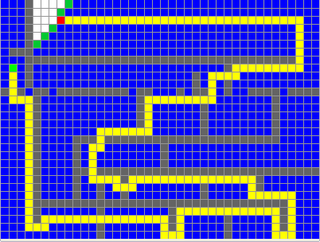
August 02, 2024
Navigating the Maze: Building a Route Finding Console Application with Dijkstra's Algorithm in C#
Introduction:
In a world where efficiency is paramount, route finding algorithms play a crucial role in various applications, from GPS navigation systems to logistics planning. Among these algorithms, Dijkstra's algorithm stands out for its simplicity and effectiveness in finding the shortest path between nodes in a graph. In this tutorial, we'll embark on a journey to implement a route finding console application using C# and Dijkstra's algorithm.
Prerequisites:
Before diving into the implementation, it's essential to have a basic understanding of C# programming language, object-oriented programming concepts, and familiarity with graph theory and Dijkstra's algorithm.
Setting Up the Project:
Let's start by creating a new C# console application project in your preferred Integrated Development Environment (IDE) such as Visual Studio or Visual Studio Code. Once the project is set up, we can proceed with the implementation.
Implementing Dijkstra's Algorithm:
Define the Graph Structure: We'll represent our graph using adjacency lists, where each node is associated with a list of adjacent nodes and their corresponding weights.
public class Graph
{
private Dictionary<int, Dictionary<int, int>> adjacencyList;
public Graph()
{
adjacencyList = new Dictionary<int, Dictionary<int, int>>();
}
public void AddEdge(int source, int destination, int weight)
{
if (!adjacencyList.ContainsKey(source))
adjacencyList[source] = new Dictionary<int, int>();
adjacencyList[source][destination] = weight;
}
public Dictionary<int, int> GetNeighbors(int node)
{
return adjacencyList.ContainsKey(node) ? adjacencyList[node] : new Dictionary<int, int>();
}
}
Implement Dijkstra's Algorithm: Dijkstra's algorithm finds the shortest path from a given start node to all other nodes in the graph.
public class Dijkstra
{
public Dictionary<int, int> ShortestPath(Graph graph, int start)
{
var distances = new Dictionary<int, int>();
var visited = new HashSet<int>();
var priorityQueue = new SortedSet<(int distance, int node)>();
distances[start] = 0;
priorityQueue.Add((0, start));
while (priorityQueue.Count > 0)
{
var (distance, node) = priorityQueue.Min;
priorityQueue.Remove((distance, node));
if (visited.Contains(node)) continue;
visited.Add(node);
foreach (var (neighbor, weight) in graph.GetNeighbors(node))
{
if (!distances.ContainsKey(neighbor) || distances[neighbor] > distance + weight)
{
distances[neighbor] = distance + weight;
priorityQueue.Add((distances[neighbor], neighbor));
}
}
}
return distances;
}
}
Building the Console Application: Now, let's put everything together in our console application to find the shortest path between two nodes in a graph.
class Program
{
static void Main(string[] args)
{
Graph graph = new Graph();
// Add edges and weights to the graph
graph.AddEdge(0, 1, 4);
graph.AddEdge(0, 2, 1);
graph.AddEdge(1, 3, 1);
graph.AddEdge(2, 1, 2);
graph.AddEdge(2, 3, 5);
graph.AddEdge(3, 4, 3);
Dijkstra dijkstra = new Dijkstra();
int startNode = 0;
Dictionary<int, int> distances = dijkstra.ShortestPath(graph, startNode);
foreach (var node in distances)
{
Console.WriteLine($"Shortest distance from node {startNode} to node {node.Key} is {node.Value}");
}
}
}
Conclusion:
In this tutorial, we've explored the implementation of a route finding console application using C# and Dijkstra's algorithm. By leveraging graph theory and algorithmic principles, we can efficiently navigate through complex networks and find the shortest paths between nodes. This application serves as a foundational example that can be extended and integrated into various real-world scenarios where route finding is essential.
598 views