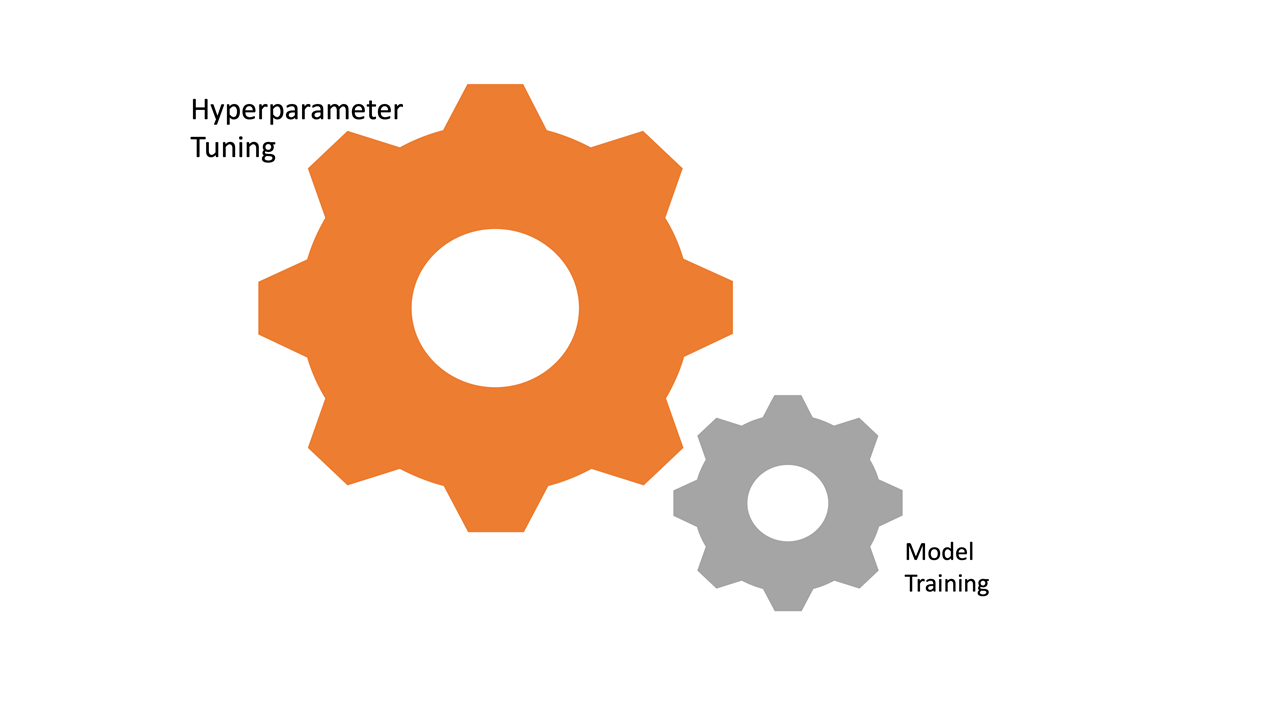
November 07, 2024
Hyperparameter Tuning in Machine Learning Models Using Java
You know sometimes it's very hard get high accuracy of machine learning models, and sometimes they don't show that much accurate results? You know the reason behind this? No worries, I've solution. Start tuning your model's hyperparameters. Hyperparameter tuning changes the settings that decide how models learn. I will talk about why hyperparameter tuning is important and give will you some Java tips to make your model run faster.
Understanding Hyperparameters
Configurations that affect how a machine learning model is trained are called hyperparameters. Instead of learning from data like neural network weights, hyperparameters are tuned before training. This includes the learning rate, the number of hidden layers in a neural network, and the decision tree's best depth, among other factors. Examples include:
- Learning Rate: Determines how quickly a model updates its weights.
- Number of Trees: In ensemble techniques like Random Forests, this hyperparameter affects prediction tree count.
Why Hyperparameter Tuning is Important?
Tuning hyperparameters is very important for getting the best model results. It can have significant impacts on accuracy and help keep things from overfitting and overfitting. A well-tuned model can better adapt to data it hasn't seen before. This makes sure that it not only does well on training data but also makes accurate predictions in the real world. In the end, hyperparameter tuning that works well can make the difference between a model that works on average level and one that works really well.
Methods of Hyperparameter Tuning
Grid Search
Grid search extensively searches a predetermined set of hyperparameters. It looks at all the possible combinations of given numbers to find the best-performing one.
- Pros: It's easy and complete.
- Cons: Costs a lot to computation, especially when there is a lot of hyperparameters space.
Random Search
However, random search selects a fixed number of hyperparameter possibilities from a distribution. Most of the time, this method works better and faster than grid search.
- Pros: More effective, particularly in high-dimensional spaces.
- Cons: It might not find the optimum set of hyperparameters.
Bayesian Optimization
You can use a more advanced method called Bayesian optimization to pick the best hyperparameters to test next. It does this by creating a statistical model of the desired function. This approach can greatly minimize the evaluations.
- Pros: It works better than grid and random search.
- Cons: It's harder to understand and put into action.
Implementing Hyperparameter Tuning in Java
There are many machine learning libraries in Java, like Weka and Java-ML, that have built-in techniques for hyperparameter tuning. For a decision tree classification, I came up with a simple example of how to use Weka's GridSearch:
import weka.classifiers.trees.J48;
import weka.classifiers.meta.GridSearch;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class HyperparameterTuningExample {
public static void main(String[] args) throws Exception {
// Load dataset
DataSource source = new DataSource("path/to/your/dataset.arff");
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1);
// Set up the classifier
J48 classifier = new J48();
// Set up the grid search
GridSearch gridSearch = new GridSearch();
gridSearch.setClassifier(classifier);
gridSearch.setEvaluationMeasure("accuracy");
// Define the hyperparameter grid
String[] options = {
"-C", "0.1", "0.2", "0.3", // Confidence factor
"-M", "2", "4", "6" // Minimum number of instances per leaf
};
gridSearch.setOptions(options);
// Perform the grid search
gridSearch.buildClassifier(data);
System.out.println("Best classifier: " + gridSearch.getBestClassifier());
}
}
Conclusion
Machine learning workflows need hyperparameter tuning to turn an average model into an effective predicting utility. You can maximize your model's potential by trying grid search, random search, and Bayesian optimization. So, start tuning hyperparameters in Java today to optimize your models!
392 views