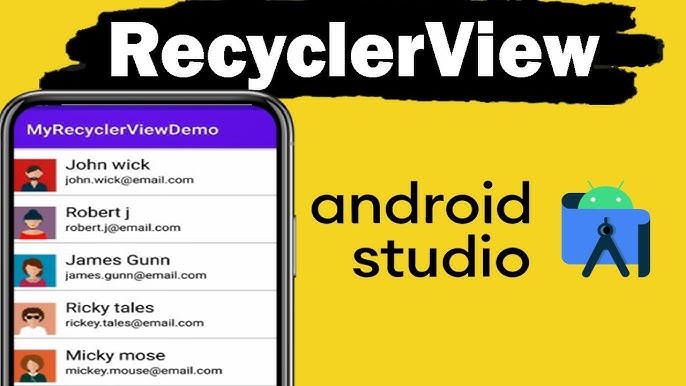
October 29, 2024
Mastering RecyclerView: Creating Dynamic Lists in Android
Hi fellas, I know most of you are thinking why these Android apps with large list of items don't get slow or crashed? Don't you worry, RecyclerView is the answer of all your queries. It plays an important part in making your apps work efficiently and user-friendly by building dynamic lists.
In this guide, I'll guide you on how to setup RecyclerView, create and adapter, manage click events, and personalize list layouts to fit different app needs.
What is RecyclerView?
RecyclerView is the most credible and powerful UI element for displaying large scrollable list items. RecyclerView is more efficient and flexible rather than ListView. As it offers the finest performance by reusing item views by view recycling. This mechanism improves memory usage, especially when you're dealing with complex layouts or large list items.
Here's a point to note that RecyclerView doesn't manage the layout of list items, instead it just works with layout mangers, adapters, and view holders to make user experience responsive and smooth.
Setting Up RecyclerView in Android
I hope you've a clear understanding of what RecyclerView is. Now let's add it in your android project. For this, you've to add the following dependencies in your build.gradle file:
implementation 'androidx.recyclerview:recyclerview:1.2.1'
Next, in your XML layout file, add a RecyclerView widget like this:
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
As I described earlier, RecyclerView doesn't work itself alone, it requires an adapter to bind data to the list, and a layout manager too for displaying list items.
Creating an Adapter for RecyclerView
An adapter connects the data to the RecyclerView. It helps to bind the dataset to the views that show every single item in the list. Here's a simple code for creating adapter:
class MyAdapter(private val dataList: List<String>) : RecyclerView.Adapter<MyAdapter.ViewHolder>() {
class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
val textView: TextView = itemView.findViewById(R.id.textView)
}
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.textView.text = dataList[position]
}
override fun getItemCount(): Int = dataList.size
}
The adapter links a simple list of strings to a TextView inside each item of the RecyclerView in the above code. ViewHolder stores a reference to the view of each item. Meanwhile, onCreateViewHolder() expands the layout of the items, and onBindViewHolder() gives the data to the views.
Handling Click Events in RecyclerView
After creating an adapter, now you've to make your list functional. For this you need to handle click events on individual items by changing onBindViewHolder() method to add a click listener.
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.textView.text = dataList[position]
holder.itemView.setOnClickListener {
Toast.makeText(holder.itemView.context, "Clicked: ${dataList[position]}", Toast.LENGTH_SHORT).show()
}
}
This code will show a Toast message whenever you click a list item. Moreover, you can also make it appealing by passing callbacks or navigating to different pages according to user interaction.
Customizing RecyclerView Layout
If you don't know this fact before, let me clear this for you, RecyclerView supports multiple layout managers to show list items in different patterns. Let's discuss them:
- LinearLayoutManager: Displays items in a vertical or horizontal list.
recyclerView.layoutManager = LinearLayoutManager(this)
- GridLayoutManager: Displays items in a grid.
recyclerView.layoutManager = GridLayoutManager(this, 2) // 2 columns
- StaggeredGridLayoutManager: Displays items in a staggered grid, often used for Pinterest-style layouts.
recyclerView.layoutManager = StaggeredGridLayoutManager(2, LinearLayoutManager.VERTICAL)
Conclusion
RecyclerView is an essential component for creating dynamic lists in Android apps. By setting up an adapter, handling click events, and customizing layouts, you can build highly efficient and user-friendly lists. Explore further by experimenting with more complex layouts and adapters, and you'll quickly master the art of RecyclerView development.
534 views