *pb.FileRequest, stream pb.FileService_ListFilesServer) error {
for _, file := range mockFileList { // Assuming mockFileList is a list of files
stream.Send(&pb.FileResponse{Message: file.Name, Success: true})
}
return nil
}
- Client-Streaming RPC: Improves memory management by sending data chunks in a stream to the server for big file uploads.
Ideal for real-time file uploads and logging, streaming RPCs allow gRPC services to easily manage massive or continuous data flows.
Conclusion
A Go gRPC service employing Protocol Buffers, unary, and streaming RPCs offers a strong platform for high-performance microservices. With minimum setup, you can build a real-time, effective communication service for many applications. Explore gRPC to build powerful, scalable solutions for modern applications!
845 views