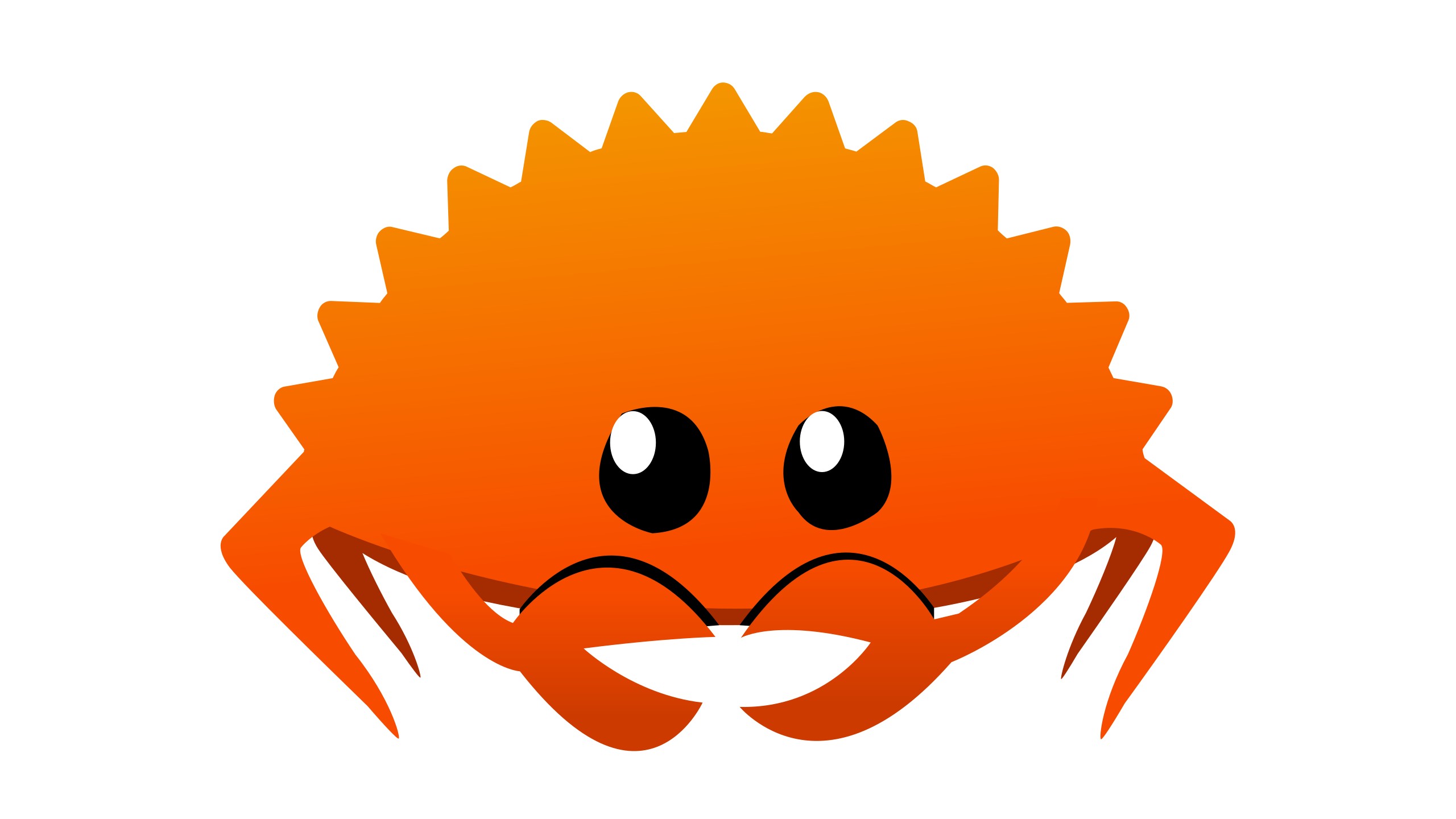
Rust is rapidly gaining popularity for its emphasis on safety and performance, and its asynchronous programming capabilities are a significant part of its appeal. Asynchronous programming in Rust allows developers to write concurrent code that is both efficient and easy to maintain. This blog will delve into the essentials of asynchronous programming in Rust, explaining the concepts, benefits, and practical applications.
