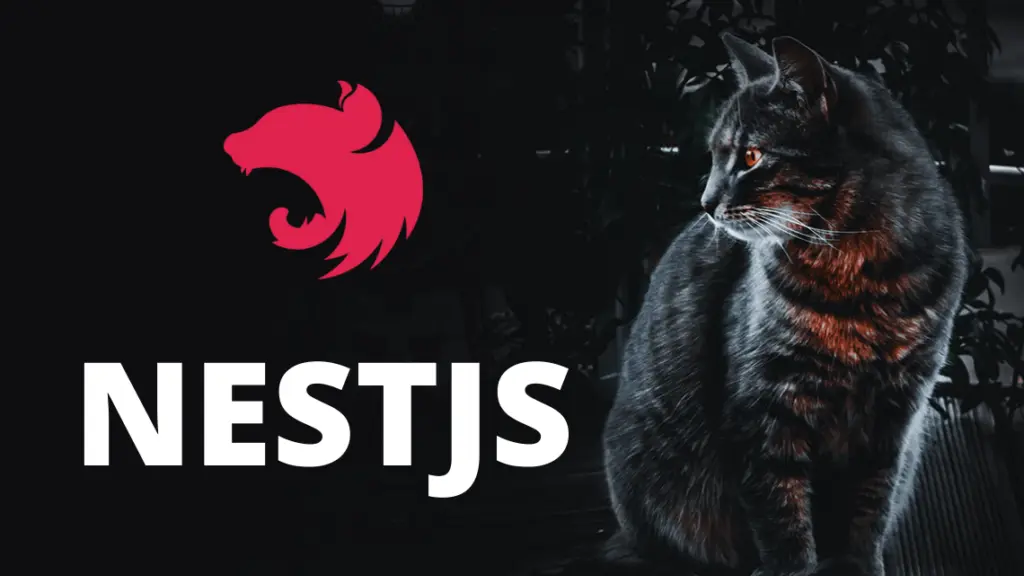
May 02, 2024
Decorators in Nest.js: A Powerful Tool for Building Expressive Applications
In the realm of web development, building robust and maintainable applications is a constant pursuit. Developers are always on the lookout for tools and patterns that can streamline development, enhance code readability, and promote best practices. One such tool that has gained significant traction in recent years is decorators.
Decorators are a feature of TypeScript (and JavaScript) that allow you to annotate and modify classes and their members at design time. They provide a way to add metadata to your code, enabling powerful runtime behaviors and facilitating clean and expressive syntax. In the context of server-side JavaScript frameworks like Nest.js, decorators play a pivotal role in defining routes, middleware, and other aspects of your application.
Understanding Decorators in Nest.js
Nest.js, a progressive Node.js framework for building efficient and scalable server-side applications, leverages decorators extensively to define various components of your application. These decorators serve as markers that denote the purpose or behavior of a particular class or method.
For example, consider the @Controller decorator. When applied to a class, it identifies that class as a controller, responsible for handling incoming HTTP requests. Similarly, the @Get, @Post, @Put, and @Delete decorators specify the HTTP request method associated with a particular route.
@Controller('cats')
export class CatsController {
constructor(private readonly catsService: CatsService) {}
@Get()
async findAll(): Promise<Cat[]> {
return this.catsService.findAll();
}
@Post()
async create(@Body() createCatDto: CreateCatDto) {
this.catsService.create(createCatDto);
}
}
In this example, CatsController is marked with @Controller('cats'), indicating that it handles requests for routes under the /cats path. The findAll method is decorated with @Get(), signifying that it responds to HTTP GET requests to the /cats endpoint, while the create method is decorated with @Post(), indicating that it handles POST requests.
Custom Decorators
While Nest.js provides a rich set of built-in decorators for common use cases, you can also create custom decorators to encapsulate reusable functionality within your application. Custom decorators enable you to abstract away complex logic and promote code reusability.
For instance, you might create a @AuthGuard decorator to enforce authentication and authorization checks for certain routes:
export const AuthGuard = () => SetMetadata('roles', ['user']);
@Injectable()
export class RolesGuard implements CanActivate {
canActivate(context: ExecutionContext): boolean {
const roles = this.reflector.get<string[]>('roles', context.getHandler());
if (!roles) {
return true;
}
// Logic to check if the user has the required role
}
}
Here, AuthGuard is a custom decorator that sets metadata indicating the required roles for accessing a particular route. You can then apply this decorator to controller methods or entire controllers to enforce role-based access control.
Advantages of Using Decorators in Nest.js Applications
Clean and Readable Code: Decorators enable you to express your application's structure and behavior in a clear and concise manner, improving code readability and maintainability.
Promotes Separation of Concerns: By encapsulating functionality within decorators, you can separate concerns and adhere to the principles of modularity and single responsibility.
Facilitates Reusability: Custom decorators allow you to encapsulate common patterns and behaviors, promoting code reuse across different parts of your application.
Enhances Developer Experience: Decorators provide a declarative way to define application components and behaviors, making it easier for developers to reason about the codebase and collaborate effectively.
In conclusion, decorators are a powerful feature of TypeScript and JavaScript that play a crucial role in defining and structuring Nest.js applications. By leveraging decorators effectively, you can build expressive, maintainable, and scalable server-side applications that adhere to best practices and promote code reusability. Whether you're defining routes, middleware, or custom functionality, decorators are an indispensable tool in the Nest.js developer's toolkit.
434 views