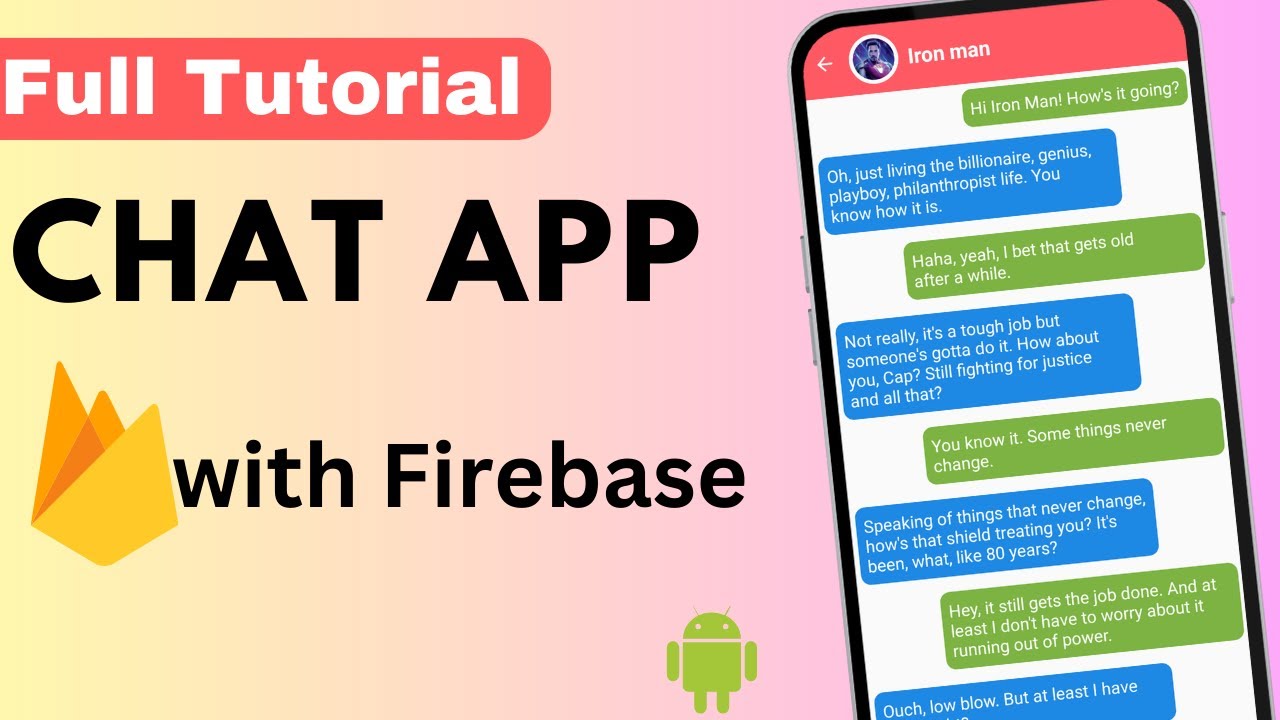
October 08, 2024
How to Create a Real-Time Chat Application with JavaScript and Firebase
Hello, coding novices! Did the idea of making a real-time chat application for your website pop into your mind? Yes? Then you're at the right post. You can create one without any backend complexities using JavaScript and Firebase.
So, no matter whether you're a pro or just stepping into the coding field, you'll find this real-time chat application's building steps very easy. Let's see below:
Setting Up Firebase
With Firebase, you can handle real-time data without any backend complexity. So, to start:
- Create a Firebase Project: Go to the Firebase console on the browser, create a new project there and set up a new web application.
- Enable Authentication: Now, in the Firebase dashboard, enable email or anonymous authentication.
- Configure Firebase: The next step is to get your configuration keys (API key, project ID) and integrate them into your web application.
You'll benefit from the real-time sync feature of Firebase. With this, you can get chat messages immediately on multiple devices.
Creating the HTML Structure
For creating this dynamic chat application, you'll require 3 main HTML elements:
- A chat window for messages.
- An input field so that users can input their messages.
- And a send button to submit your messages.
<div id="chatWindow"></div>
<input type="text" id="messageInput" />
<button id="sendBtn">Send</button>
Integrating Firebase with JavaScript
Ok, so now it's time to connect your chat application with Firebase to make it respond in real-time. And to connect it you've to add Firebase SDK to your HTML file and initialize the chat application.
// Firebase configuration
const firebaseConfig = { /* your Firebase config */ };
firebase.initializeApp(firebaseConfig);
// Reference to Firebase database
const db = firebase.database().ref("messages");
Use JavaScript to listen for new messages and update the chat window in real time:
db.on("child_added", function(snapshot) {
const message = snapshot.val();
displayMessage(message);
});
Sending and Receiving Messages
In this step we'll make it able to send and receive chat messages. When the user type a message and clicks on submit button, then message will be forwarded to Firebase:
document.getElementById("sendBtn").addEventListener("click", function() {
const message = document.getElementById("messageInput").value;
db.push().set({ text: message });
});
Firebase's real-time listener updates the chat window with every database message. This gives any chat application the dynamic feel of a real-time exchange of conversation.
Securing Your Chat Application
Chat applications must be safe and sound. Firebase's security policies restrict message sending and viewing to authorized users. You can also restrict database reads and writes according to user authentication status to make your application secure.
Conclusion
You can build a real-time chat app using JavaScript and Firebase in a few minutes. In this post, I discussed how you can configure Firebase, build its UI, and integrate JavaScript for message processing. Now, you can add features to the app, such as type signs or user messages, to make it better.
469 views