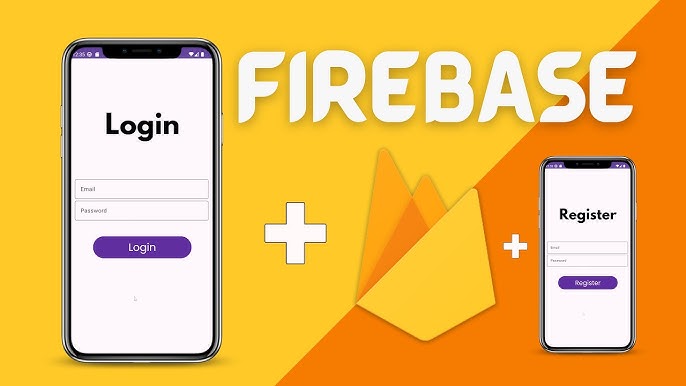
October 28, 2024
Integrating Firebase for Android Authentication: Full Coding Guide
Do you have any idea how the popular apps manage to save user logins and sign-up? The answer revolves around Firebase, which is the most powerful platform for authenticating Android apps in a simpler way. Firebase makes it very easy and helpful for developers to create secure and reliable login systems, no matter they are creating Google Sign In or email/password authentication. So, today in this guide, I came up with step by step procedure for integrating Firebase for authentication in Android.
Setting Up Firebase in Your Android Project
So, before starting to go deep with Firebase, let's first install or setup Firebase on your Android project.
- From Firebase Console, create a new project.
- Now give your application's package name to register your Android app in the project.
- Next, download the google-services.json file given by Firebase and add it in the app directory of your Android project.
After these steps, now add the following dependencies in your build.gradle file to integrate Firebase SDK in your Android project.
// Add this in the app-level build.gradle
implementation 'com.google.firebase:firebase-auth:21.0.1'
// Sync the project with Gradle files
By syncing the project, you've linked your app with Firebase, now you can enable authentication methods and can implement user authentication.
Enabling Firebase Authentication Methods
You can enable authentication using different methods given by Firebase such as, Google Sign-In, Email/Password, and so on. You just have to choose from the given options.
- Go to Firebase Console, select your project from there, and then navigate to Authentication.
- Next, click on Sign-in Method, and choose your preferred methods such as, Google Sign-In, or Email/Password.
And don't forgot to restrict the authentication system to follow security policies, such as, creating strong passwords for Email/Password authentication and using OAuth tokens for Google Sign-In for data security.
Implementing Firebase Email/Password Authentication
You can create Email/Password authentication very easily using Firebase. Let's see process of user registration first:
User Registration
Using the following code, you'll first create a Signup or registration form where users can input their Email and Passwords for the first time. Then, use Firebase to register.
val email = userEmailInput.text.toString()
val password = userPasswordInput.text.toString()
FirebaseAuth.getInstance().createUserWithEmailAndPassword(email, password)
.addOnCompleteListener { task ->
if (task.isSuccessful) {
// User registered successfully
val user = FirebaseAuth.getInstance().currentUser
// Handle user account
} else {
// Handle registration error
Toast.makeText(context, "Registration failed", Toast.LENGTH_SHORT).show()
}
}
User Login
After registering, now it is time to authenticate your credentials like this:
FirebaseAuth.getInstance().signInWithEmailAndPassword(email, password)
.addOnCompleteListener { task ->
if (task.isSuccessful) {
// User logged in successfully
val user = FirebaseAuth.getInstance().currentUser
} else {
// Handle login error
Toast.makeText(context, "Login failed", Toast.LENGTH_SHORT).show()
}
}
Adding Google Sign-In with Firebase
Google Sign-in is the most convenient login experience as you don't need to create a new user. Let's see how you can integrate Google Sign-In in your project using Firebase:
- First, enable Google Sign-In in the Firebase Console under Authentication > Sign-in Method.
- Next, add the below dependencies to your build.gradle file:
implementation 'com.google.android.gms:play-services-auth:19.2.0'
- And at the end, configure Google Sign-In in your activity:
val googleSignInClient = GoogleSignIn.getClient(this, GoogleSignInOptions.DEFAULT_SIGN_IN)
val signInIntent = googleSignInClient.signInIntent
startActivityForResult(signInIntent, RC_SIGN_IN)
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == RC_SIGN_IN) {
val task = GoogleSignIn.getSignedInAccountFromIntent(data)
val account = task.getResult(ApiException::class.java)
firebaseAuthWithGoogle(account.idToken)
}
}
private fun firebaseAuthWithGoogle(idToken: String?) {
val credential = GoogleAuthProvider.getCredential(idToken, null)
FirebaseAuth.getInstance().signInWithCredential(credential)
.addOnCompleteListener { task ->
if (task.isSuccessful) {
// Google sign-in successful
val user = FirebaseAuth.getInstance().currentUser
} else {
// Handle error
Toast.makeText(context, "Google Sign-In failed", Toast.LENGTH_SHORT).show()
}
}
}
Conclusion
Integrating Firebase Authentication into your Android app can be seamless and secure with just a few steps. Whether you're using Email/Password or Google Sign-In, Firebase simplifies the process of managing user authentication. Dive deeper into Firebase to explore additional services like cloud storage, real-time databases, and more to enhance your app's functionality.
302 views