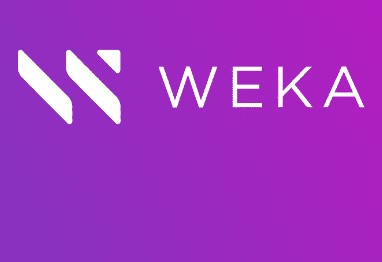
November 08, 2024
Using Weka for Data Preprocessing and Classification
How data scientists turn raw data into worth actioning insights? Any idea? You can also do this transformation easily if you have the right tools. And here comes Weka. Weka is a suite of machine learning software that makes it easier to prepare data for classification and one of the easiest to use and most powerful tools for this job. So, in this article, learn how to load datasets, preprocess data, and develop classification models using Weka.
What is Weka?
Weka, which stands for "Waikato Environment for Knowledge Analysis," is a free and open-source software suite that has many machine learning methods for data mining jobs. Weka's graphical user interface (GUI) makes it easy for anyone, from students to experienced data scientists, to analyse information without knowing a lot about code. The software works with many different types of data, so users can easily import datasets and use different machine learning techniques for tasks like clustering, regression, and classification.
Importance of Data Preprocessing
A very important part of any machine learning task is preparing the data. Raw data is often disorganised and has problems like missing numbers, noise, and traits that don't matter. These problems can have a major impact on performance of your machine learning models. You can make your data better by preprocessing it, which will lead to more accurate and reliable classification results. Cleaning, transforming, and normalising data are common steps in data preprocessing.
Steps for Data Preprocessing in Weka
Here's how to use Weka to preprocess your data:
- Loading Data: Let's start preprocess your data. First, you've to use the Graphical User Interface (GUI) or write code to load your data to Weka.
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class LoadData {
public static void main(String[] args) throws Exception {
DataSource source = new DataSource("your-dataset.csv");
Instances data = source.getDataSet();
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1); // Set class attribute
}
System.out.println(data);
}
}
In this example we've use Java and Weka's API to load a CSV file:
- Data Cleaning: Take care of missing values by either eliminating the instances or adding them in. This can be done with Weka's filters. So, to remove the instances with missing values, you can use this code:
import weka.filters.Filter;
import weka.filters.unsupervised.instance.RemoveWithValues;
RemoveWithValues filter = new RemoveWithValues();
filter.setInputFormat(data);
filter.setAttributeIndex("1"); // Specify attribute index with missing values
filter.setMissingValue("?");
Instances cleanedData = Filter.useFilter(data, filter);
- Data Transformation: You can use the StringToWordVector filter or another good filter to transform category attributes into numbers.
- Normalisation: Normalise your data to make sure that every feature gives the same value to the model:
import weka.filters.unsupervised.attribute.Normalize;
Normalize normalize = new Normalize();
normalize.setInputFormat(cleanedData);
Instances normalizedData = Filter.useFilter(cleanedData, normalize);
- Feature Selection: Use Weka's built-in feature selecting utilities to keep only the important and relevant functions.
import weka.filters.supervised.attribute.RemoveUseless;
RemoveUseless removeUseless = new RemoveUseless();
removeUseless.setInputFormat(normalizedData);
Instances selectedData = Filter.useFilter(normalizedData, removeUseless);
Classification in Weka
After preprocessing your data, now you can move on to classification. Decision Trees (J48), Naive Bayes, and Support Vector Machines (SVM) are some of the classification techniques that Weka offers. Let's see how you can create a sample classification model:
- Selecting a Classifier: Pick out the right classifier. Such as, the J48 decision tree classifier:
import weka.classifiers.trees.J48;
J48 classifier = new J48();
classifier.buildClassifier(selectedData);
- Running the Classifier: Now evaluate your model using cross-validation or a separate test set:
import weka.classifiers.Evaluation;
Evaluation evaluation = new Evaluation(selectedData);
evaluation.crossValidateModel(classifier, selectedData, 10, new Random(1));
System.out.println(evaluation.toSummaryString());
- Interpreting Results: At the end, let's interpret the results. Use Weka's accuracy, precision, and recall metrics to evaluate your classifier's efficiency.
Conclusion
Data preprocessing and classification in Weka offer valuable insights. This guide helps you load datasets, preprocess data, and build strong classification models. Try Weka with your datasets now. A few clicks could lead to your next data discovery!
807 views