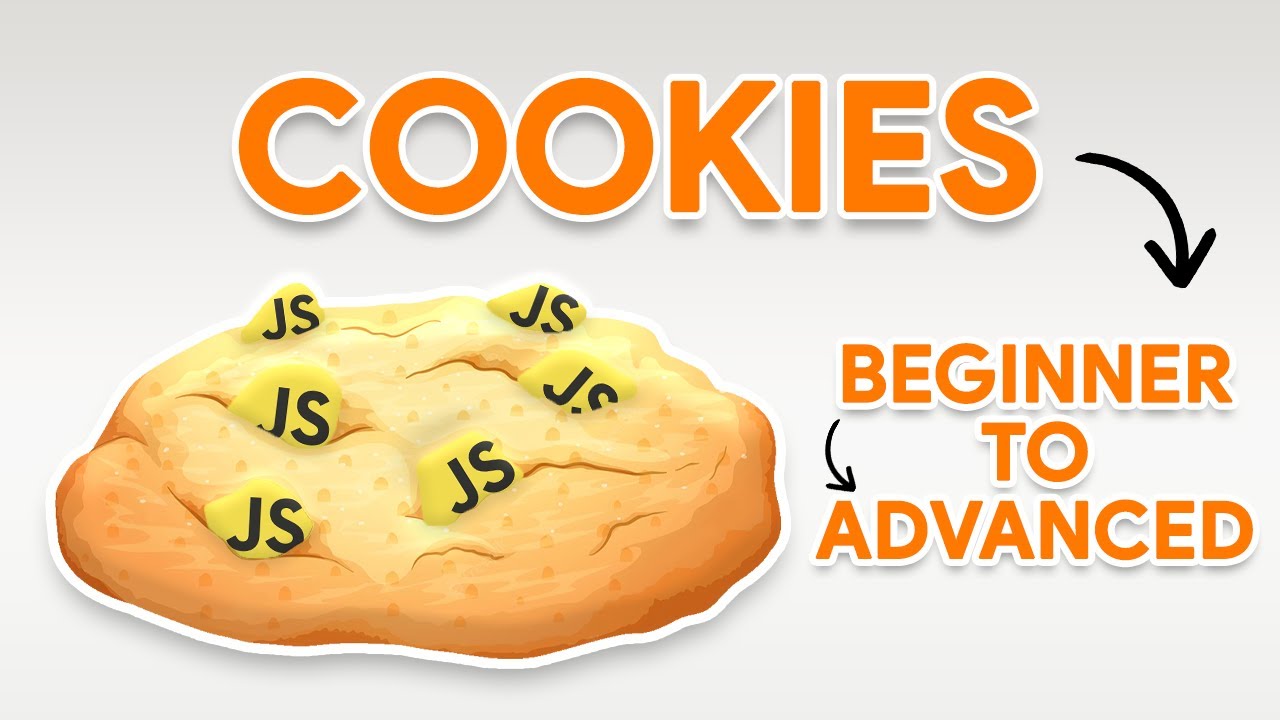
Cookies are small pieces of data stored on the client side, often used to manage state and user preferences in web applications. In this blog post, we'll explore how to set and delete cookies using Remix, a modern web framework for building better user experiences.
Prerequisites
Before we dive into the code, make sure you have the following:
- Basic understanding of JavaScript and web development.
- Familiarity with Remix framework. If not, check out the official documentation.
Setting Up a Remix Project
First, create a new Remix project. If you haven't already installed the Remix CLI, do so with:
npm install -g create-remix
Then create your project:
npx create-remix@latest
Follow the prompts to set up your project. Once it's set up, navigate to the project directory:
cd your-remix-project
Setting a Cookie
Setting a cookie in Remix is straightforward. You can do it within a loader or action function using the cookie
helper.
Step-by-Step Guide to Setting a Cookie
- Create a Cookie:
First, define your cookie. This is usually done in a separate module for better organization. Create a file called cookies.js
in your utils
folder.
// utils/cookies.js
import { createCookie } from "@remix-run/node";
export const userPreferencesCookie = createCookie("user-preferences", {
maxAge: 60 * 60 * 24 * 7 // 1 week
});
- Set the Cookie in a Loader or Action:
Next, use this cookie in your loader or action. For example, in your routes/index.jsx
file:
// routes/index.jsx
import { json } from "@remix-run/node";
import { useLoaderData } from "@remix-run/react";
import { userPreferencesCookie } from "../utils/cookies";
export const loader = async ({ request }) => {
const cookieHeader = request.headers.get("Cookie");
const userPreferences = (await userPreferencesCookie.parse(cookieHeader)) || {};
return json({ userPreferences }, {
headers: {
"Set-Cookie": await userPreferencesCookie.serialize({ theme: "dark" })
}
});
};
export default function Index() {
const { userPreferences } = useLoaderData();
return (
<div>
<h1>Welcome to Remix!</h1>
<p>Your preferred theme is: {userPreferences.theme}</p>
</div>
);
}
This sets a cookie named user-preferences
with a value { theme: "dark" }
when the page loads.
Deleting a Cookie
Deleting a cookie involves setting its expiration date to a past date. Remix provides a convenient way to do this.
Step-by-Step Guide to Deleting a Cookie
- Create a Cookie (if not already created):
Ensure you have the cookie defined as shown earlier.
- Delete the Cookie in a Loader or Action:
To delete the cookie, set its expiration date to the past. For example, in your routes/index.jsx
file:
// routes/index.jsx
import { json, redirect } from "@remix-run/node";
import { userPreferencesCookie } from "../utils/cookies";
export const action = async ({ request }) => {
const formData = await request.formData();
if (formData.get("action") === "delete") {
return redirect("/", {
headers: {
"Set-Cookie": await userPreferencesCookie.serialize("", { expires: new Date(0) })
}
});
}
return null;
};
export default function Index() {
return (
<div>
<h1>Welcome to Remix!</h1>
<form method="post">
<button type="submit" name="action" value="delete">Delete Cookie</button>
</form>
</div>
);
}
Here, when the form is submitted, it triggers the action function which deletes the cookie by setting its expiration date to January 1, 1970.
Conclusion
Managing cookies in Remix is simple and efficient. By following the steps above, you can easily set and delete cookies in your Remix application, enhancing user experience and state management.
Happy coding with Remix! If you have any questions or run into issues, feel free to reach out in the comments below.
831 views