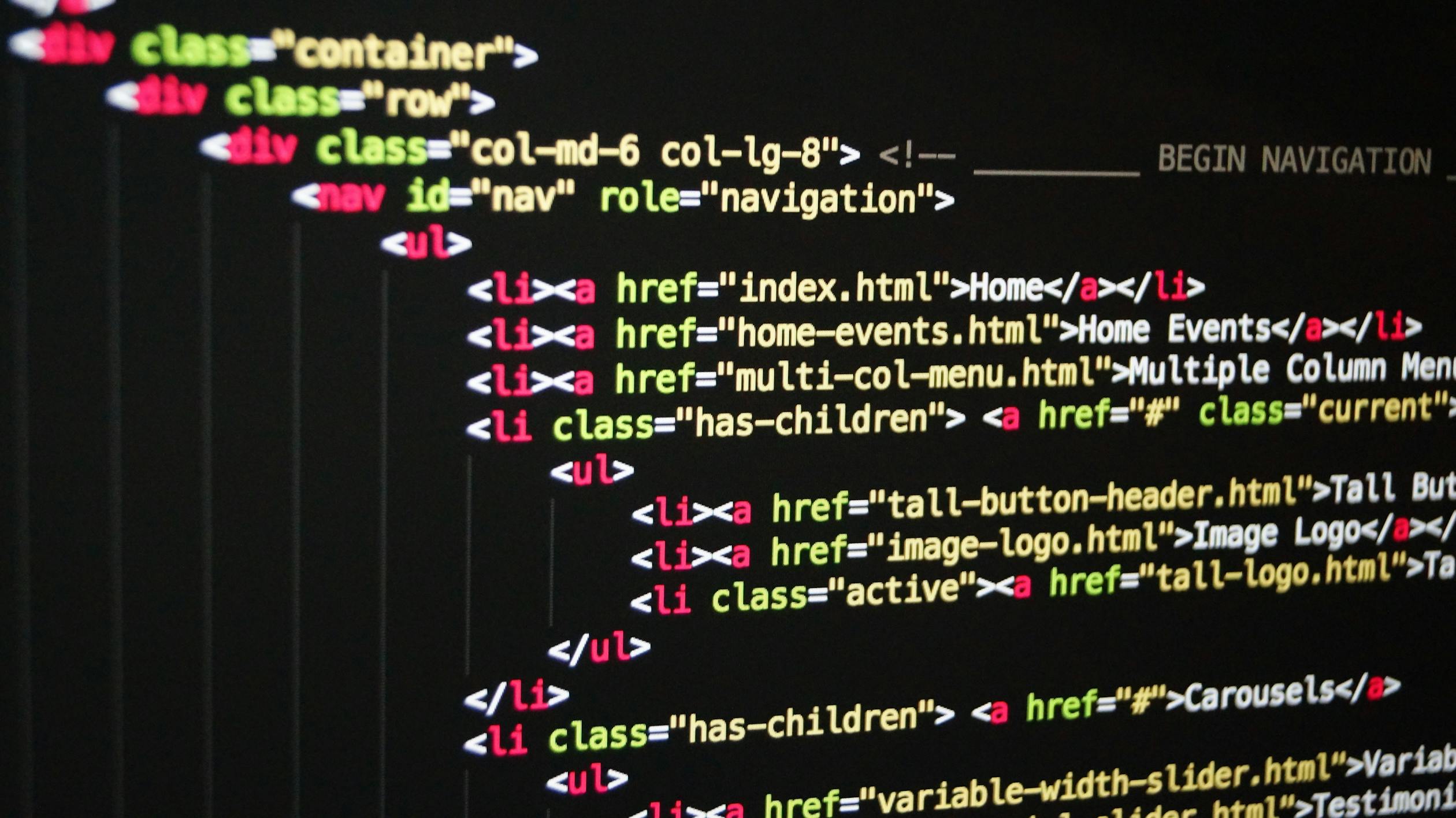
Overview:
The E-commerce Platform Upgrade project was a significant undertaking in which I played a central role as a Fullstack Developer. The goal of this project was to enhance the performance, scalability, and user experience of an existing e-commerce platform. To achieve this, we leveraged JavaScript, ReactJS, Next.js, and MongoDB as the core technologies.
JavaScript/ReactJS:
I utilized ReactJS to build a dynamic and responsive user interface for the platform. React's component-based architecture allowed us to create reusable UI elements, improving development efficiency and ensuring a consistent look and feel throughout the application.
Features I implemented using React included:
- Product catalog with filter and search functionality
- User authentication and authorization for secure transactions
- Shopping cart management with real-time updates
- Integration with third-party payment gateways for seamless checkout
Here's an example of how I structured a React component:
import React, { useState, useEffect } from 'react';
function ProductCatalog() {
const [products, setProducts] = useState([]);
useEffect(() => {
// Fetch product data from the server
// Update the 'products' state with the fetched data
}, []);
return (
<div className="product-catalog">
{/* Render product list */}
{products.map((product) => (
<div key={product.id} className="product">
<h2>{product.name}</h2>
<p>{product.description}</p>
{/* Additional product details */}
</div>
))}
</div>
);
}
export default ProductCatalog;
Next.js:
Next.js was instrumental in optimizing the application's performance. Server-side rendering (SSR) and incremental static site generation (ISG) were employed to reduce page load times and enhance SEO. Additionally, I implemented dynamic routing and code splitting to ensure that the application remained highly responsive even as it scaled.
Here's an example of how I used Next.js for server-side rendering:
// pages/index.js
import React from 'react';
function HomePage({ products }) {
return (
<div>
{/* Render products using server-side data */}
{products.map((product) => (
<div key={product.id}>
<h2>{product.name}</h2>
<p>{product.description}</p>
{/* Additional product details */}
</div>
))}
</div>
);
}
export async function getServerSideProps() {
// Fetch product data from the server
// Return it as 'products' prop
return {
props: {
products: /* Fetched product data */,
},
};
}
export default HomePage;
MongoDB:
MongoDB served as the database technology, providing the flexibility needed to handle vast amounts of product data and user information. I designed the database schema to support efficient querying and indexing, resulting in faster data retrieval and improved overall application performance. MongoDB's support for geospatial data was leveraged to enable location-based features such as store locator and geofencing for promotions.
Here's an example of a MongoDB schema definition:
// MongoDB schema definition using Mongoose (Node.js ODM)
const mongoose = require('mongoose');
const productSchema = new mongoose.Schema({
name: String,
description: String,
// Additional fields
});
const Product = mongoose.model('Product', productSchema);
module.exports = Product;
Challenges and Achievements:
Throughout the project, I faced several challenges, including optimizing database queries for peak performance, ensuring data consistency in real-time, and maintaining a smooth user experience under heavy traffic. By employing caching strategies, load balancing, and asynchronous processing, we were able to overcome these challenges and achieve remarkable results:
- Reduced page load times by 40%
- Improved server response times during peak traffic by 50%
- Increased user engagement and conversion rates by 20%
Conclusion:
The E-commerce Platform Upgrade project was a testament to my skills and experience as a Fullstack Developer. It showcased my proficiency in JavaScript/ReactJS, Next.js, and MongoDB and demonstrated my ability to tackle complex challenges in web application development.
297 views