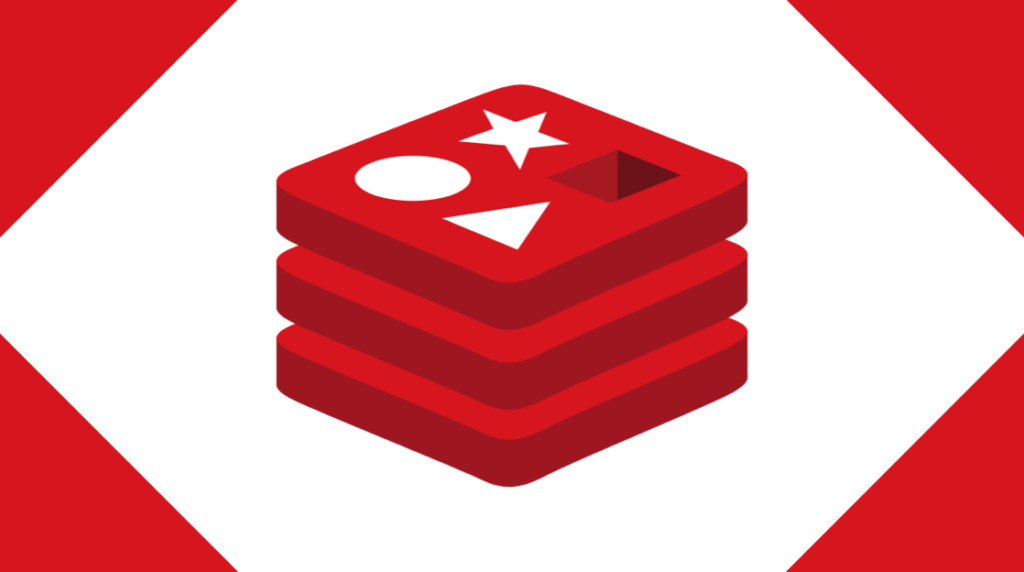
Redis is an in-memory data structure store often used as a database, cache, and message broker. Its speed and efficiency make it a popular choice for developers looking to enhance the performance of their applications. In this blog, weâll explore how to use Redis in Golang, covering everything from installation to advanced usage.
Why Use Redis?
Redis offers several benefits that make it an attractive option for developers:
- Speed: Redis operates in memory, providing extremely fast read and write operations.
- Data Structures: Redis supports various data structures such as strings, hashes, lists, sets, and sorted sets.
- Persistence: While Redis is primarily an in-memory database, it supports persistence by saving data to disk.
- Scalability: Redis can handle a large number of operations per second and can be scaled horizontally with clustering.
Setting Up Redis
Before diving into using Redis with Golang, ensure you have Redis installed on your system. You can download and install Redis from the official website. After installation, start the Redis server:
redis-server
You can interact with Redis through the command line interface (CLI) to verify that itâs running:
redis-cli
Using Redis in Golang
To use Redis in Golang, we'll leverage the go-redis
library, a Redis client for Go. Follow these steps to get started:
Step 1: Installing the go-redis Package
First, you need to install the go-redis
package. You can do this using go get
:
go get github.com/go-redis/redis/v8
Step 2: Connecting to Redis
Create a new Go file and import the necessary packages. Then, establish a connection to the Redis server:
package main
import (
"context"
"fmt"
"github.com/go-redis/redis/v8"
)
var ctx = context.Background()
func main() {
rdb := redis.NewClient(&redis.Options{
Addr: "localhost:6379",
Password: "", // no password set
DB: 0, // use default DB
})
_, err := rdb.Ping(ctx).Result()
if err != nil {
fmt.Println("Could not connect to Redis:", err)
} else {
fmt.Println("Connected to Redis")
}
}
Step 3: Basic Redis Operations
With the connection established, you can perform various Redis operations such as setting and getting values.
Set a Value
func setValue(rdb *redis.Client, key string, value interface{}) {
err := rdb.Set(ctx, key, value, 0).Err()
if err != nil {
fmt.Println("Error setting value:", err)
}
}
Get a Value
func getValue(rdb *redis.Client, key string) {
val, err := rdb.Get(ctx, key).Result()
if err == redis.Nil {
fmt.Println("Key does not exist")
} else if err != nil {
fmt.Println("Error getting value:", err)
} else {
fmt.Println("Value:", val)
}
}
Step 4: Working with Other Data Structures
Redis supports a variety of data structures. Hereâs how you can use some of them in Golang:
Lists
func pushToList(rdb *redis.Client, listName string, values ...interface{}) {
err := rdb.RPush(ctx, listName, values...).Err()
if err != nil {
fmt.Println("Error pushing to list:", err)
}
}
func getList(rdb *redis.Client, listName string) {
vals, err := rdb.LRange(ctx, listName, 0, -1).Result()
if err != nil {
fmt.Println("Error getting list:", err)
} else {
fmt.Println("List values:", vals)
}
}
Hashes
func setHashValue(rdb *redis.Client, hashName, key string, value interface{}) {
err := rdb.HSet(ctx, hashName, key, value).Err()
if err != nil {
fmt.Println("Error setting hash value:", err)
}
}
func getHashValue(rdb *redis.Client, hashName, key string) {
val, err := rdb.HGet(ctx, hashName, key).Result()
if err == redis.Nil {
fmt.Println("Key does not exist")
} else if err != nil {
fmt.Println("Error getting hash value:", err)
} else {
fmt.Println("Hash value:", val)
}
}
Step 5: Handling Errors and Best Practices
When working with Redis and go-redis
, handle errors gracefully. Always check for errors returned by Redis operations to ensure your application can respond appropriately.
Conclusion
Using Redis in Golang can significantly enhance the performance and scalability of your applications. With the go-redis
library, interacting with Redis is straightforward, allowing you to take advantage of its powerful features with minimal effort. Whether youâre caching data, managing sessions, or handling real-time data processing, Redis provides the tools you need to build efficient and robust applications.
602 views