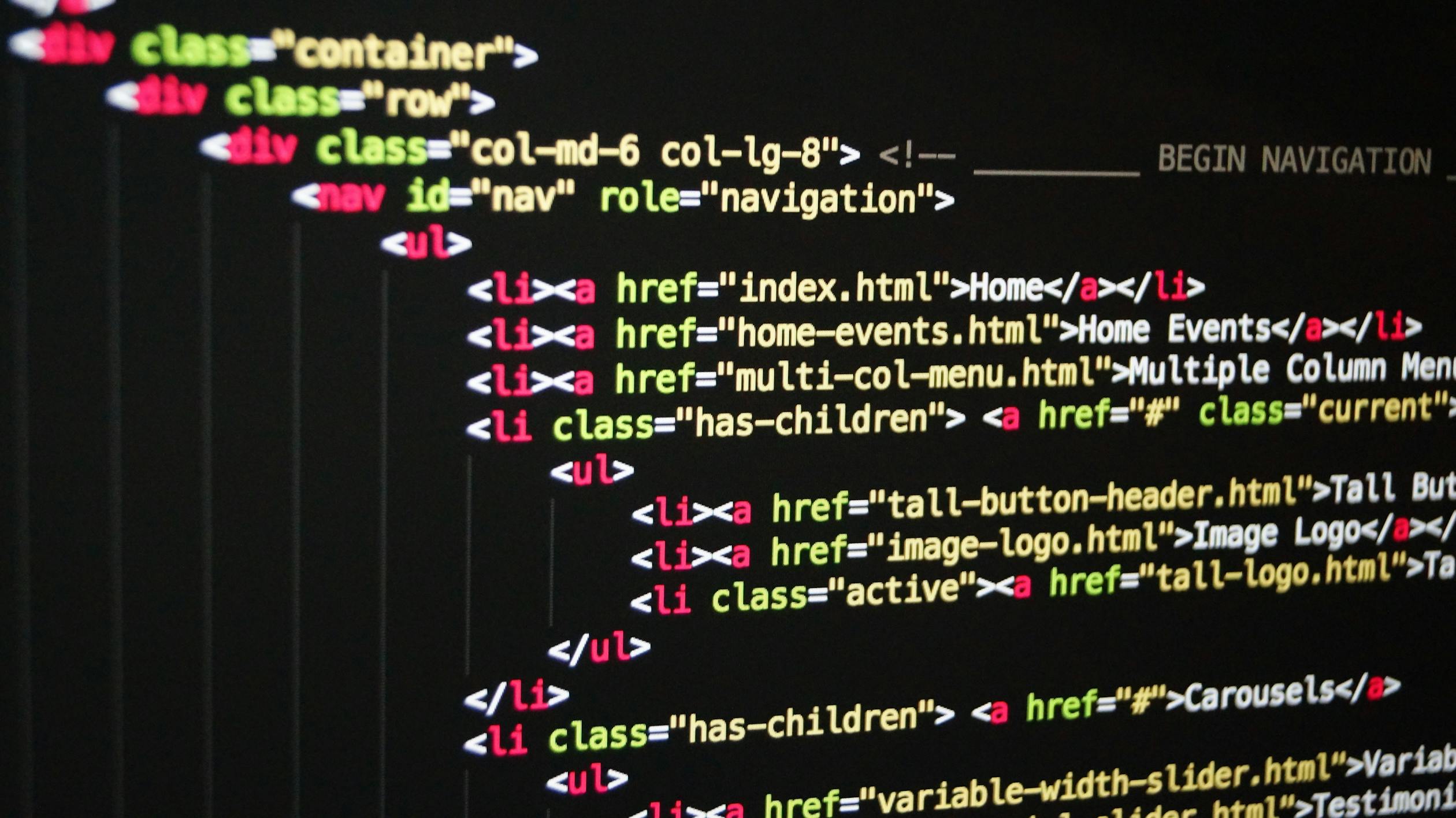
September 18, 2024
Building a Custom Python Shell: Creating Your Own REPL
What happens when you put a command into a coding console and see the results immediately? This is REPL magic. It is a favourite among learning and debugging programmers since it helps you test and execute code in real time.
