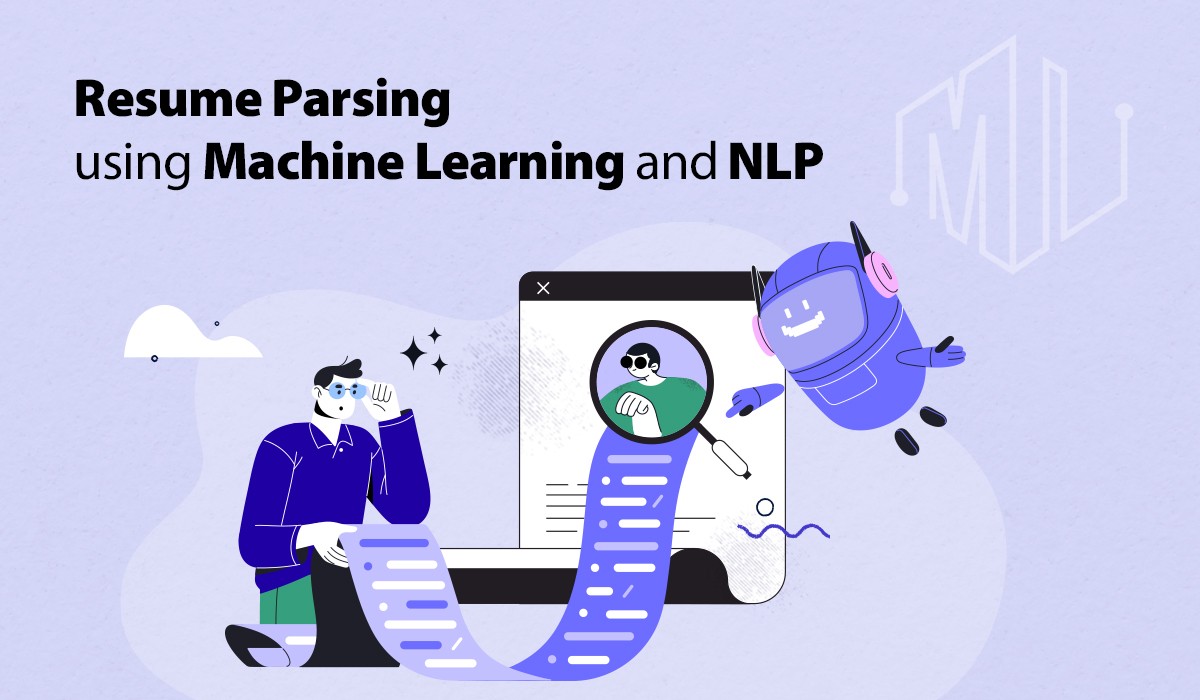
November 18, 2024
Creating an AI-Based Resume Parser Using NLP
Are you running a big business and have no time to analyze all candidate's resumes? No worries, I've a solution for this problem, you can build your own ai-based resume parser using NLP that can automatically reads and understands resumes. Exciting right?
Using machine learning and NLP (Natural Language Processing), it is possible now. Imagine how is it would if you don't have to read each resume manually and AI do this work for you, like thousands of resumes in a few seconds.
That's why, in this blog post, I'll give you a step by step guide to create an AI-based resume parser from scratch using NLP.
What is a Resume Parser?
A resume parser is an efficient program that can analyze numerous resumes at a time and extracts useful information from it such as; applicant's name, contact details, work experience, education, etc. After that this program organizes the extracted information in such a way that companies can get it easily using filters and search queries. So, in this way employers get rid of manually screening resumes one by one, in just a few clicks. Let's see how to make one.
Using NLP for Resume Parsing
As you know Natural Language Processing helps to understand and analyze text. That's why I'm using NLP techniques while creating resume parser to process text and extract meaningful information.
In Python, I'll use popular libraries; spaCy, nltk, and Transformers to build parsers. Let's discuss the important elements:
- Tokenization: This process splits the text into smaller tokens like words and sentences.
- Named Entity Recognition: It will recognize and extract entities like; names, contact details, experience, etc.
- POS Tagging: This will handle the grammatical structure to understand applicant's achievements and tasks.
Key Components and Code Implementation
1. Tokenization
For tokenization, means splitting resume text into separate words, you can use spaCy:
import spacy
nlp = spacy.load('en_core_web_sm')
def tokenize_resume(text):
doc = nlp(text)
tokens = [token.text for token in doc]
return tokens
# Example Usage
resume_text = "John Doe, Senior Software Engineer with 5 years of experience."
print(tokenize_resume(resume_text))
2. Named Entity Recognition (NER)
For labeling and extracting entities like; names, job titles, duties, contact details, and more, I'll use spaCy's pre-trained NER model:
def extract_entities(text):
doc = nlp(text)
entities = [(ent.text, ent.label_) for ent in doc.ents]
return entities
# Example Usage
print(extract_entities(resume_text))
3. Skill Extraction
Next component is keyword-based skill extraction, helpful in extracting applicant's skills from resumes. Here employer can give a preset list of skills to match the resumes for relevant job.
skills_list = ["Python", "Java", "Machine Learning", "NLP", "Data Analysis"]
def extract_skills(text):
extracted_skills = []
for skill in skills_list:
if skill.lower() in text.lower():
extracted_skills.append(skill)
return extracted_skills
# Example Usage
print(extract_skills(resume_text))
4. Handling Different File Formats
You know resumes come in multiple file formats, like word or pdf. So to tackle this I'll use libraries like; PyPDF2 for pdf parsing or python-docx for Word files. But in this example I've used pdf library:
import PyPDF2
def extract_text_from_pdf(pdf_path):
with open(pdf_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
text = ""
for page in range(reader.numPages):
text += reader.getPage(page).extract_text()
return text
# Example Usage
print(extract_text_from_pdf('resume.pdf'))
Steps to Build an AI-Based Resume Parser
Let's now discuss the steps for creating AI-based resume parser:
- Data Collection: The first step is to get a dataset and load it in your model.
- Text Preprocessing: Next step is to clean your data like remove special characters, spaces, stop words; and normalize text to remove redundancy and ensure uniformity.
- Feature Extraction: Here you can use NLP techniques like Named Entity Recognition (NER) and POS tagging to extract key details.
- Model Training: Now train an NER model using libraries like spaCy or Hugging Face Transformers if your niche requires specific entity types.
- Testing and Validation: Now the last step, test your parser with some real resumes, and check its accuracy.
Conclusion
Building an AI-based resume parser using NLP is an interesting and valuable project for companies trying to speed up the hiring process. Tokenization, named entity recognition, and machine learning can simplify resume screening.
So, build your own resume parser and start a more efficient recruiting process.
100 views