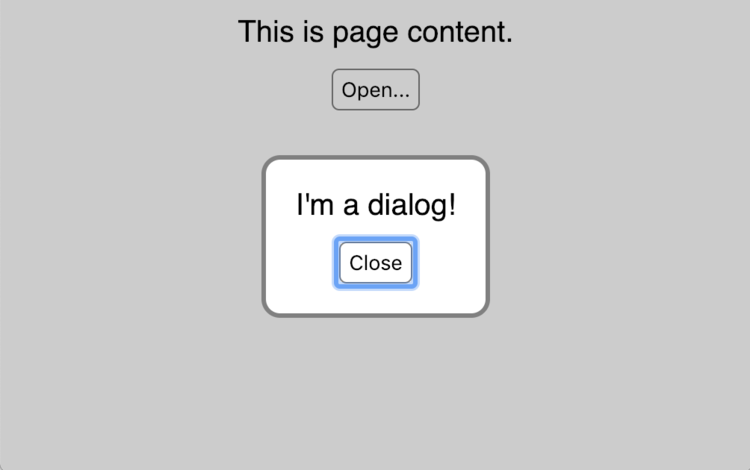
August 28, 2024
Adding Dialogs to Your React Application Using the <dialog> HTML Tag
Dialogs are essential components in web applications, used to alert users, gather input, or display important information. In React applications, implementing a dialog can be efficiently handled using the native <dialog> HTML tag, which provides a built-in way to create modal dialogs. Here's a comprehensive guide on the best way to add dialogs to your React application using the <dialog> tag.
Why Use the <dialog>
Tag?
The <dialog>
HTML tag offers several advantages:
- Native Support: It comes with built-in functionality, reducing the need for additional JavaScript to manage dialog behavior.
- Accessibility: It supports keyboard accessibility features out of the box.
- Simplicity: It simplifies the creation of modals, minimizing the amount of code you need to write.
Step-by-Step Guide
1. Setting Up Your React Project
First, ensure you have a React project set up. If not, you can create one using Create React App:
npx create-react-app my-dialog-app
cd my-dialog-app
npm start
2. Creating the Dialog Component
Create a new component called DialogComponent.js
in the src
directory:
import React, { useRef } from 'react';
const DialogComponent = () => {
const dialogRef = useRef(null);
const openDialog = () => {
dialogRef.current.showModal();
};
const closeDialog = () => {
dialogRef.current.close();
};
return (
<div>
<button onClick={openDialog}>Open Dialog</button>
<dialog ref={dialogRef}>
<h2>Dialog Title</h2>
<p>This is a dialog using the <dialog> HTML tag in React.</p>
<button onClick={closeDialog}>Close</button>
</dialog>
</div>
);
};
export default DialogComponent;
3. Using the Dialog Component
Now, you need to include the DialogComponent
in your main application component, typically App.js
:
import React from 'react';
import DialogComponent from './DialogComponent';
function App() {
return (
<div className="App">
<h1>Welcome to the Dialog Demo</h1>
<DialogComponent />
</div>
);
}
export default App;
4. Styling the Dialog
To ensure your dialog looks good, you can add some basic CSS. Create a DialogComponent.css
file:
dialog {
border: none;
border-radius: 8px;
padding: 20px;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.1);
width: 300px;
}
dialog::backdrop {
background: rgba(0, 0, 0, 0.5);
}
And import this CSS file in your DialogComponent.js
:
import './DialogComponent.css';
5. Enhancing the Dialog
You can further enhance the dialog by adding animations, more complex content, or integrating form elements. Hereâs an example with a form inside the dialog:
import React, { useRef } from 'react';
import './DialogComponent.css';
const DialogComponent = () => {
const dialogRef = useRef(null);
const openDialog = () => {
dialogRef.current.showModal();
};
const closeDialog = () => {
dialogRef.current.close();
};
const handleSubmit = (event) => {
event.preventDefault();
// Handle form submission logic here
closeDialog();
};
return (
<div>
<button onClick={openDialog}>Open Dialog</button>
<dialog ref={dialogRef}>
<form onSubmit={handleSubmit}>
<h2>Submit Your Info</h2>
<label>
Name:
<input type="text" name="name" required />
</label>
<label>
Email:
<input type="email" name="email" required />
</label>
<button type="submit">Submit</button>
<button type="button" onClick={closeDialog}>Cancel</button>
</form>
</dialog>
</div>
);
};
export default DialogComponent;
Conclusion
Using the <dialog>
HTML tag in a React application is a straightforward and effective way to create modal dialogs. It leverages native browser capabilities, ensuring your dialogs are both accessible and easy to implement. By following the steps outlined in this guide, you can quickly add functional and stylish dialogs to your React projects.
462 views