Even though Python's REPL is already very strong, what if you could make your own shell? Imagine having full control over how it reads, processes, and executes commandsâto your specifications.
This blog post explains REPLs, why developing your own Python shell is fun, and how to make one. I will also provide fascinating methods to improve it! So, ready to code better? Let's start!
What is a REPL?
You can use a Read-Eval-Print Loop (REPL) to work with a programming language sequentially. Read what you write, evaluate (or execute) the code, print the result, and then loop back for additional input. This process is ideal for quick code testing, understanding, and debugging.
Python, Node.js, and Ruby rely on REPLs to let users write code and check the results dynamically. Using these makes programming easier, quicker, and more enjoyable while letting users do real-time code testing.
Why Build a Custom Python Shell?
You can control code execution by building your own Python shell. Customise it to handle unique commands, provide shortcuts, or add capabilities the Python shell lacks. This makes it great for personalised coding.
It also helps you understand Python. Build a REPL to use strong shell functions like exec() and eval(). Also, you can use a unique shell in real-time projects! You can enter it in an app to enable clients to execute code or build a developer debugging tool.
Core Components of a REPL
- Input Handling: First, REPLs read what the user types. It converts text into program-friendly form.
- Evaluation: After receiving input, the REPL runs code using Python's eval() and exec() functions. eval() runs expressions like basic computations, whereas exec() runs code statements like loops or function declarations.
- Output: REPL produces output after evaluating input. As you know the REPL displays error messages so users can understand what went wrong.
- Looping: It loops through reading, evaluating, and printing until the user exits. This is what keeps it going and makes it engaging.
Step-by-Step Guide to Building a Simple Python Shell
Detailed and sequential instructions for building a Python shell:
Step 1: Setting Up a Basic Loop
Start with a basic while loop that receives user input:
while True:
user_input = input(">>> ") # Take user input
This loop is the REPL's basis until we stop it.
Step 2: Adding Input Parsing
Check whether the input is a command (e.g., help or exit). For now, keep things simple:
if user_input == "exit":
break # Exit the loop
This lets users type "exit" to quit shells.
Step 3: Executing the Input
Python's eval() and exec() could evaluate expressions and statements, respectively:
try:
result = eval(user_input) # Handle expressions print(result)
except:
exec(user_input) # Handle statements like loops or functions
Step 4: Handling Errors Gracefully
Try-except error handling prevents REPL crashes from poor input:
try:
exec(user_input)
except Exception as e:
print(f"Error: {e}")
Step 5: Exit Mechanism
Finally, make REPL exit easy:
if user_input.lower() == "exit":
break
Improvements for a More Advanced Python Shell
To make a basic Python shell more interesting and powerful, you may add the following features:
- Custom Commands: Create custom commands, such as help to list available commands or exit to quit. By this customisation, your shell becomes more easier to use.
- Multiline Input Handling: Allow users to input numerous lines (e.g., for loops or function definitions) before running complicated code.
- History Management: Use the readline module to save earlier commands so users may use the up arrow to repeat them, like the Python shell.
- Syntax Highlighting: Adding syntax highlighting to your shell will make it look better and be easier to understand. Python tools, such as pygments, can make it easier to code by highlighting keywords, variables, and strings.
Applications and Future Expansions
You can use your Python shell in numerous ways:
- Project Embedding: You can integrate your REPL in bigger Python programs to let people interact with your code in real time.
- Adding Debugging Features: Integrating with Python's built-in debugger (pdb) lets developers use powerful shell debugging to find and fix errors.
- Creating an IDE-Like Environment: You can use your shell to make a portable IDE that has syntax highlighting and a command log.
Conclusion
A great way to learn Python is to make your own Python shell and tool. Use a custom shell to learn how to code and change how things work. After you understand the basics, try the code! You can also add your own changes or additions. Check out and change your Python shell to make it more useful.
479 views