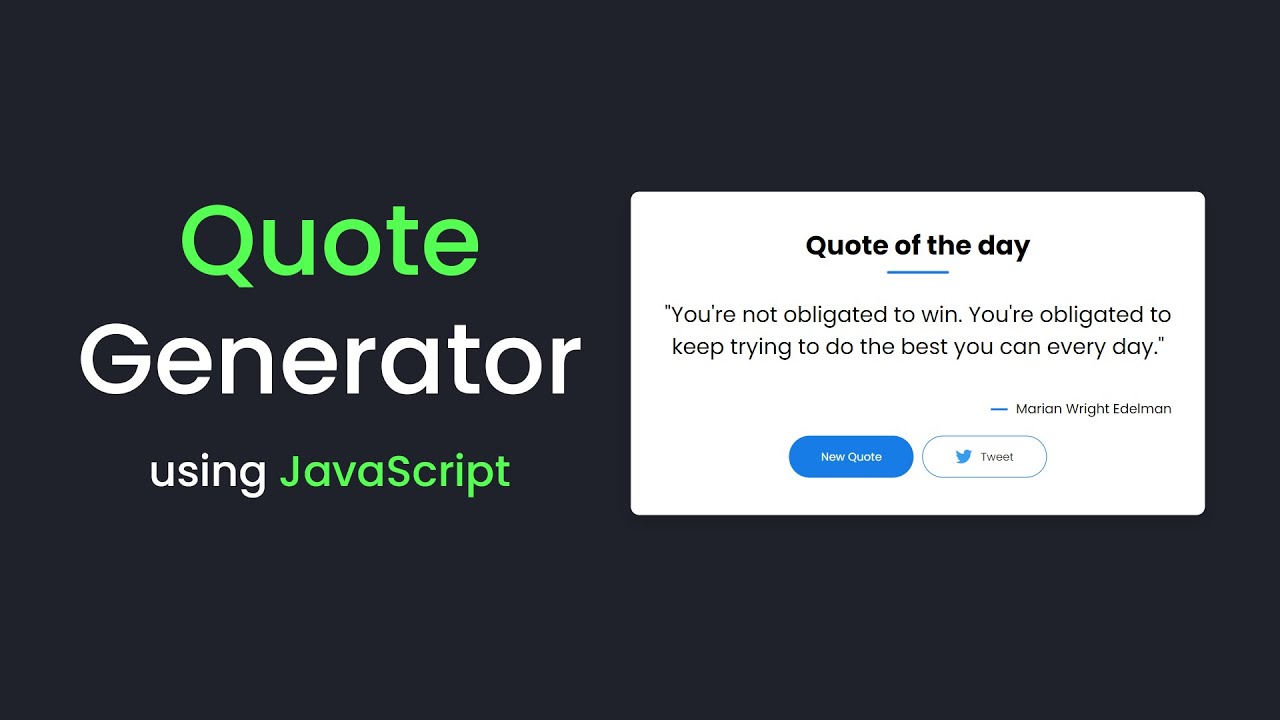
November 25, 2024
Creating a Random Quote Generator with JavaScript
Are you fed up of your life and want some motivation to move on? What about making your own random quote generator with JavaScript? Exciting Right? Let's make it.
Why Build a Random Quote Generator?
You know quotes have a great impact on a person's life to motivate and inspire them. A random quote generator can give you daily wisdom, make one is exciting and fun too. Moreover, by creating this random quote generator you're not only motivating yourself but you're JavaScript skills will also get polished, by understanding the concepts of, arrays, functions and DOM (Document Object Model) manipulation. Let's get into its implementation.
Setting Up the Project
Before start developing, first you'll need the basic structure containing three main files:
- HTML (index.htm): This main file with hold the main structure of quote generator, like button to generate quote and a specific are to show the quotes.
- CSS (style.css): This file helps to style the applications and make it appealing.
- JavaScript (script.js): And this file will contain all code to make quote generator functional and in working state.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="style.css">
<title>Random Quote Generator</title>
</head>
<body>
<div id="quote-container">
<p id="quote">Press the button for a quote!</p>
<button id="new-quote-btn">New Quote</button>
</div>
<script src="script.js"></script>
</body>
</html>
Now you've to create a pre-defined array of quotes to get randomly. This array will act like a database from which when button clicked the generator will show a random quote.
const quotes = [
"The best way to predict the future is to create it.",
"Life is what happens when you're busy making other plans.",
"You only live once, but if you do it right, once is enough.",
"The purpose of our lives is to be happy."
];
Implementing the Random Quote Function
So, once you're done creating an array, it is time to create a function to select random quotes. For this, I'll use Math.random() to generate random index so that your app can retrieve a quote from array randomly.
function getRandomQuote() {
const randomIndex = Math.floor(Math.random() * quotes.length);
return quotes[randomIndex];
}
Updating the DOM
So, now you've created an array of quotes as database and also define a function to randomly get those quotes. Now it's time to show these quotes on webpage. And you can do this by updating the text
const quoteElement = document.getElementById('quote');
const button = document.getElementById('new-quote-btn');
button.addEventListener('click', () => {
const randomIndex = Math.floor(Math.random() * quotes.length);
quoteElement.textContent = quotes[randomIndex];
});
Conclusion
Developing a random quote generator is a pleasant creative and coding task. It inspires you everyday and improves your JavaScript skills. Building this application taught you array manipulation, function implementation, and DOM interaction. Add your favorite quotations, modify themes, and try new features like sharing quotes on social media! Endless possibilities.
Create and customize your own random quote generator. Happy coding!
344 views