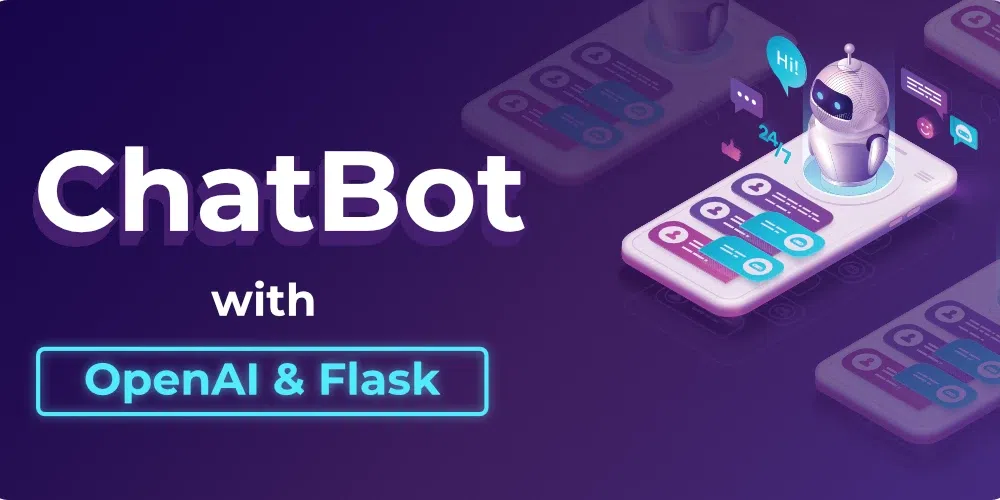
December 11, 2024
Creating Your Own Chatbot with GPT and Flask in 30 Minutes
Hi fresh coders, do you want to make a chatbot but do not know how to code, this post is for you. Making an AI chatbot is easier than you can think! All you need is OpenAI's GPT model and some help from Flask. In just 30 minutes it is possible, you just have to follow the steps in this guide to create your smart assistant.
Prerequisites
It is important to know how to use Python and have an OpenAI API key before you start. You will also need to have Python 3.7 or later and the Flask library loaded on your computer.
What you need:
- Python 3.7+
- OpenAI API key
- Basic knowledge of APIs and Flask
Setting Up Your Environment
First, install Flask and OpenAI's API package. Open your command line and enter:
pip install flask openai
Next, create a new Python file, say chatbot.py. Open this file in your best code editor. It has all the code for your chatbot.
Coding the Chatbot
Create a Basic Flask App
Before making the chatbot, you need to create a simple Flask app. This will interact with the OpenAI API and handle the HTTP requests. Implement this code in chatbot.py:
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route('/chat', methods=['POST'])
def chat():
user_message = request.json.get('message')
# Chatbot response will be generated here
return jsonify({"response": "Chatbot response here"})
Integrate GPT API
Let's use the OpenAI API to give the chatbot some life. You can add this code to the chat() method to handle comments from users:
import openai
openai.api_key = 'YOUR_OPENAI_API_KEY'
@app.route('/chat', methods=['POST'])
def chat():
user_message = request.json.get('message')
response = openai.Completion.create(
engine="text-davinci-003",
prompt=user_message,
max_tokens=50
)
bot_reply = response.choices[0].text.strip()
return jsonify({"response": bot_reply})
Note: Replace 'YOUR_OPENAI_API_KEY' with your actual API key.
Testing Your Chatbot
Running the Flask app locally will let you test your chatbot. For this, go to the project path in the command line and type:
python chatbot.py
In another terminal window, use curl or an API testing tool (like Postman) to send a message to the bot:
curl -X POST -H "Content-Type: application/json" -d '{"message": "Hello!"}' http://127.0.0.1:5000/chat
Deploying the Chatbot
Are you ready to share your chatbot? Platforms like Heroku and Glitch make it easy to put it into use. With these tools, you can put your chatbot online so that anyone can use it.
Conclusion
So, using the robust combination of GPT and Flask, you have made a fully working chatbot in just 30 minutes! You can add more features to this simple setup, like user authentication or memory, to make it perfectly fit your needs.
366 views