What is Asynchronous Programming?
Asynchronous programming is a paradigm that allows a program to handle multiple operations concurrently, without waiting for each operation to complete before starting the next one. This is especially useful for I/O-bound tasks, such as network requests or file operations, where waiting for a response can significantly delay the execution of other tasks.
Why Rust for Asynchronous Programming?
Rust provides several features that make it a powerful language for asynchronous programming:
- Memory Safety: Rust's ownership system ensures that your code is free from data races and other concurrency bugs.
- Performance: Rust's zero-cost abstractions mean that asynchronous code runs with minimal overhead.
- Ecosystem: The Rust ecosystem, particularly the async-std and tokio libraries, provides robust support for asynchronous programming.
Key Concepts
Futures
In Rust, the core abstraction for asynchronous programming is the Future trait. A Future represents a value that may not be available yet. When the value is ready, the future is resolved.
use std::future::Future;
fn example() -> impl Future<Output = u32> {
async {
42
}
}
async/await
The async and await keywords simplify working with futures. An async function returns a future, and await can be used to pause execution until the future is ready.
async fn fetch_data() -> u32 {
42
}
async fn example() {
let result = fetch_data().await;
println!("Result: {}", result);
}
Executors
Executors are responsible for running asynchronous tasks. The two most popular executors in Rust are tokio and async-std. They provide the runtime environment needed to poll futures and drive them to completion.
use tokio::runtime::Runtime;
fn main() {
let rt = Runtime::new().unwrap();
rt.block_on(async {
example().await;
});
}
Building an Asynchronous Application
Letâs walk through building a simple asynchronous web server using tokio.
- Setup
First, add tokio to your Cargo.toml:
[dependencies]
tokio = { version = "1", features = ["full"] }
- Creating the Server
Create a new Rust file (e.g., main.rs) and set up the basic server structure:
use tokio::net::TcpListener;
use tokio::prelude::*;
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let listener = TcpListener::bind("127.0.0.1:8080").await?;
loop {
let (mut socket, _) = listener.accept().await?;
tokio::spawn(async move {
let mut buf = [0; 1024];
loop {
let n = match socket.read(&mut buf).await {
Ok(n) if n == 0 => return,
Ok(n) => n,
Err(e) => {
eprintln!("failed to read from socket; err = {:?}", e);
return;
}
};
if socket.write_all(&buf[0..n]).await.is_err() {
eprintln!("failed to write to socket");
return;
}
}
});
}
}
Explanation
- TcpListener: Listens for incoming TCP connections.
- tokio::spawn: Spawns a new asynchronous task.
- socket.read: Reads data from the socket.
- socket.write_all: Writes data to the socket.
This server listens on 127.0.0.1:8080 and echoes any received data back to the client.
Advanced Topics
Async Streams
Rust also supports asynchronous streams, which are similar to futures but yield multiple values over time.
use futures::stream::StreamExt;
async fn example() {
let mut stream = tokio::stream::iter(vec![1, 2, 3]);
while let Some(value) = stream.next().await {
println!("Got value: {}", value);
}
}
Error Handling
Handling errors in asynchronous Rust code follows the same principles as synchronous code, often using the Result type.
async fn fetch_data() -> Result<u32, &'static str> {
Ok(42)
}
async fn example() {
match fetch_data().await {
Ok(value) => println!("Got value: {}", value),
Err(e) => eprintln!("Error: {}", e),
}
}
Conclusion
Asynchronous programming in Rust is a powerful tool for building efficient and scalable applications. With the async and await syntax, combined with robust libraries like tokio, Rust makes it easier than ever to write high-performance concurrent code. Whether you are building a simple web server or a complex distributed system, Rustâs asynchronous programming model provides the tools you need to succeed.
553 views