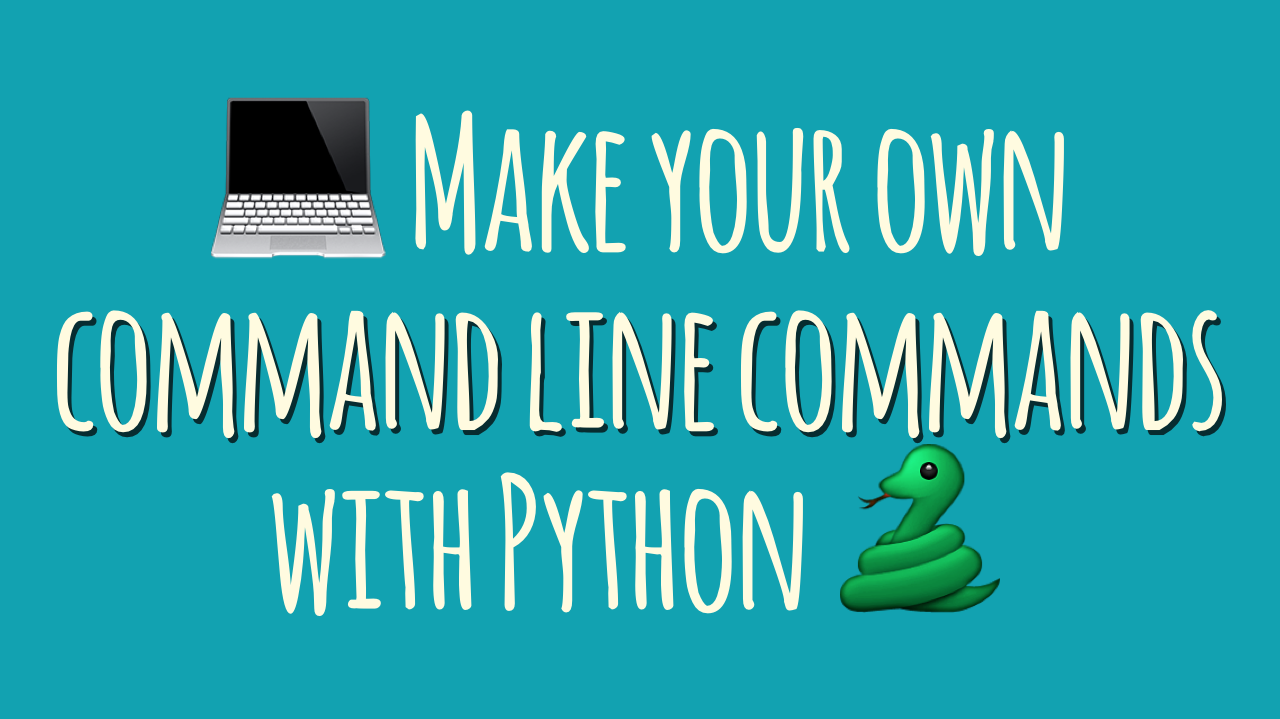
September 30, 2024
Create a Command-Line Tool in Python: Parsing and Handling User Input
Have you ever experienced doing the same task again and again on your computer? How frustrating it is. Right? What if you can solve this problem with just a few simple lines of code? This is where you can take advantage of command-line tools. The plus point for beginners is that you can create your own automated custom tool with Python. And I must say this tool will ease your life to a greater level.
So in this blog post, I'll show you how you can make a simple and base-level Python command-line tool, how you can use the argparse library to parse your input data easily, and I'll also give you tips to handle that parsed use input efficiently.
Let's dive into itâ¦
Why Build Command-Line Tools in Python?
Command-line tools are always a developer's dream. These tools can automate every task, from simple tasks like renaming bulk files to handling complex tasks like executing applications.
I'll recommend Python for creating these types of tools due to its versatility and ease of use. No matter if you want to build a custom tool for your daily life tasks or want to use it to make something complex and advanced, Python excels in making your programming needs easier. There are many programs, like Git, cURL, and even subparts of Python, that are command-line tools. What about making the same tool for yourself?
Basic Structure of a Python Command-Line Tool
In this post, I have a basic structure of Python command-line tools rather than the fancy and complex tools. Let's check out the code of this structure.
def main():
user_input = input("Enter something: ")
print(f"You entered: {user_input}")
if __name__ == "__main__":
main()
This code is for prompting the users to input something and then print it back.
Using argparse to Parse Arguments
In the above code, you saw that the tool prompts the user each time to enter input. But can it be possible that you don't get prompt messages each time? Yes, you can get help from command-line arguments to handle inputs efficiently. And this is where argparse (Python's built-in library) has the expertise.
You can define expected inputs using argparse and can make your command-line tool user-friendly to a greater extent. Here's an example piece of code:
import argparse
def main():
parser = argparse.ArgumentParser(description="A simple command-line tool")
parser.add_argument('name', type=str, help="Your name")
args = parser.parse_args()
print(f"Hello, {args.name}!")
if __name__ == "__main__":
main()
In the above code, argparse library helps to set up the command-line argument "name". After you've run the code such as "python my_tool.py John", you will get "Hello, John!" as output.
Handling User Input Effectively
You've known how to parse the input into your command-line tool; now it's time to know how to handle it. Let's discuss a few things while handling user input:
- Input Validation: Input validation makes sure the user enters input according to the data type you used in the code. For example, if you use text data types but the user enters a number input, then your application will crash immediately. So, you must add some conditional constraints, or you can also add the following try-except block for error handling:
try:
age = int(input("Enter your age: "))
print(f"Your age is: {age}")
except ValueError:
print("Please enter a valid number.")
- Graceful Error Handling: Make sure to provide informative error messages in case any error occurs. For example, if there is an error due to the wrong data type or missing argument, then you can add some tips on how to fix it.
Running and Testing Your Command-Line Tool
Now, the most important step in every developer's life is running and testing. As you've created your own command-line tool, it's time to run and test it. Run your tool by changing different arguments. Like if your script is my_tool.py, try:
python my_tool.py Alice
Same as above, test your tool by passing different names. This helps to check for errors, too, like how well your tool handles errors for wrong data types of blank or missing arguments.
Conclusion
Developing command-line tools in Python helps you automate your tasks, enhance your work speed, and improve your productivity. With a general understanding of the argparse library in Python, you can develop different tools to enter input and handle errors like a pro!
So, why not start from today? Learn it and try to add different arguments to make your command-line tools even more functional
166 views